PyCharm Python Code Completion Guide: Smart Assistance to Boost Development Speed
发布时间: 2024-09-14 21:50:55 阅读量: 20 订阅数: 24 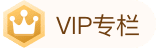
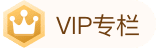
# 1. An Introduction to PyCharm Python Code Hints
PyCharm is a powerful Python integrated development environment (IDE) that offers a wide range of code hinting features designed to enhance development efficiency and ensure code quality. These code hints cover various aspects, including code completion, code inspection, code refactoring, and documentation hints, providing comprehensive support for developers.
The code hinting features dynamically offer suggestions and assistance by analyzing the code context and project structure. They can help developers write code quickly and accurately, reduce errors, and maintain consistency in coding style. In subsequent chapters, we will delve into the various types, applications, and customization options of PyCharm Python code hints to fully leverage their advantages.
# 2. Types of PyCharm Python Code Hints
PyCharm offers a variety of code hint types to assist developers in improving the efficiency and quality of their code writing. These hints include:
### 2.1 Code Completion
The code completion feature can automatically complete code, including:
#### 2.1.1 Auto-Completion
The auto-completion feature can automatically complete possible options based on the code that has been input. For example, when you enter a class name, PyCharm will automatically complete all methods and attributes of that class.
```python
class MyClass:
def __init__(self):
self.name = "John"
my_class = MyClass()
my_class.n # Auto-completed as name
```
#### 2.1.2 Code Snippet Completion
The code snippet completion feature can automatically complete predefined code snippets. These snippets can be common code patterns, function calls, or entire class definitions.
```python
import pandas as pd
df = pd.read_csv("data.csv")
df.head() # Auto-completed as df.head()
```
### 2.2 Code Inspection
The code inspection feature can help identify potential issues in the code, including:
#### 2.2.1 Syntax Checking
The syntax checking feature can detect syntax errors in the code, such as unclosed parentheses or quotes.
```python
def my_function():
print("Hello world") # Missing closing quote
```
#### 2.2.2 Code Quality Checking
The code quality checking feature can detect potential issues in the code, such as unused variables or redundant code.
```python
def my_function(x):
if x == 0:
return 0
else:
return x # Redundant else branch
```
### 2.3 Code Refactoring
The code refactoring feature can help refactor the code structure to improve readability and maintainability.
#### 2.3.1 Renaming
The renaming feature can rename variables, functions, or classes.
```python
class MyClass:
def my_method(self):
pass
my_class = MyClass()
my_class.my_method() # Renamed to new_method
```
#### 2.3.2 Extracting Methods
The extract method feature can take a piece of code and extract it into a separate method, increasing the reusability of the code.
```python
def my_function():
print("Hello world")
print("Goodbye world")
my_function() # Extracted as print_message() method
```
### 2.4 Documentation Hints
The documentation hint feature can provide documentation information for functions, classes, or modules.
#### 2.4.1 Function Documentation
Function documentation hints can provide information about function parameters, return values, and usage.
```python
def my_function(x, y):
"""
Calculates the sum of x and y.
Args:
x: The first number
y: The second number
Returns:
The sum of x and y
"""
return x + y
my_function(1, 2) # View documentation hints
```
#### 2.4.2 Class Documentation
Class documentation hints can provide a description of the class, its methods, and attributes.
```python
class MyClass:
"""
A simple class.
Attributes:
name: The name of the class
"""
def __init__(self, name):
self.name = name
my_class = MyClass("John") # View documentation hints
```
# 3.1 Applying Code Completion
Code completion is one of the most commonly used code hinting features in PyCharm. It can help developers quickly complete code, thus improving development efficiency.
#### 3.1.1 Importing Modules and Classes
In PyCharm, you can quickly import modules and classes through code completion. The specific steps are as follows:
1. Type the name of the module or class in the code, then press `Ctrl` + `Space` keys.
2.
0
0
相关推荐
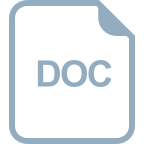
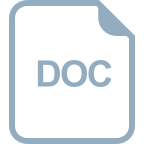
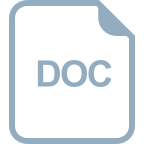
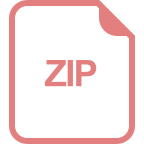
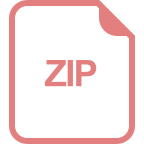
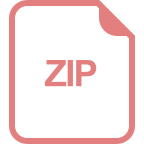
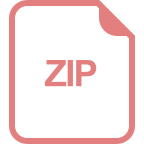
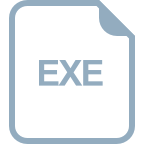