PyCharm Python Code Extraction Guide: Reusing Code, Boosting Development Efficiency
发布时间: 2024-09-14 21:57:20 阅读量: 25 订阅数: 34 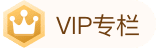
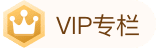
# 1. Basic Code Extraction with PyCharm
PyCharm's code extraction feature is a powerful tool that aids developers in reusing code, thus enhancing development efficiency. It allows users to extract code blocks or functions from existing code and store them as reusable components.
The first step in code extraction is selecting the code to be extracted. This can be accomplished using keyboard shortcuts or by selecting "Refactor" > "Extract" from the menu. Users can then specify the name and location of the extracted code block.
Extracted code blocks can be stored in a file or on the clipboard. If stored in a file, they can be imported into other projects. If stored on the clipboard, they can be pasted wherever needed.
# 2. Tips for Practicing Code Extraction
### 2.1 Code Block Extraction
Code block extraction involves taking a continuous section of code from the current file and placing it into a new file or module. This helps to organize related code together, improving code maintainability and reusability.
#### 2.1.1 Single-line Code Extraction
Extracting a single line of code is straightforward. Simply place the cursor on the line to be extracted and use the following shortcut keys:
```
Windows/Linux: Ctrl + Alt + C
macOS: Cmd + Option + C
```
This will bring up the "Extract Code" dialog, which offers the following options:
***
***
***
*** "OK" button to complete the extraction.
#### 2.1.2 Multi-line Code Extraction
To extract multiple lines of code, first select the code block you wish to extract, then use the same shortcut keys as for single-line extraction (Ctrl + Alt + C / Cmd + Option + C). In the "Extract Code" dialog, select the "File" option and specify the file where the extracted code should be saved.
### 2.2 Function Extraction
Function extraction refers to encapsulating a section of code into a function. This can enhance the readability, maintainability, and reusability of the code.
#### 2.2.1 Creating a New Function
To create a new function, first select the block of code to be encapsulated, then use the following shortcut keys:
```
Windows/Linux: Ctrl + Alt + M
macOS: Cmd + Option + M
```
This will bring up the "Extract Method" dialog, which includes the following options:
***Function Name:** Specify the name of the function to be created.
***Visibility:** Specify the function's visibility (public, protected, private).
***Return Type:** Specify the return type of the function.
***Parameters:** Specify the parameters of the function.
After selecting the desired options, click the "OK" button to complete the function extraction.
#### 2.2.2 Extracting Existing Code into a Function
To extract existing code into a function, first select the code block to be extracted, then use the same shortcut keys as for creating a new function (Ctrl + Alt + M / Cmd + Option + M). In the "Extract Method" dialog, select the "Existing Method" option and specify which existing function to encapsulate the extracted code in.
**Code Block:**
```python
# Define a function to calculate the sum of two numbers
def add_numbers(a, b):
return a + b
# Use the function to calculate the sum of two numbers
result = add_numbers(10, 20)
print(result) # Output: 30
```
**Logical Analysis:**
This code block defines a function named `add_numbers`, which takes two numbers as parameters and returns their sum. It then uses this function to calculate the sum of two numbers and print the result.
**Parameter Description:**
* `a`: The first number to be added.
* `b`: The second number to be added.
# 3. Best Practices for Code Extraction
### 3.1 Principle of Code Reusability
#### 3.1.1 DRY Principle
The DRY (Don't Repeat Yourself) principle is an important rule in software development, emphasizing the avoidance of repeating the same code segments in code. Repeated code can cause maintenance issues, as modifications need to be made in multiple places, increasing the likelihood of errors. Code extraction can effectively follow the DRY principle by extracting repeated code segments into functions or modules, which can be called when needed.
#### 3.1.2 Maintainability
Maintainability is a crucial property of code, referring to how easily the code can be understood, modified, and extended. Code extraction can enhance code maintainability by extracting complex or repeated code segments into separate functions or modules, making the code structure clearer and easier to understand and maintain.
### 3.2 Timing for Code Extraction
#### 3.2.1 When Code is Repeated
The best time for code extraction is when repeated code segments appear in the code. Repeated code segments not only cause maintenance problems but also affect the readability of the code. By extracting the repeated code segments into functions or modules, duplication can be eliminated, and the readability and maintainability of the code can be improved.
#### 3.2.2 When Code is Complex
When the code becomes complex, extracting code segments into functions or modules can help to simplify the code structure, ***plex code is often difficult to understand and maintain. By extracting code segments into separate functions or modules, complex code can be broken down into smaller, more manageable units, thus enhancing the understandability and maintainability of the code.
### Code Extraction Example
``
0
0
相关推荐








