PyCharm Python Project Structure Analysis: Understanding the Project Layout for Enhanced Development Efficiency
发布时间: 2024-09-14 18:52:49 阅读量: 20 订阅数: 21 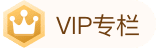
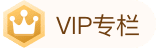
# 1. Overview of PyCharm Python Project Structure
PyCharm is a powerful Python Integrated Development Environment (IDE) that provides comprehensive project management features to help developers organize and manage Python projects efficiently. The project structure of PyCharm follows industry best practices and offers a range of tools and functionalities to optimize the project structure and enhance development efficiency.
This chapter will outline the PyCharm Python project structure, including its theoretical foundation, project structure management, and practical applications. We will explore the principles of project directory structures, common structures, project file management, project interpreter configuration, and the process of creating, importing, and customizing projects. By understanding PyCharm's project structure, developers can fully leverage the IDE's features to create and manage efficient and maintainable Python projects.
# 2. Theoretical Foundations of PyCharm Project Structure
### 2.1 Best Practices for Python Project Structure
#### 2.1.1 Principles of Project Directory Structure
A good Python project structure should follow these principles:
- **Modularity:** Divide the project into independent modules, with each module responsible for specific functionality.
- **Scalability:** Easily add new features and modules without affecting existing code.
- **Maintainability:** Easily understandable, modifiable, and debuggable.
- **Consistency:** Follow industry standards and best practices to ensure code readability and reusability.
#### 2.1.2 Common Project Directory Structures
The most common Python project directory structure includes:
- **Root Directory:** Contains the project's main files, such as `README.md`, `requirements.txt`, and `setup.py`.
- **Source Code Directory:** Contains the project's code, usually named `src` or `app`.
- **Test Directory:** Contains unit tests and integration tests.
- **Documentation Directory:** Contains user guides, API documentation, and other documents.
- **Data Directory:** Contains data files used by the project.
### 2.2 Project Structure Management in PyCharm
#### 2.2.1 Organization and Management of Project Files
PyCharm offers a range of features to organize and manage project files:
- **Project View:** Displays the project file tree, allowing users to browse, add, and delete files.
- **File Templates:** Provides predefined file templates for creating new Python files.
- **Code Folding:** Allows users to fold code blocks to improve readability and reduce scrolling.
- **Bookmarks:** Allows users to mark specific lines of code for quick navigation.
#### 2.2.2 Configuration and Management of Project Interpreters
PyCharm allows users to configure and manage multiple project interpreters:
- **Interpreter Configuration:** Users can specify the path, version, and environment variables of the interpreter.
- **Interpreter Selection:** Users can choose different interpreters within a project to run different Python versions or environments.
- **Interpreter Management:** PyCharm provides an interpreter management tool for installing, updating, and deleting interpreters.
```python
# Import necessary libraries
import os
# Get the current working directory
cwd = os.getcwd()
# Create a new Python project directory
os.mkdir('my_project')
# Change into the newly created directory
os.chdir('my_project')
# Create a new Python file
open('main.py', 'w').close()
# Create a new test directory
os.mkdir('tests')
# Create a new test file
open('tests/test_main.py', 'w').close()
```
**Code Logic Analysis:**
This code uses Python's `
0
0
相关推荐
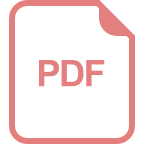
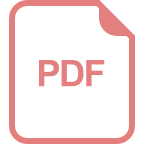
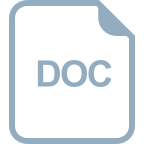
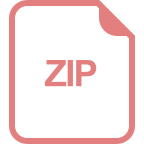
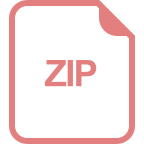
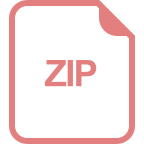
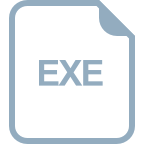
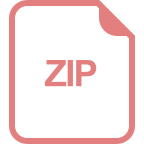