Unveiling Insufficient MATLAB Input Parameters: From Error Messages to Comprehensive Solutions Guide
发布时间: 2024-09-14 14:32:42 阅读量: 37 订阅数: 22 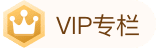
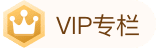
**Uncovering MATLAB Insufficient Input Parameters: From Error Messages to Comprehensive Solutions**
# 1. Error Messages for Insufficient Input Parameters in MATLAB
## 1.1 Meaning of Error Messages
Error messages indicating insufficient input parameters in MATLAB generally mean that the number of parameters provided during a function call is less than the number specified in the function definition. This causes the function to be unable to execute correctly and results in an error.
## 1.2 Common Error Message Examples
Here are some examples of common error messages for insufficient input parameters:
```
Error using <function_name> (line <line_number>)
Not enough input arguments.
```
```
Error using <function_name> (line <line_number>)
Function <function_name> expected at least <expected_number> input arguments, but only <provided_number> were provided.
```
# 2. Theoretical Roots of Insufficient Input Parameters
## 2.1 Definition and Parameter Passing Mechanism of MATLAB Functions
The definition of MATLAB functions follows this syntax:
```
function [output1, output2, ...] = function_name(input1, input2, ...)
```
Here, `function_name` is the name of the function, `input1`, `input2`, ... are the input parameters, and `output1`, `output2`, ... are the output parameters of the function.
MATLAB uses a **call-by-value** parameter passing mechanism, which means that the function receives copies of the input parameters, and modifications to these copies do not affect the original variables.
## 2.2 The Essence of Insufficient Input Parameters
Insufficient input parameters refer to a situation where the number of parameters provided during a function call is less than the number specified in the function definition. This results in MATLAB throwing an error message, such as:
```
Error: Not enough input arguments.
```
The essence of insufficient input parameters lies in:
* A function needs a certain number of parameters to run normally.
* When the number of provided parameters is insufficient, the function cannot obtain the necessary input information, resulting in an inability to perform the intended operation.
# 3.1 Checking Function Definitions and Documentation
## Checking Function Definitions
Errors related to insufficient input parameters often stem from a mismatch between the number of parameters defined in the function and the number of parameters actually passed. To resolve this issue, one must first check the function's definition.
```matlab
function myFunction(x, y)
% Function body
end
```
In this example, the `myFunction` function defines two input parameters: `x` and `y`. If only one parameter is passed when calling this function, an error related to insufficient input parameters will occur.
## Checking Function Documentation
The documentation of MATLAB functions provides detailed information about the required input and output parameters of the functions. By consulting the function documentation, one can understand the specific input parameters needed for a function.
```matlab
help myFunction
```
In the function documentation, the `Inputs` section lists the required input parameters. For the `myFunction` function, the documentation would show:
```
Inputs:
x - First input parameter
y - Second input parameter
```
By checking the function definitions and documentation, one can determine the required number of input parameters for a function and avoid errors related to insufficient input parameters.
# 4. Advanced Handling of Insufficient Input Parameters
### 4.1 Parameter Validation and Error Handling
In some cases, simply providing default parameter values or using a variable parameter list may not be enough. In such situations, stricter validation and error handling of input parameters are necessary to ensure the robustness and reliability of the function.
**Parameter Validation**
Parameter validation involves checking whether input parameters meet expected constraints before the function executes. This can prevent the function from producing unexpected results due to invalid or inconsistent parameters. MATLAB provides various functions for parameter validation, such as:
- `validateattributes`: Validates the type, size, range, and other attributes of input parameters.
- `narginchk`: Checks if the number of input parameters is within a specified range.
- `inputParser`: Creates a custom parameter parser, offering more flexible parameter validation and error handling.
**Code Block: Using `validateattributes` to Validate Parameters**
```matlab
function myFunction(x, y)
% Validate the type and range of input parameters
validateattributes(x, {'numeric'}, {'scalar', 'positive'});
validateattributes(y, {'numeric'}, {'vector', 'nonempty'});
end
```
**Logical Analysis:**
This code block uses the `validateattributes` function to validate the type and range of input parameters `x` and `y`. `x` must be a positive scalar number, and `y` must be a non-empty numeric vector. If any parameter does not meet these constraints, the function will throw a `MATLAB:validateattributes:InvalidValue` error.
**Error Handling**
Error handling refers to capturing and processing errors during the execution of a function. This can prevent the function from crashing due to unexpected errors and allows the program to recover gracefully or provide meaningful error messages. MATLAB provides the `try-catch` statement for error handling:
- The `try` block contains code that may raise an error.
- The `catch` block captures and processes the error.
**Code Block: Using `try-catch` for Error Handling**
```matlab
function myFunction(x, y)
try
% Function body
catch ME
% Handle errors
disp(ME.message);
end
end
```
**Logical Analysis:**
This code block uses the `try-catch` statement to capture any errors that occur during the execution of the function. If an error occurs, the `catch` block will catch the error message and display it in the console.
