[PyCharm Python Environment Configuration Guide]: Step-by-Step Instructions for Building an Efficient Development Environment
发布时间: 2024-09-14 18:36:34 阅读量: 36 订阅数: 37 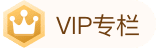
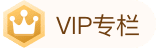
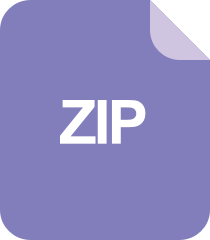
Python编译软件pycharm-community-2022.2.3.zip
**【PyCharm Python Environment Setup Guide】: Step-by-Step Instructions to Build an Efficient Development Setup**
PyCharm is a powerful Python development environment that offers a wealth of tools and functionalities to help developers work efficiently on Python code development and debugging. This chapter will cover the basic setup of the PyCharm Python environment, including the installation and configuration of Python interpreters, setting environment variables, and installing PyCharm plugins and tools.
### 1.1 Installation and Configuration of Python Interpreters
A Python interpreter is a program that executes Python code. In PyCharm, you can configure multiple Python interpreters to use different versions or environments of Python. When installing a Python interpreter, you must choose the correct version and platform. After installation, you need to configure the interpreter in PyCharm, including the interpreter's path, version, and environment variables.
### 2. Advanced Configuration of PyCharm Python Environment
#### 2.1 Configuration of Python Interpreters and Environment Variables
##### 2.1.1 Installation and Configuration of Python Interpreters
PyCharm supports multiple Python interpreters, including the official CPython, Anaconda, Miniconda, etc. When installing an interpreter, you can choose the appropriate version and environment according to the project's needs.
**Steps:**
1. Open PyCharm, go to the "File" menu, and select "Settings".
2. In the left navigation bar, choose "Project Interpreter".
3. Click the "Add" button, and select "Existing Interpreter".
4. Navigate to the installation directory of the Python interpreter and choose the interpreter executable file.
5. Click "OK" to complete the addition.
**Code Block:**
```python
import sys
# Get the path of the current Python interpreter
print(sys.executable)
```
**Logical Analysis:**
This code block uses `sys.executable` to get the path of the currently used Python interpreter and prints the output.
**Parameters Explanation:**
* `sys.executable`: Returns the path of the current Python interpreter.
##### 2.1.2 Setting and Management of Environment Variables
Environment variables are used to store system configuration information. PyCharm can specify the path to the Python interpreter and the installation directory of third-party libraries using environment variables.
**Steps:**
1. In PyCharm, go to the "File" menu, select "Settings".
2. In the left navigation bar, choose "Project Interpreter".
3. Click the "Show Path Variables" button.
4. In the pop-up dialog, you can add, edit, or delete environment variables.
**Code Block:**
```python
import os
# Get the value of an environment variable
print(os.environ["PATH"])
```
**Logical Analysis:**
This code block uses `os.environ["PATH"]` to get the value of the system environment variable `PATH` and prints the output.
**Parameters Explanation:**
* `os.environ["PATH"]`: Returns the value of the system environment variable `PATH`.
### 2.2 Installation of PyCharm Plugins and Tools
#### 2.2.1 Introduction and Installation of Common Plugins
PyCharm offers a variety of plugins to extend the IDE'***mon plugins include:
***CodeGlance**: Provides a visual representation of the code structure.
***Rainbow Brackets**: Colors brackets to enhance code readability.
***Material Theme**: Offers a modern IDE theme.
**Steps:**
1. In PyCharm, go to the "File" menu, select "Settings".
2. In the left navigation bar, choose "Plugins".
3. In the search box, enter the plugin name, search, and install the desired plugin.
#### 2.2.2 Configuration of Debugging and Testing Tools
PyCharm integrates powerful debugging and testing tools that can help developers quickly locate and solve problems.
