1、有P1,P2,P3三个进程,P1和P2负责从键盘接收字符串,均发送给P3,P3接收到字符串,根据发送方分别显示”P3 received *** from P1(或P2)" ;用消息队列实现
时间: 2024-02-06 13:09:47 浏览: 151
下面是使用消息队列实现题目要求的C语言代码,其中用到了Linux系统的IPC机制:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_TYPE1 1
#define MSG_TYPE2 2
struct msgbuf {
long mtype;
char mtext[1024];
};
int main() {
int mqid1, mqid2;
key_t key1, key2;
struct msgbuf buf;
pid_t pid;
// 创建两个消息队列
key1 = ftok(".", 'a');
mqid1 = msgget(key1, IPC_CREAT|0666);
if(mqid1 < 0) {
perror("msgget error");
exit(1);
}
key2 = ftok(".", 'b');
mqid2 = msgget(key2, IPC_CREAT|0666);
if(mqid2 < 0) {
perror("msgget error");
exit(1);
}
// 创建P1进程
pid = fork();
if(pid == -1) {
perror("fork error");
exit(1);
} else if(pid == 0) { // 子进程P1
printf("P1 process\n");
while(1) {
memset(&buf, 0, sizeof(struct msgbuf));
buf.mtype = MSG_TYPE1;
fgets(buf.mtext, sizeof(buf.mtext), stdin);
msgsnd(mqid1, &buf, sizeof(buf.mtext), 0);
}
}
// 创建P2进程
pid = fork();
if(pid == -1) {
perror("fork error");
exit(1);
} else if(pid == 0) { // 子进程P2
printf("P2 process\n");
while(1) {
memset(&buf, 0, sizeof(struct msgbuf));
buf.mtype = MSG_TYPE2;
fgets(buf.mtext, sizeof(buf.mtext), stdin);
msgsnd(mqid2, &buf, sizeof(buf.mtext), 0);
}
}
// 父进程P3
printf("P3 process\n");
while(1) {
memset(&buf, 0, sizeof(struct msgbuf));
msgrcv(mqid1, &buf, sizeof(buf.mtext), MSG_TYPE1, 0);
printf("P3 received %s from P1\n", buf.mtext);
memset(&buf, 0, sizeof(struct msgbuf));
msgrcv(mqid2, &buf, sizeof(buf.mtext), MSG_TYPE2, 0);
printf("P3 received %s from P2\n", buf.mtext);
}
return 0;
}
```
程序中首先创建了两个消息队列,分别用于P1和P2向P3发送消息。接着创建了两个子进程P1和P2,它们分别从键盘读取字符串,并将其发送到相应的消息队列中。最后父进程P3从两个消息队列中接收消息,并根据发送方分别显示接收到的字符串。
需要注意的是,消息队列的发送和接收操作都是阻塞的,因此程序会一直阻塞在相应的函数调用处,直到有消息到来。如果要退出程序,可以使用Ctrl+C等方式发送SIGINT信号,或者在代码中添加相应的信号处理函数来实现优雅地退出。
阅读全文
相关推荐
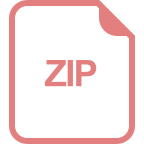











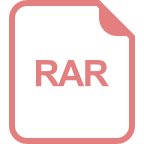
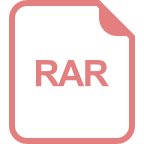
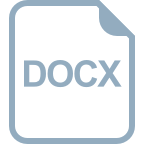