写一个贪吃蛇游戏,c语言
时间: 2023-07-13 12:31:58 浏览: 56
好的,这是一个简单的贪吃蛇游戏的C语言代码,希望能帮到你。
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define ROW 20
#define COL 20
#define INIT_LENGTH 3
typedef struct snake
{
int x;
int y;
struct snake *next;
} Snake;
void init_map(int map[][COL], Snake *snake_head)
{
for (int i = 0; i < ROW; i++)
{
for (int j = 0; j < COL; j++)
{
if (i == 0 || i == ROW - 1 || j == 0 || j == COL - 1)
{
map[i][j] = 1;
}
else
{
map[i][j] = 0;
}
}
}
Snake *p = snake_head;
for (int i = 0; i < INIT_LENGTH; i++)
{
map[p->x][p->y] = 2;
p = p->next;
}
}
void print_map(int map[][COL])
{
system("cls");
for (int i = 0; i < ROW; i++)
{
for (int j = 0; j < COL; j++)
{
if (map[i][j] == 0)
{
printf(" ");
}
else if (map[i][j] == 1)
{
printf("# ");
}
else if (map[i][j] == 2)
{
printf("* ");
}
else if (map[i][j] == 3)
{
printf("+ ");
}
}
printf("\n");
}
}
void create_food(int map[][COL])
{
int x, y;
do
{
x = rand() % (ROW - 2) + 1;
y = rand() % (COL - 2) + 1;
} while (map[x][y] != 0);
map[x][y] = 3;
}
void init_snake(Snake *snake_head)
{
Snake *p = snake_head;
for (int i = 0; i < INIT_LENGTH; i++)
{
p->x = ROW / 2;
p->y = COL / 2 - i;
p->next = (Snake *)malloc(sizeof(Snake));
p = p->next;
}
p->next = NULL;
}
int move(Snake *snake_head, int map[][COL], int direction)
{
Snake *p = snake_head;
int x = p->x, y = p->y;
if (direction == 'w')
{
x--;
}
else if (direction == 's')
{
x++;
}
else if (direction == 'a')
{
y--;
}
else if (direction == 'd')
{
y++;
}
if (map[x][y] == 1 || map[x][y] == 2)
{
return -1;
}
if (map[x][y] == 3)
{
map[x][y] = 2;
Snake *new_node = (Snake *)malloc(sizeof(Snake));
new_node->x = x;
new_node->y = y;
new_node->next = NULL;
while (p->next)
{
p = p->next;
}
p->next = new_node;
create_food(map);
}
else
{
map[p->x][p->y] = 0;
map[x][y] = 2;
p->x = x;
p->y = y;
while (p->next)
{
int temp_x = p->next->x;
int temp_y = p->next->y;
p->next->x = x;
p->next->y = y;
x = temp_x;
y = temp_y;
p = p->next;
}
}
return 0;
}
int main()
{
int map[ROW][COL];
Snake *snake_head = (Snake *)malloc(sizeof(Snake));
init_snake(snake_head);
init_map(map, snake_head);
create_food(map);
print_map(map);
int direction = 'd';
while (1)
{
if (_kbhit())
{
int key = _getch();
if (key == 'w' || key == 's' || key == 'a' || key == 'd')
{
if ((direction == 'w' && key == 's') || (direction == 's' && key == 'w') || (direction == 'a' && key == 'd') || (direction == 'd' && key == 'a'))
{
continue;
}
direction = key;
}
}
int ret = move(snake_head, map, direction);
if (ret == -1)
{
printf("GAME OVER\n");
break;
}
print_map(map);
Sleep(200);
}
return 0;
}
```
这个代码使用了链表来表示贪吃蛇的身体,使用了随机数来生成食物,使用了`_kbhit()`和`_getch()`函数来获取键盘输入,使用了`Sleep()`函数来让程序暂停一段时间。在Windows下编译运行即可。
相关推荐
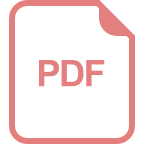
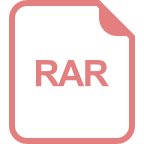
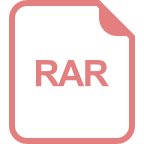
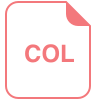
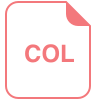
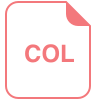
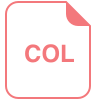
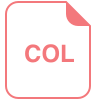





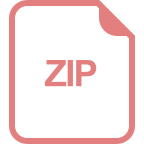
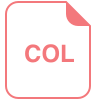
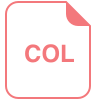