**Debugging Tools:**
***Breakpoints**: Set breakpoints in the code, and the program will pause when it reaches a breakpoint.
***Debugger**: Provides an interactive debugging environment where you can inspect variable values and modify the code.
**Testing Tools:**
***Unit Tests**: Supports unit testing frameworks such as unittest, pytest, etc.
***Code Coverage**: Statistics and analysis of code coverage to identify untested code.
**Steps:**
1. In PyCharm, open the code file that needs debugging or testing.
2. Set breakpoints or use the debugger.
3. Run tests or view code coverage reports.
### 2.3 PyCharm Project Management and Version Control
#### 2.3.1 Creation and Management of Projects
PyCharm offers project management capabilities to create, open, close, and manage projects.
**Steps:**
1. Open PyCharm, go to the "File" menu, and select "New Project".
2. Choose the project type, enter the project name, and specify the location.
3. Click the "Create" button to create the project.
#### 2.3.2 Integration of Version Control Tools
PyCharm supports various version control tools such as Git, Mercurial, etc. Integrating version control tools allows for managing code changes and collaborative development.
**Steps:**
1. In PyCharm, open the project that requires version control.
2. Go to the "VCS" menu, select "Enable Version Control Integration".
3. Choose the version control tool you want to use and configure the related settings.
# 3.1 Code Editing and Debugging
#### 3.1.1 Code Completion and Syntax Highlighting
PyCharm offers powerful code completion features, which can automatically complete code snippets, functions, and class names, enhancing coding efficiency. It also supports syntax highlighting, where different syntax elements are displayed in different colors for easier code reading and understanding.
#### 3.1.2 Debugger and Breakpoint Settings
PyCharm integrates a debugger that allows developers to set breakpoints in the code, step through the code line by line, and inspect variable values. The debugger helps to locate and resolve issues in the code, enhancing development efficiency.
### 3.2 Unit Testing and Code Coverage
#### 3.2.1 Using Unit Testing Frameworks
PyCharm supports multiple unit testing frameworks such as unittest, pytest, and nose. Developers can write test cases in the code to verify its correctness. PyCharm offers a convenient test running interface that allows for quick execution of tests and viewing of results.
#### 3.2.2 Statistics and Analysis of Code Coverage
PyCharm can calculate and analyze code coverage, showing which lines of code are covered by test cases. Code coverage helps ensure the adequacy of the code, improving code quality.
### 3.3 Code Refactoring and Optimization
#### 3.3.1 Common Code Refactoring Operations
PyCharm offers a rich set of code refactoring operations, such as renaming variables, extracting methods, inlining variables, and moving code blocks. These operations can help optimize the code structure, improving code readability and maintainability.
#### 3.3.2 Strategies and Tips for Code Optimization
In addition to code refactoring, PyCharm also provides code optimization suggestions. Developers can use these suggestions to optimize code performance, reduce memory consumption, and enhance code readability.
# 4. PyCharm Python Project Deployment
### 4.1 Project Packaging and Distribution
#### 4.1.1 Packaging Tools and Configuration
PyCharm offers several packaging tools to package Python projects into executable files or distributable packages.
- **PyInstaller**: Used to package Python scripts into standalone executable files that can run on different platforms.
- **cx_Freeze**: Similar to PyInstaller but provides finer-grained control and smaller executable files.
- **Nuitka**: Compiles Python code into native code, thereby improving performance and reducing the size of the executable file.
**Configuring Packaging Tools:**
1. In PyCharm, open project settings (File -> Settings).
2. Go to the "Project Interpreter" tab.
3. In the "Packaging Tool" dropdown menu, select the desired packaging tool.
4. Configure additional options according to the documentation of the packaging tool.
#### 4.1.2 Project Release and Distribution
After packaging the project, it can be released and distributed to users.
- **PyPI**: The Python Package Index is the official repository for Python packages.
- **Conda**: A package manager system for managing Python packages and environments.
- **Private Repositories**: Used for storing and distributing private or internal projects.
