Performance Impact of Insufficient Input Parameters in MATLAB: In-depth Analysis and Optimization Strategies
发布时间: 2024-09-14 14:38:32 阅读量: 26 订阅数: 31 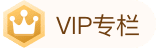
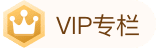
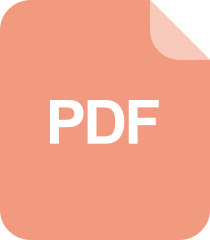
Pharmacologic treatment and behavior therapy: Allies in the management of hyperactive children
# 1. Overview of Insufficient Input Parameters in MATLAB
Insufficient input parameters in MATLAB refers to a scenario where a function is called without providing a sufficient number of parameters. This can lead to several issues:
- **Decreased Algorithm Efficiency:** Missing parameters might result in unnecessary calculations or incorrect execution of branches, lowering the algorithm's efficiency.
- **Increased Memory Consumption:** Missing parameters can lead to unnecessary variable allocation or redundancy in data structures, thereby increasing memory usage.
# 2. Impact of Insufficient Input Parameters on Performance
### 2.1 Decline in Algorithm Efficiency
Insufficient input parameters can result in a decrease in algorithm efficiency, which is mainly observed in the following two aspects:
#### 2.1.1 Unnecessary Calculations Due to Missing Parameters
When necessary input parameters are missing, an algorithm may not be able to execute correctly, leading to unnecessary calculations. For example, in image processing algorithms, without the image size parameter, the algorithm cannot determine the shape of the image, causing unnecessary loops and calculations.
#### 2.1.2 Incorrect Branch Execution Due to Missing Parameters
Missing parameters can also lead to incorrect branch execution, thereby reducing the efficiency of the algorithm. For instance, in numerical computation algorithms, without the convergence threshold parameter, the algorithm might not be able to accurately determine whether convergence conditions have been met, resulting in an infinite loop.
### 2.2 Increased Memory Consumption
Insufficient input parameters can also lead to increased memory consumption, which is mainly seen in the following two aspects:
#### 2.2.1 Unnecessary Variable Allocation Due to Missing Parameters
When necessary input parameters are missing, an algorithm may need to allocate unnecessary variables to store default values or temporary data. For example, in image processing algorithms, without the image data parameter, the algorithm might allocate an empty matrix to store image data, resulting in wasted memory space.
#### 2.2.2 Redundancy in Data Structures Due to Missing Parameters
Missing parameters can also lead to redundancy in data structures, increasing memory consumption. For example, in numerical computation algorithms, without the convergence threshold parameter, the algorithm might need to store convergence values for each iteration step, resulting in redundant data structures.
### Code Example
The following code example demonstrates the impact of insufficient input parameters on algorithm efficiency:
```matlab
function calculate_mean(data)
% Calculate the average of data
if nargin < 1
error('Missing input argument: data');
end
mean_value = sum(data) / length(data);
end
```
**Analysis of Code Logic:**
This code defines a function `calculate_mean` used to calculate the average of data. If the input parameter `data` is missing, the function will throw an error indicating a missing input argument.
**Parameter Explanation:**
* `data`: The input data, which should be a numerical array.
**Execution Logic:**
If the input parameter `data` is present, the function calculates the average of the data and stores it in the variable `mean_value`. Otherwise, the function throws an error indicating a missing input argument.
**Optimization Suggestions:**
To optimize algorithm efficiency, consider the following strategies:
* Use the `nargin` function to check the number of input parameters.
* Use `varargin` and `varargout` to collect variable arguments.
# 3. Optimization Strategies
### 3.1 Improving Parameter Checking
#### 3.1.1 Usin
0
0
相关推荐







