PyCharm Python Unit Testing: Writing and Running Unit Tests to Ensure Code Quality
发布时间: 2024-09-14 18:56:05 阅读量: 18 订阅数: 25 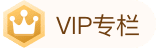
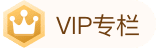
# 1. Overview of PyCharm Python Unit Testing
Unit testing is a crucial practice in software development that ensures the correctness of code blocks. PyCharm, a popular Python IDE, offers robust unit testing support, enabling developers to easily write, run, and debug test cases. This guide will introduce the basics of Python unit testing in PyCharm, including its concepts, writing principles, and specific implementations within PyCharm.
# 2. Writing PyCharm Python Unit Tests
### 2.1 Basics of Unit Testing
#### 2.1.1 Concepts and Functions of Unit Testing
Unit testing is a software testing technique used to verify the correctness of individual functionalities or modules within software. It involves writing test cases to examine the expected behavior of code, ensuring it functions as intended. Unit testing aids in early detection of errors during the development process, preventing their appearance in production environments.
#### 2.1.2 Principles for Writing Unit Tests
When writing unit tests, the following principles should be adhered to:
***Atomicity:** Each test case should test only a specific functionality.
***Independence:** Test cases should be independent of each other to avoid mutual influence.
***Repeatability:** Test cases should be able to run repeatedly at any time and produce the same results.
***Readability:** Test cases should be easy to understand and maintain.
### 2.2 Writing Unit Tests in PyCharm
#### 2.2.1 Creating Unit Test Files
Creating unit test files in PyCharm is straightforward. Right-click on the project directory, select "New" > "Python File," and append "_test" to the file name. For example, if you are testing `my_module.py`, the unit test file should be named `my_module_test.py`.
#### 2.2.2 Writing Test Cases
Test cases are the core of unit tests. They contain a set of assertions to check the expected behavior of the code. Below is a simple example of a test case:
```python
import unittest
class MyModuleTest(unittest.TestCase):
def test_add(self):
result = my_module.add(1, 2)
self.assertEqual(result, 3)
```
In this test case:
* `MyModuleTest` is a test class that inherits from `unittest.TestCase`.
* `test_add` is a test method that begins with "test_."
* `my_module.add` is the function being tested.
* `self.assertEqual` is an assertion used to check if `result` equals 3.
#### 2.2.3 Running Test Cases
Running test cases in PyCharm is also convenient. Here are a few methods:
***Using shortcuts:
0
0
相关推荐
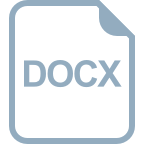
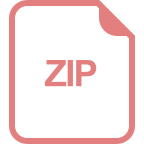