### 4.2 Type Checking of Input Parameters
Besides validating parameter constraints, one can also check the types of input parameters. This ensures that the function only accepts parameters of specific types and prevents errors due to type mismatches.
**Code Block: Using `isa` to Check Parameter Types**
```matlab
function myFunction(x)
if ~isa(x, 'double')
error('Input parameter must be a double-precision number.');
end
end
```
**Logical Analysis:**
This code block uses the `isa` function to check if the input parameter `x` is a double-precision floating-point number. If not, the function will throw a `MATLAB:error` error with a meaningful error message.
# ***mon Scenarios of Insufficient Input Parameters in MATLAB
In practical applications, the problem of insufficient input parameters in MATLAB may occur in the following common scenarios:
### 5.1 Function Overloading
MATLAB allows multiple overloaded versions of the same function name, each accepting a different number or type of input parameters. If a overloaded function is called but the provided input parameters do not match any defined version, an error related to insufficient input parameters will occur.
**Example:**
```
function sum(a, b)
% Calculate the sum of two numbers
result = a + b;
end
function sum(a, b, c)
% Calculate the sum of three numbers
result = a + b + c;
end
% Call the function, but only provide two parameters
result = sum(1, 2); % Insufficient input parameters, as the overloaded version requires three parameters
```
**Solution:**
* Carefully check the function documentation to understand the input parameter requirements for different overloaded versions.
* Provide the correct number of input parameters as needed.
### 5.2 Nested Functions
Nested functions are defined within another function. When calling a nested function, it can access the local variables of the outer function. However, if the input parameters for the nested function are insufficient, an error will occur.
**Example:**
```
function outerFunction()
a = 1;
b = 2;
function innerFunction(c)
% Use the local variables of the outer function
result = a + b + c;
end
% Call the nested function, but only provide one parameter
result = innerFunction(3); % Insufficient input parameters, as the nested function requires two parameters
```
**Solution:**
* Ensure that the number of input parameters for the nested function matches the function definition.
* Provide all required input parameters when calling the nested function.
### 5.3 Anonymous Functions
Anonymous functions are defined using the `@(arg1, arg2, ...) expression` syntax. Like named functions, anonymous functions may also require input parameters. If the provided input parameters are insufficient, an error will occur.
**Example:**
```
% Define an anonymous function
sumFunction = @(a, b) a + b;
% Call the anonymous function, but only provide one parameter
result = sumFunction(1); % Insufficient input parameters, as the anonymous function requires two parameters
```
**Solution:**
* Carefully check the definition of the anonymous function to understand its input parameter requirements.
* Provide all required input parameters when calling the anonymous function.
# 6. Best Practices for Insufficient Input Parameters in MATLAB**
To avoid errors related to insufficient input parameters and to write robust MATLAB code, it is recommended to follow these best practices:
- **Clear Function Documentation:** Clearly state the required input parameters in the function documentation, including the names, types, and default values of the parameters. This helps users understand the expected behavior of the function and avoids errors related to insufficient input parameters.
- **Robust Parameter Handling:** Use parameter validation and error handling mechanisms to check the validity of input parameters. MATLAB provides functions like `nargin` and `varargin` to check the number and type of input parameters. If insufficient input parameters are detected, errors can be thrown or default values can be used.
- **Avoid Traps for Insufficient Input Parameters:** Avoid using optional parameters or default parameter values in functions, as this may lead to errors related to insufficient input parameters. If optional parameters are needed, use variable parameter lists or overloaded functions.
- **Use Parameter Validation Functions:** MATLAB provides the `validateattributes` function to verify the type, range, and size of input parameters. This helps ensure the validity of input parameters and prevents errors related to insufficient input parameters.
- **Use Type Checking:** Use functions like `isnumeric`, `ischar`, and `islogical` to check the types of input parameters. This helps ensure that input parameters match the expected data types of the function and prevents errors related to insufficient input parameters.
- **Use Error Handling:** Use `try` and `catch` blocks to handle errors related to insufficient input parameters. If insufficient input parameters are detected, custom errors can be thrown or default values can be used. This helps provide meaningful error messages and prevent code from crashing.
By following these best practices, robust MATLAB code can be written, errors related to insufficient input parameters can be avoided, and the reliability and maintainability of the code can be ensured.
0
0
相关推荐
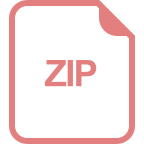
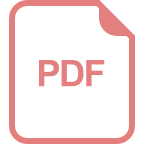
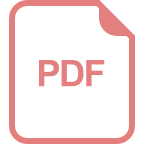
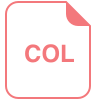
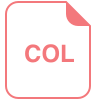
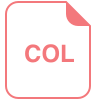
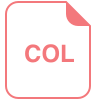
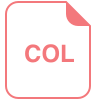
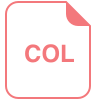
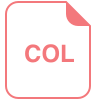