用C语言写个代码:客户到银行存1年期的定期存款。请编写程序,输入1年期定期存款利率和存款金额,帮客户计算到期本金、利息和合计金额。
时间: 2024-05-14 13:13:01 浏览: 99
```c
#include <stdio.h>
int main() {
float rate, amount, interest, total;
int year = 1; // 存1年期
printf("请输入1年期定期存款利率(%):");
scanf("%f", &rate);
printf("请输入存款金额(元):");
scanf("%f", &amount);
interest = amount * rate / 100 * year;
total = amount + interest;
printf("到期本金为:%.2f元\n", amount);
printf("利息为:%.2f元\n", interest);
printf("合计金额为:%.2f元\n", total);
return 0;
}
```
相关问题
客户到银行存1年期的定期存款。请编写c程序,输入1年期定期存款利率和存款金额,帮客户计算到期本金、利息和合计金额。
好的,以下是计算到期本金、利息和合计金额的C语言代码:
```c
#include <stdio.h>
int main() {
float rate, amount, interest, total;
int year = 1;
printf("请输入1年期定期存款利率和存款金额:\n");
scanf("%f%f", &rate, &amount);
interest = amount * rate * year;
total = amount + interest;
printf("到期本金为:%.2f元\n", amount);
printf("利息为:%.2f元\n", interest);
printf("合计金额为:%.2f元\n", total);
return 0;
}
```
编写c语言程序计算存款利息。有1000元,想存一年。有3种方法可选:(1)活期,年利率为r1;(2)一年定期,年利率为r2;(3)存两次半年定期,年利率为r3。请分别计算出一年后按3种方法所得到的本息和。
```c
#include<stdio.h>
#include<math.h>
int main()
{
float r1, r2, r3;
float amount1, amount2, amount3;
float principal = 1000.0;
int year = 1;
printf("请输入三种存款的年利率(如0.01表示1%%):\n");
printf("活期:");
scanf("%f", &r1);
printf("一年定期:");
scanf("%f", &r2);
printf("半年定期:");
scanf("%f", &r3);
// 活期计算
amount1 = principal * (1 + r1);
printf("活期本息和:%f\n", amount1);
// 一年定期计算
amount2 = principal * pow(1 + r2, year);
printf("一年定期本息和:%f\n", amount2);
// 半年定期计算
float half_year_rate = r3 / 2.0;
float first_half_year_amount = principal * pow(1 + half_year_rate, 2);
amount3 = first_half_year_amount * pow(1 + half_year_rate, 2);
printf("半年定期本息和:%f\n", amount3);
return 0;
}
```
阅读全文
相关推荐
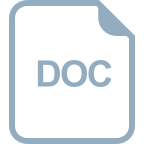
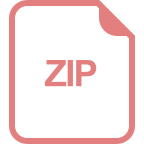
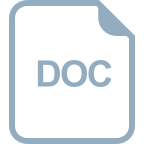
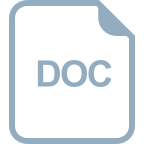
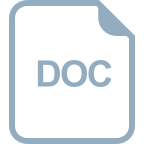
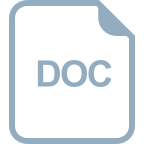
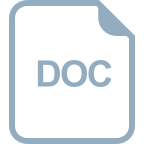
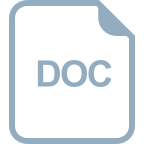






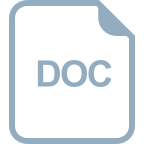