PyCharm Python Code Generation Guide: Boosting Development Efficiency
发布时间: 2024-09-14 21:48:44 阅读量: 8 订阅数: 18 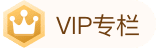
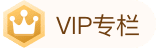
# 1. PyCharm Overview**
PyCharm is a powerful Python IDE that offers a variety of code generation tools and features to significantly boost development efficiency. PyCharm's code generation capabilities include:
***Code Templates:** Predefined code snippets that can be quickly inserted into code.
***Code Generation Tools:** For auto-generating classes, functions, docstrings, and other code elements.
***Advanced Code Generation:** Supports the creation of complex data structures and refactoring existing code.
# 2. Basic Code Generation
### 2.1 Code Templates and Shortcuts
#### 2.1.1 Using Code Templates
PyCharm offers a rich set of code templates to help you quickly generate common code structures. To use a code template, simply type the template's abbreviation in the editor and press the Tab key. For example, typing `def` and then pressing Tab will cause PyCharm to automatically generate a Python function template.
**Code Block:**
```python
def my_function(arg1, arg2):
"""
This is a sample function.
Args:
arg1: The first argument.
arg2: The second argument.
Returns:
The return value.
"""
pass
```
**Logical Analysis:**
This code block demonstrates how to use the `def` code template to generate a Python function. The function is named `my_function`, which takes two parameters, `arg1` and `arg2`. The function has a docstring that describes the function's purpose, parameters, and return value. The function body contains a `pass` statement, indicating that the function is not yet implemented.
#### 2.1.2 Creating and Managing Custom Templates
In addition to built-in templates, you can also create your own custom templates. To do so, navigate to `Settings` > `Editor` > `Code Templates`. In the `Templates` tab, click the `+` button to create a new template. Give your template a name and abbreviation, then input the template content.
**Code Block:**
```xml
<template name="My Custom Template" value="print($expr$)" description="Prints the expression" abbreviation="pct" />
```
**Logical Analysis:**
This code block demonstrates how to create a custom code template. The template is named `My Custom Template`, with an abbreviation of `pct`, and it prints the expression `$expr$` to the console.
### 2.2 Code Generation Tools
#### 2.2.1 Using Generators to Create Classes and Functions
PyCharm provides generators to help you quickly create classes and functions. To use a generator, right-click in the editor where you want to create the code, then select `Generate` > `Class` or `Generate` > `Function`. The generator will create a class or function template based on the context you select.
**Code Block:**
```python
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, {self.name}!")
```
**Logical Analysis:**
This code block demonstrates how to use a generator to create the `MyClass` class. The class has a constructor `__init__` that accepts a parameter `name` and stores it in the `self.name` attribute. The class also has a `greet` method that prints a message containing `self.name`.
#### 2.2.2 Generating Docstrings and Comments
PyCharm can assist you in generating docstrings and comments. To generate a docstring, place the cursor at the beginning of a function or class definition and press `Ctr
0
0
相关推荐
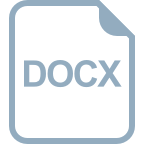
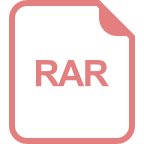





