PyCharm Python Code Search Guide: Fast Code Snippet Lookup
发布时间: 2024-09-14 21:54:35 阅读量: 39 订阅数: 34 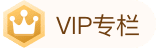
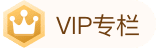
# 1. PyCharm Python Code Search Basics
PyCharm offers robust code search capabilities to help developers find and navigate code quickly and efficiently. This section will introduce the basics of PyCharm code search, including:
- **Search Scope:** Specify search directories and file types to narrow down the search area.
- **Search Conditions:** Perform advanced searches using text matching, regular expressions, and symbol names.
- **Search Results:** Filter and sort search results by file, symbol, or usage to quickly locate the required information.
# 2. PyCharm Code Search Tips
### 2.1 Setting Search Scope and Conditions
#### 2.1.1 Specifying Search Directories and File Types
In PyCharm, you can specify search directories and file types to narrow down the search area. In the "Find" toolbar, click on the "Directory" field and select the directory you wish to search. You can also further limit your search by clicking on the "File Type" field and selecting specific file types (e.g., ".py" or ".txt").
**Code Block:**
```python
find_in_path = "/path/to/directory"
file_type = ".py"
# Search for all .py files in the specified directory
PyCharm.find_in_path(find_in_path, file_type)
```
**Logical Analysis:**
This code block specifies searching for all ".py" files in the "/path/to/directory" directory. The `find_in_path()` function takes two parameters: the directory to search and the file type to search for.
#### 2.1.2 Advanced Search Using Regular Expressions
PyCharm supports advanced searches using regular expressions. In the "Find" toolbar, click on the "Use Regular Expressions" checkbox. This will allow you to specify a search pattern using regular expression syntax. For instance, to search for all words starting with "foo", you can use the following regular expression:
```
^foo
```
**Code Block:**
```python
regex = "^foo"
# Search using a regular expression
PyCharm.find(regex)
```
**Logical Analysis:**
This code block uses the regular expression "^foo" to search for all words starting with "foo". The `find()` function takes a regular expression string as a parameter.
### 2.2 Filtering and Sorting Search Results
#### 2.2.1 Filtering by File, Symbol, or Usage
After searching, you can filter results by file, symbol, or usage. In the "Find" toolbar, click the "Filter" button. This will open a dialog where you can select the conditions to filter by. For example, you can choose to display matches from a specific file or containing a specific symbol.
**Code Block:**
```python
filter_by_file = "main.py"
filter_by_symbol = "MyClass"
# Filter search results by file and symbol
PyCharm.find_and_filter(filter_by_file, filter_by_symbol)
```
**Logical Analysis:**
This code block filters search results by file ("main.py") and symbol ("MyClass"). The `find_and_filter()` function takes two parameters: the file to filter by and the symbol to filter by.
#### 2.2.2 Sorting by Name, Size, or Modification Date
You can also sort search results by name, size, or modification date. In the "Find" toolbar, click the "Sort" button. This will open a dialog where you can select sorting conditions. For instance, you can choose to sort results by name in ascending order or by modification date in descending order.
**Code Block:**
```python
sort_by_name = True
sort_order = "ascending"
# Sort search results by name in ascending order
PyCharm.sort_results(sort_by_name, sort_order)
```
**Logical Analysis:**
This code block sorts search results by name in ascending order. The `sort_results()` function takes two parameters: the sorting condition (`sort_by_name`) and the sorting order (`sort_order`).
### 2.3 Search History and Bookmark Management
#### 2.3.1 Viewing and Managing Search History
PyCharm saves your recent search history. To view the search history, click on the "History" button in the "Find" toolbar. This will open a dialog listing your recent searches. You can click on any search to perform it again.
**Code Block:**
```python
# View search history
PyCharm.view_history()
```
**Logical Analysis:**
This code block views the search history. The `view_history()` function opens a dialog that lists the most recent searches.
#### 2.3.2 Creating and Using Bookmarks
You can create bookmarks to save specific search results. To create a bookmark, right-click on a search result and select "Create Bookmark". This will create a bookmark that you can access anytime by clicking the "Bookmark" button in the "Find" toolbar.
**Code Block:**
```python
bookmark_name = "my_bookmark"
# Create a bookmark
PyChar
```
0
0