PyCharm Python Code Debugging Guide: Step-by-Step Tracking, Quick Issue Localization
发布时间: 2024-09-14 21:41:42 阅读量: 39 订阅数: 27 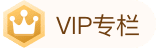
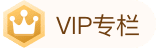
# 1. Overview of PyCharm Python Debugging
PyCharm is a powerful Integrated Development Environment (IDE) for Python, offering a comprehensive set of debugging tools that enable developers to easily identify and resolve issues in their code. Debugging is the process of identifying and fixing code defects, which is crucial for ensuring the correctness and reliability of applications.
The debugging features of PyCharm include breakpoints, watches, variable inspectors, and debug consoles. With these tools, developers can step through code, inspect variable values, and detect errors during code execution. Moreover, PyCharm offers advanced debugging capabilities such as conditional breakpoints, remote debugging, and integration with unit testing, allowing developers to delve deep into code behavior and tackle complex problems.
# 2. PyCharm Debugging Tools and Settings
### 2.1 Breakpoints and Watches
Breakpoints are one of the most important tools in a debugger, allowing us to pause execution when the code reaches a specific line. In PyCharm, breakpoints can be set in several ways:
- By clicking in the gray area to the left of the line number in the code.
- By right-clicking on a line of code and selecting "Toggle Breakpoint."
- By using the shortcut keys: Windows/Linux: F8, macOS: Fn+F8.
Once a breakpoint is set, PyCharm will pause the execution of the code when it reaches that line. We can use the debug console (introduced in the next section) to inspect variable values, modify the code, and resume execution.
Watches are another useful debugging tool that allows us to monitor the values of variables during debugging. To set a watch, right-click on a variable and choose "Add to Watches." The values of watched variables will be displayed in the "Watches" tab of the debug console.
### 2.2 Debugging Configurations and Run Modes
PyCharm allows us to configure different debugging scenarios with various debugging configurations. Debugging configurations define how the code is launched, breakpoints, watches, and other debugging options.
To create a debugging configuration, go to the "Run" menu and select "Edit Configurations...". In the "Debugger" tab, we can configure the following settings:
- **Script path:** The path to the Python script to be debugged.
- **Arguments:** Arguments to be passed to the script.
- **Working directory:** The working directory where the script is executed.
- **Environment variables:** Environment variables to be set.
- **Breakpoints:** A list of breakpoints to be set.
- **Watches:** A list of variables to be monitored.
PyCharm offers several run modes for debugging code:
- **Debug:** The most commonly used mode that allows us to pause and inspect variables while the code is executing.
- **Run:** Executes the code without pausing.
- **Pro***
***
***
***
***
***
***
***
***
***
***
```python
def my_function(a, b):
c = a + b
return c
# Set a breakpoint
breakpoint()
# Call the function
result = my_function(1, 2)
```
When the code execution reaches the breakpoint, PyCharm will pause. We can use the debug console to check variable values:
```
>>> a
1
>>> b
2
>>> c
3
```
We can modify the value of a variable and continue executing the code:
```
>>> c = 10
>>> continue
```
The code will continue executing, using the new value of c:
```
>>> result
10
```
# 3. Practical Python Code Debugging
### 3.1 Step-by-Step Debugging and Breakpoint Management
Step-by-step debugging is a process of executing code line by line and inspecting its state. It allows developers to view the execution of the code line by line and identify any issues or errors. In PyCharm, the following keyboard shortcuts can be used for step-by-step debugging:
- F8: Step over (execute the next line)
- F7: Step into (execute the next function call)
- F9: Step out (execute until the current function is finished)
Breakpoints are markers set in the code that cause the debugger to pause when execution reaches that point. This allows developers to inspect variable values or the flow of execution at specific points. Breakpoints can be set in PyCharm in the following ways:
1. Click the left edge next to the line number in the code.
2. Right-click on a line of code and choose "Toggle Breakpoint."
3. Click the "Add Breakpoint" button in the debug toolbar.
Breakpoints can be conditional, triggering only when a specific condition is met. This is particularly useful for debugging complex code or when you want to inspect variable values only under certain conditions. To set a conditional breakpoint, right-click on the breakpoin
0
0
相关推荐
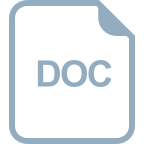
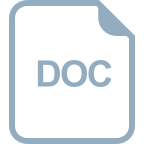
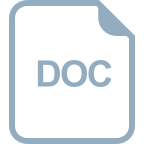
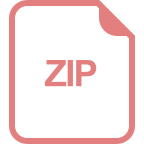
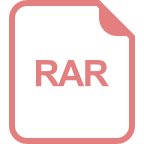
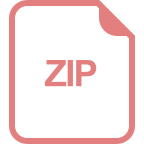
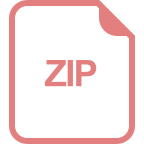
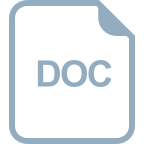
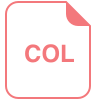