3、有P1,P2,P3三个进程,P1和P2负责从键盘接收字符串,均发送给P3,P3接收到字符串,根据发送方分别显示”P3 received *** from P1(或P2)" ;用c语言共享存储通信方式实现
时间: 2024-05-15 13:14:29 浏览: 144
以下是使用共享内存实现进程间通信的示例代码:
P1进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main() {
int shmid;
char *shmaddr;
char input[SHM_SIZE];
// 创建共享内存
shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
// 将共享内存连接到当前进程的地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
while (1) {
// 从键盘读取输入
printf("P1: ");
fgets(input, SHM_SIZE, stdin);
// 将输入写入共享内存
strncpy(shmaddr, input, SHM_SIZE);
// 发送信号,通知P3读取共享内存中的数据
kill(getpid() + 2, SIGUSR1);
}
// 分离共享内存
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
// 删除共享内存
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
P2进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main() {
int shmid;
char *shmaddr;
char input[SHM_SIZE];
// 创建共享内存
shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
// 将共享内存连接到当前进程的地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
while (1) {
// 从键盘读取输入
printf("P2: ");
fgets(input, SHM_SIZE, stdin);
// 将输入写入共享内存
strncpy(shmaddr, input, SHM_SIZE);
// 发送信号,通知P3读取共享内存中的数据
kill(getpid() + 2, SIGUSR1);
}
// 分离共享内存
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
// 删除共享内存
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
P3进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <signal.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
void handler(int signo);
int main() {
int shmid;
char *shmaddr;
char input[SHM_SIZE];
// 创建共享内存
shmid = shmget(IPC_PRIVATE, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
// 将共享内存连接到当前进程的地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
// 注册信号处理函数
signal(SIGUSR1, handler);
while (1) {
// 等待P1和P2发送信号
pause();
// 读取共享内存中的数据
strncpy(input, shmaddr, SHM_SIZE);
// 根据发送方显示消息
if (getpid() == (getppid() + 1)) {
printf("P3 received %s from P1\n", input);
} else if (getpid() == (getppid() + 2)) {
printf("P3 received %s from P2\n", input);
}
}
// 分离共享内存
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
// 删除共享内存
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(EXIT_FAILURE);
}
return 0;
}
void handler(int signo) {
// 什么也不做,只是用来唤醒pause函数
}
```
阅读全文
相关推荐
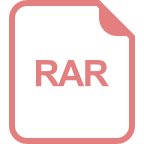