**Release to PyPI:**
1. Register for a PyPI account.
2. Create a `setup.py` file containing project metadata and packaging instructions.
3. Use the `python setup.py sdist` and `python setup.py bdist_wheel` commands to create source distribution and binary wheel packages.
4. Use the `twine upload` command to upload the package to PyPI.
### 4.2 Docker Containerized Deployment
#### 4.2.1 Basic Concepts of Docker Containers
Docker is a containerization technology that isolates applications from the underlying infrastructure. Containers include all the code, runtime, and dependencies required to run the application.
**Advantages of Docker Containers:**
- **Portability**: Containers can run on any platform that supports Docker.
- **Isolation**: Containers are isolated from the host system, ensuring that the application is not affected by other processes.
- **Scalability**: Containers can be easily scaled to handle increased loads.
#### 4.2.2 Implementation of Python Project Containerization
The process of containerizing a Python project involves the following steps:
1. **Create a Dockerfile**: Define the build process of the container, including the base image, installed dependencies, and application code.
2. **Build Container Images**: Use the `docker build` command to build container images based on the Dockerfile.
3. **Run Containers**: Use the `docker run` command to run containers and specify port mappings and environment variables.
### 4.3 Cloud Platform Deployment
#### 4.3.1 Introduction and Selection of Cloud Platforms
Cloud platforms offer hosted services for deploying and managing applications. Popular cloud platforms include:
- **AWS**: Amazon Web Services
- **Azure**: Microsoft Azure
- **GCP**: Google Cloud Platform
**Factors for selecting a cloud platform:**
- **Features**: Services and features provided by the platform.
- **Cost**: Expenses involved in deploying and maintaining applications.
- **Support**: Technical support and documentation provided by the platform.
#### 4.3.2 Deployment of Python Projects on Cloud Platforms
Deploying Python projects on cloud platforms typically involves the following steps:
1. **Create Virtual Machines or Containers**: Create virtual machines or containers on the cloud platform to run Python applications.
2. **Configure Web Servers**: Install and configure web servers such as Apache or Nginx to host the application.
3. **Deploy Code**: Deploy Python code and dependencies to virtual machines or containers.
4. **Configure Databases**: If the application requires a database, configure and connect a database on the cloud platform.
5. **Monitoring and Management**: Use tools provided by the cloud platform to monitor and manage the application's performance and health.
# 5.1 Remote Development and Collaboration
### 5.1.1 Configuration of Remote Development Tools
**PyCharm Remote Development**
PyCharm offers built-in remote development tools that allow developers to develop and debug code on remote servers or cloud platforms.
**Configuration Steps:**
1. Install PyCharm Professional Edition.
2. In PyCharm, go to "File" -> "Settings" -> "Tools" -> "Remote Development".
3. Click the "Add" button and select "SSH Configuration".
4. Enter the IP address, username, and password of the remote server.
5. Test the connection and save the configuration.
**Visual Studio Code Remote Development**
Visual Studio Code also offers remote development extensions that allow developers to connect to remote servers or containers.
**Configuration Steps:**
1. Install the Visual Studio Code Remote Development extension.
2. In Visual Studio Code, select "View" -> "Command Palette".
3. Type "Remote-SSH: Connect to Host".
4. Enter the IP address, username, and password of the remote server.
5. Connect to the remote server and start developing.
### 5.1.2 Team Collaboration and Code Sharing
**Git and Version Control**
Git is a distributed version control system that allows team members to collaborate on code development and management.
**Configuration Steps:**
***
***
***mit code and create branches.
4. Push code to a remote repository (e.g., GitHub or GitLab).
**Collaboration Workflow:**
1. Developers clone the remote repository to their local machine.
2. Developers make changes on a local branch.
3. Developers commit the changes and push them back to the remote repository.
4. Other developers pull the latest changes and merge them into their own branches.
**Code Sharing Platforms**
Besides Git, there are many other code sharing platforms such as GitHub, Bitbucket, and GitLab. These platforms allow team members to share code, track issues, and collaborate on development.
0
0
相关推荐







