Linux 队列实现生产者和消费者C语言实例
时间: 2023-10-21 12:03:37 浏览: 47
下面是一个简单的基于 Linux 队列的生产者和消费者 C 语言实例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <mqueue.h>
#include <errno.h>
#include <unistd.h>
#define QUEUE_NAME "/my_queue"
#define MAX_MESSAGES 10
#define MAX_MSG_SIZE 256
#define MSG_BUFFER_SIZE MAX_MSG_SIZE + 10
int main(int argc, char **argv) {
mqd_t mq;
struct mq_attr attr;
char buffer[MSG_BUFFER_SIZE];
int terminate = 0;
// 设置消息队列属性
attr.mq_flags = 0;
attr.mq_maxmsg = MAX_MESSAGES;
attr.mq_msgsize = MAX_MSG_SIZE;
attr.mq_curmsgs = 0;
// 创建消息队列
mq = mq_open(QUEUE_NAME, O_CREAT | O_RDONLY, 0644, &attr);
if (mq == -1) {
perror("mq_open");
exit(1);
}
// 消费者循环等待消息
while (!terminate) {
ssize_t bytes_read;
bytes_read = mq_receive(mq, buffer, MSG_BUFFER_SIZE, NULL);
if (bytes_read == -1) {
perror("mq_receive");
exit(1);
}
buffer[bytes_read] = '\0';
if (strncmp(buffer, "exit", strlen("exit")) == 0) {
terminate = 1;
} else {
printf("Received message: %s\n", buffer);
}
}
// 关闭消息队列
mq_close(mq);
mq_unlink(QUEUE_NAME);
return 0;
}
```
生产者代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <mqueue.h>
#include <errno.h>
#include <unistd.h>
#define QUEUE_NAME "/my_queue"
#define MAX_MESSAGES 10
#define MAX_MSG_SIZE 256
#define MSG_BUFFER_SIZE MAX_MSG_SIZE + 10
int main(int argc, char **argv) {
mqd_t mq;
char buffer[MSG_BUFFER_SIZE];
int terminate = 0;
// 打开消息队列
mq = mq_open(QUEUE_NAME, O_WRONLY);
if (mq == -1) {
perror("mq_open");
exit(1);
}
// 生产者循环发送消息
while (!terminate) {
printf("Enter message to send (exit to terminate): ");
fgets(buffer, MSG_BUFFER_SIZE, stdin);
if (strncmp(buffer, "exit", strlen("exit")) == 0) {
terminate = 1;
} else {
ssize_t bytes_sent;
bytes_sent = mq_send(mq, buffer, strlen(buffer) + 1, 0);
if (bytes_sent == -1) {
perror("mq_send");
exit(1);
}
}
}
// 发送终止消息
if (mq_send(mq, "exit", strlen("exit") + 1, 0) == -1) {
perror("mq_send");
exit(1);
}
// 关闭消息队列
mq_close(mq);
return 0;
}
```
这个例子中,生产者循环等待用户输入消息并将其发送到消息队列中。消费者循环等待从消息队列中接收消息并将其打印到控制台上。当用户输入 "exit" 时,生产者发送一个终止消息,消费者收到终止消息后退出循环并关闭消息队列。
相关推荐
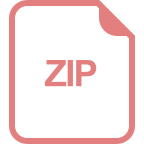
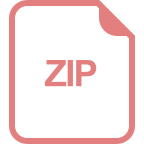
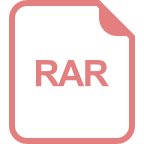
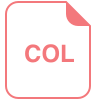
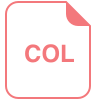












