PyCharm Python Code Completion Guide: Automate Code Writing, Save Time
发布时间: 2024-09-14 21:52:08 阅读量: 24 订阅数: 28 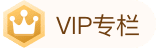
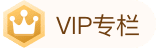
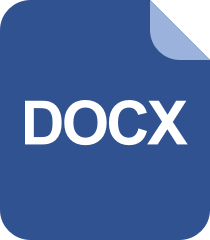
Python与PyCharm的入门到精通:安装配置全流程指南
# Introduction to PyCharm Python Code Completion
PyCharm, as a professional IDE for Python, offers robust code completion features that can enhance developers' coding efficiency and accuracy. Code completion predicts the code developers might input and suggests completions, reducing the time and effort spent on manual coding. It can automatically complete code snippets, keywords, variables, functions, and even generate code structures and refactor code.
PyCharm's code completion is based on advanced algorithms and language models that intelligently analyze the context of the code and provide the most relevant suggestions based on the developer's input. It also supports custom code completion templates and external libraries, allowing developers to customize code completion behavior according to their needs.
# The Theoretical Foundation of Python Code Completion
### 2.1 Code Completion Algorithms and Technologies
The code completion algorithm aims to predict possible completion options based on the input code snippet. PyCharm utilizes a variety of algorithms, including:
- **Pattern-based completion:** Matches existing code snippets based on the pattern of the input code, providing completion suggestions.
- **Context-aware completion:** Takes into account the semantic and syntactic information of the code context to provide more accurate completion options.
- **Machine learning-based completion:** Utilizes machine learning models to analyze code patterns and completion histories, offering personalized completion suggestions.
### 2.2 Language Models and Syntax Analysis for Code Completion
PyCharm's code completion relies on a thorough understanding of the Python language. It employs the following language models and syntax analysis techniques:
- **Abstract Syntax Tree (AST):** Represents Python code as a tree structure, facilitating the analysis of code structure and semantics.
- **Lexical analysis:** Breaks code down into smaller syntax units (tokens), such as keywords, identifiers, and operators.
- **Syntax parsing:** Analyzes code structures according to syntax rules, identifying statements, expressions, and blocks.
By combining these techniques, PyCharm can understand the syntax and semantics of the code and provide accurate and relevant completion options.
#### Code Block Example:
```python
# Code snippet
my_list = [1, 2, 3]
```
#### Logical Analysis:
- **Lexical analysis:** Breaks the code into tokens: `my_list` (identifier), `=` (assignment operator), `[1, 2, 3]` (list literal).
- **Syntax parsing:** Identifies the code structure: Variable `my_list` is assigned a list.
- **Completion suggestion:** Based on the context, PyCharm provides completion options related to list operations, such as `.append()`, `.sort()`, `.reverse()`.
#### Table: Comparison of Python Code Completion Algorithms
| Algorithm | Advantages | Disadvantages |
|---|---|---|
| Pattern-based completion | Fast, simple | Completion options may be inaccurate |
| Context-aware completion | More accurate, more relevant | Higher computational cost |
| Machine learning-based completion | Personalized, efficient | Requires training data |
#### Mermaid Flowchart: Python Code Completion Process
```mermaid
sequenceDiagram
participant User
participant PyCharm
User->PyCharm: Input code fragment
PyCharm->PyCharm: Analyze code using language models and syntax analysis
PyCharm->PyCharm: Apply code completion algorithms
PyCharm->User: Provide completion suggestions
```
# 3.1 Automatically Completing Code Snippets and Keywords
PyCharm's code completion is not limited to automatically completing individual identifiers; it can also automatically complete code snippets and keywords. This is very useful for quickly inputting common code patterns and structures.
#### Automatically Completing Code Snippets
PyCharm offers a rich library of code snippets covering various common programming tasks, such as loops, conditional statements, and function calls. To use a code snippet, just type the first few characters of the snippet in the editor and then press the `Tab` key
0
0
相关推荐
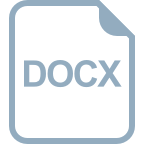
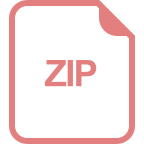
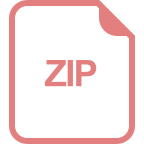
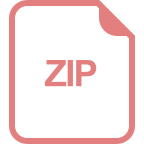
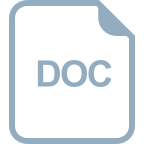
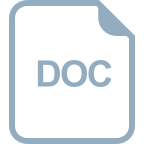
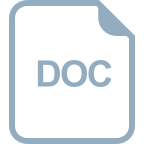