PyCharm Python Environment Configuration and Management: In-depth Understanding and Optimizing Development Workflow
发布时间: 2024-09-14 18:42:58 阅读量: 32 订阅数: 25 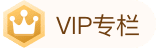
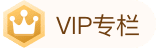
# 1. PyCharm Python Environment Setup and Management: A Deep Dive to Optimize the Development Workflow
## 1.1 PyCharm Installation and Setup
PyCharm is a powerful Python IDE that offers a range of tools and features to simplify Python development. To get started with PyCharm, you must first install it. You can download the latest version from the official website and choose the appropriate installer based on your operating system. Once installed, running PyCharm will guide you through the initial setup process.
## 1.2 Python Interpreter Selection and Configuration
PyCharm allows you to use different Python interpreters, including the system default interpreter, an interpreter in a virtual environment, or a custom interpreter. To select or configure a Python interpreter, navigate to "File" > "Settings" > "Project" > "Python Interpreter." Here, you can choose an existing interpreter or create and configure a new one.
# 2. Optimizing the Python Development Environment
## 2.1 Code Style and Formatting
### Code Style Guidelines
Code style guidelines are a set of conventions to ensure consistency and readability in your code. PyCharm offers several built-in code style guidelines, including PEP 8, Google Python Style Guide, and Airbnb Python Style Guide.
### Code Formatting
Code formatting tools can automatically format code according to style guidelines. PyCharm integrates code formatters such as Black and autopep8, which can format code with a single click.
```python
# Unformatted code
def my_function(a, b, c):
print(a + b + c)
```
```python
# Code formatted with Black
def my_function(a, b, c):
print(a + b + c)
```
## 2.2 Debugging and Error Handling
### Debugger
PyCharm comes with a robust debugger that allows developers to step through code line by line, inspect variable values, and set breakpoints.
### Breakpoints
Breakpoints are positions in the code where the debugger will pause execution when it reaches that point. Developers can use breakpoints to examine specific code paths or variable values.
```python
# Setting a breakpoint
def my_function(a, b):
breakpoint()
return a + b
```
### Error Handling
Error handling is the process of dealing with exceptions and errors that may occur in your code. PyCharm provides an error console to display error messages and stack traces.
```python
# Exception handling
try:
result = my_function(a, b)
except Exception as e:
print(e)
```
## 2.3 Performance Optimization and Analysis
### Performance Analysis
PyCharm offers profiling tools to identify performance bottlenecks in your code. These tools can analyze CPU and memory usage and generate performance reports.
### Optimization Techniques
Here are some common Python performance optimization techniques:
- Use list comprehensions and generator expressions instead of loops
- Use caching and memoization to store computed results
- Optimize data structures and algorithms
- Parallelize code
# 3. PyCharm Project Management
## 3.1 Project Structure and Organization
PyCharm provides robust project management features that enable developers to organize and manage their code effectively. A well-organized project structure is crucial for maintaining clarity, maintainability, and scalability of the codebase.
**Project Structure**
PyCharm projects typically consist of the following directories:
-
0
0
相关推荐
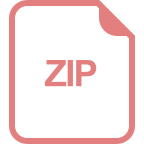
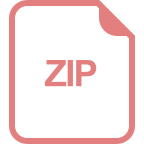
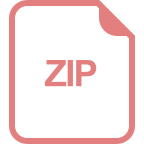
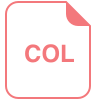
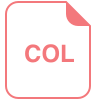
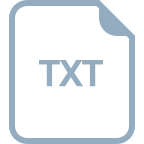
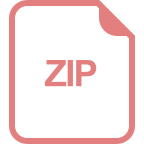
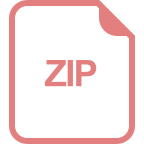
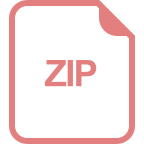