PyCharm Python Code Renaming Guide: Safely and Efficiently Modify Code Identifiers
发布时间: 2024-09-14 21:55:31 阅读量: 19 订阅数: 28 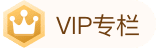
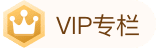
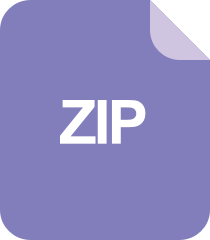
MWeb 3.1.1 for mac 免激活 激活码

# 1. PyCharm Python Code Refactoring Overview
PyCharm is a powerful integrated development environment (IDE) for Python, offering a wide range of code refactoring features to help improve code quality and maintainability. Code refactoring refers to the process of modifying the structure or organization of code without altering its behavior. PyCharm's refactoring tools assist in renaming identifiers, extracting methods, inlining variables, and more.
This chapter provides an overview of PyCharm's code refactoring capabilities and demonstrates how to utilize these features to optimize your Python code. We will cover the fundamental principles of refactoring, the available refactoring tools in PyCharm, and best practices for using these tools.
# 2. Fundamental Principles of Code Renaming
### 2.1 Principles and Best Practices for Renaming Identifiers
When renaming identifiers, it's crucial to follow these principles and best practices:
- **Clarity and simplicity:** The new identifier should clearly reflect its purpose and be as concise as possible.
- **Adhere to naming conventions:** Follow industry standards or team-specific naming conventions to ensure code readability and consistency.
- **Avoid reserved words:** Reserved words are identifiers with special meanings in Python and should not be used as variable or function names.
- **Use descriptive names:** Identifiers should describe the object or concept they represent.
- **Avoid abbreviations:** Abbreviations can confuse the readability of the code unless they are widely recognized industry standards.
### 2.2 Scope and Limitations of Renaming Identifiers
PyCharm's renaming tool allows you to rename identifiers within the following scope:
- **Local variables:** Variables visible only within a function or method.
- **Global variables:** Variables visible throughout a module.
- **Class attributes:** Attributes visible within a class and its subclasses.
- **Methods:** Methods defined within a class or module.
- **Functions:** Functions defined within a module.
- **Modules:** The Python file itself.
It is important to note the following limitations when renaming identifiers:
- **Cannot rename built-in identifiers:** Identifiers such as `True`, `False`, `None`, etc., cannot be renamed.
- **Cannot rename imported identifiers:** Identifiers imported from other modules cannot be renamed.
- **Cannot rename identifiers in use:** If an identifier is currently being used, it cannot be renamed.
# 3. PyCharm Rename Tool
### 3.1 Renaming a Single Identifier
PyCharm offers a convenient rename tool to easily rename individual identifiers. Follow these steps to rename an identifier:
1. Place the cursor on the identifier to be renamed.
2. Press `Ctrl` + `R` (Windows/Linux) or `Cmd` + `R` (macOS).
3. In the "Rename" dialog box that appears, enter the new identifier name.
4. Click the "Rename" button.
**Code Example:**
```python
def old_function_name(argument):
print(argument)
```
```python
# Using PyCharm Rename Tool
def new_function_name(argument):
print(argument)
```
**Logical Analysis:**
PyCharm's rename tool automatically updates all code lines referencing the identifier by analyzing the code context. In the example above, `old_function_name` is renamed to `new_function_name`, and all references in the code are correspondingly updated.
### 3.2 Renaming Multiple Identifiers
PyCharm also allows renaming multiple identifiers simultaneously. Follow these steps to rename multiple identifiers:
1. Select the identifiers to be renamed.
2. Press `Ctrl` + `Alt` + `R` (Windows/Linux) or `Cmd`
0
0
相关推荐
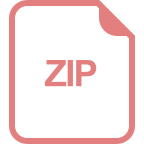
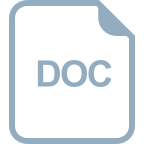
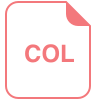
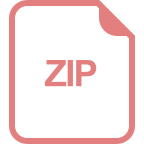
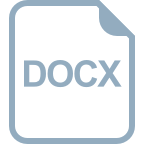
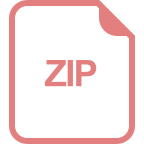