Video Tutorial on Insufficient MATLAB Input Parameters: Visually Learn and Understand Concepts
发布时间: 2024-09-14 14:47:45 阅读量: 25 订阅数: 31 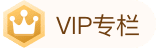
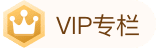
# 1. Overview of Insufficient Input Parameters in MATLAB
In MATLAB, functions generally require input parameters to perform their operations. However, sometimes a function may not receive all the required input parameters. This situation is known as insufficient input parameters.
Insufficient input parameters can cause the function to fail to execute correctly, or even produce errors. Therefore, it is essential to understand how to handle insufficient input parameters. This chapter will outline the concept of insufficient input parameters and discuss its impact in MATLAB.
# 2.1 Function Definition and Parameter Passing
In MATLAB, a function definition uses the `function` keyword, followed by the function name and a list of input parameters in parentheses. For example:
```matlab
function sum = mySum(x, y)
% Calculate the sum of x and y
sum = x + y;
end
```
In this function, `x` and `y` are input parameters. When the function is called, these parameters will be replaced with actual values. For instance:
```matlab
result = mySum(1, 2);
```
In this call, `1` and `2` are passed to `x` and `y`, and the function returns `3`.
### Methods of Parameter Passing
There are two ways to pass parameters in MATLAB:
***By value:** Basic data types (such as numbers, characters) are passed by value. This means that a copy of the parameter passed to the function is made, and any modifications the function makes to the parameter do not affect the original variable.
***By reference:** Objects (such as arrays, structs) are passed by reference. This means that a reference to the original variable is passed to the function, and any modifications the function makes to the parameter will affect the original variable.
### Default Parameter Values
Functions can specify default values for their input parameters. If no parameter value is provided when the function is called, the default value is used. For example:
```matlab
function mySum(x, y, z)
% Calculate the sum of x, y, and z, defaulting to 0 if z is not provided
if nargin < 3
z = 0;
end
sum = x + y + z;
end
```
In this function, `z` has a default value of `0`. If `z` is not provided when calling the function, `0` is used.
### Optional Parameters
Functions can also define optional parameters. Optional parameters allow the caller to provide or not provide a parameter value when calling the function. For example:
```matlab
function mySum(x, y, z)
% Calculate the sum of x, y, and z, defaulting to 0 if z is not provided
if nargin < 3
z = 0;
end
sum = x + y + z;
% If z is provided, print its value
if nargin == 3
fprintf('z = %d\n', z);
end
end
```
In this function, `z` is an optional parameter. If `z` is provided when calling the function, its value is printed.
### Parameter Validation and Error Handling
In MATLAB, the `nargin` and `nargout` functions can be used to c
0
0
相关推荐

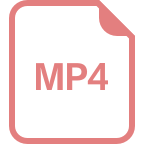
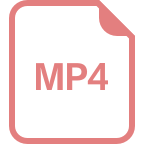





