给我找一个用java实现hadoop的mapreduce编程的教程文档
时间: 2024-06-07 12:05:22 浏览: 97
请参考以下链接,里面详细介绍了如何使用Java实现Hadoop的MapReduce编程:
https://hadoop.apache.org/docs/stable/hadoop-mapreduce-client/hadoop-mapreduce-client-core/MapReduceTutorial.html
相关问题
帮我搭建Hadoop单机版,使用MapReduce解决姓名分析问题
好的,以下是基本的步骤:
1. 安装Java环境
首先需要安装Java环境,可以从Oracle官网下载并安装。
2. 下载Hadoop
可以从Hadoop官网下载并解压缩Hadoop二进制包。
3. 配置Hadoop环境变量
在系统环境变量中添加Hadoop的bin目录,例如在Linux系统中可以在/etc/profile文件中添加以下内容:
```
export HADOOP_HOME=/path/to/hadoop
export PATH=$PATH:$HADOOP_HOME/bin
```
4. 配置Hadoop单机版模式
在Hadoop的配置文件中,修改以下配置项:
- core-site.xml:
```
<configuration>
<property>
<name>fs.defaultFS</name>
<value>hdfs://localhost:9000</value>
</property>
</configuration>
```
- hdfs-site.xml:
```
<configuration>
<property>
<name>dfs.replication</name>
<value>1</value>
</property>
</configuration>
```
- mapred-site.xml:
```
<configuration>
<property>
<name>mapreduce.framework.name</name>
<value>yarn</value>
</property>
<property>
<name>yarn.app.mapreduce.am.env</name>
<value>HADOOP_MAPRED_HOME=/path/to/hadoop</value>
</property>
<property>
<name>mapreduce.map.env</name>
<value>HADOOP_MAPRED_HOME=/path/to/hadoop</value>
</property>
<property>
<name>mapreduce.reduce.env</name>
<value>HADOOP_MAPRED_HOME=/path/to/hadoop</value>
</property>
</configuration>
```
5. 启动Hadoop
在终端中进入Hadoop的bin目录,执行以下命令启动Hadoop:
```
$ start-all.sh
```
6. 准备数据
在Hadoop的文件系统中创建一个输入目录,将待处理的数据文件上传到该目录下。
7. 编写MapReduce程序
使用Java编写MapReduce程序,实现姓名分析的功能。可以参考官方文档或者其他教程学习MapReduce编程。
8. 打包程序并提交任务
将编写好的程序打包成jar包,并将jar包上传到Hadoop的文件系统中。执行以下命令提交任务:
```
$ hadoop jar /path/to/your/jar/file.jar input output
```
其中input为输入目录,output为输出目录。
9. 查看任务执行结果
在Hadoop的web界面中查看任务的执行情况和结果。可以通过以下命令访问web界面:
```
$ hadoop dfsadmin -report
```
以上就是基本的Hadoop单机版搭建和MapReduce程序开发的步骤。
给我一篇关于Spark和Hadoop实验总结报告
Spark和Hadoop实验总结报告
一、实验背景
本次实验是为了了解分布式系统中的大数据处理工具,主要学习Apache Spark和Hadoop两个分布式大数据处理工具的使用和比较。
二、实验目的
1. 了解Apache Spark和Hadoop大数据处理框架的基本原理和架构;
2. 学习使用Spark和Hadoop处理大规模数据;
3. 掌握Spark和Hadoop的优缺点比较。
三、实验内容
1. 安装配置Hadoop和Spark;
2. 学习Hadoop和Spark的基本概念和使用方法;
3. 使用Hadoop和Spark进行数据处理;
4. 分析比较Hadoop和Spark的优缺点。
四、实验步骤
1. 安装配置Hadoop和Spark
我采用的是在Ubuntu 18.04系统下使用wget工具下载并解压Hadoop、Spark等软件包进行安装配置。安装配置的过程中主要遇到了如下几个问题:
(1) Hadoop配置文件修改:Hadoop的配置文件许多,操作起来较为繁琐,需要仔细阅读并修改配置文件信息。
(2) Spark的环境变量配置:Spark需要在bashrc文件做全局环境变量的配置。我遇到过Spark安装完成后,hadoop-daemon.sh start namenode启动失败的问题,其中还涉及到了Java的配置问题。最后通过重启Ubuntu系统和再次配置后解决。
2. 学习Hadoop和Spark基本概念和使用方法
Hadoop和Spark都是分布式大数据处理的工具。Hadoop分为两个子项目:HDFS和MapReduce,其中HDFS是分布式文件系统,MapReduce是分布式计算框架;而Spark则是一种快速、通用的集群计算系统,可以支持内存计算,对于迭代计算模型有较好的支持。
3. 使用Hadoop和Spark进行数据处理
我采用了两个较基础的数据处理算法:
(1) WordCount算法:统计给定文本中每个单词出现的次数。这是大数据处理领域中最常用的算法之一,也是Hadoop的HelloWorld。
(2) 温度计算算法:使用气象站收集的数据计算出每个月的平均气温。
通过这两个算法,我掌握了Hadoop和Spark的数据处理流程、API接口和调试方法。数据处理过程中我的主要工作是对Hadoop和Spark处理结果的正确性和可读性进行验证和评估。
4. 分析比较Hadoop和Spark的优缺点
(1) 简便性方面:Hadoop的安装配置过程较为繁琐,安装配置过程中遇到的问题也比较多,需要仔细阅读文档才能进行操作。相比之下,Spark的安装配置过程较为简单,同时也成为了大数据处理工具中的一种主流工具之一。
(2) 易用性方面:Hadoop的编写代码和编译打包需要耗费一定的时间,查错也比较困难,而Spark的交互式编程可以更直观便捷地进行数据操作。
(3) 性能方面:Spark的RDD(弹性分布式数据集)是一个高速缓存,可以将数据缓存到内存中,从而快速响应数据操作请求。而Hadoop的分布式计算则需要中间文件,处理较为频繁。因此在对大规模数据进行处理的时候,Spark具有更快的计算速度。
五、实验感想
本次实验,我对大数据处理这一领域有了更深入的了解。Hadoop和Spark都是本领域中非常重要的工具,在学习的过程中,我不仅提升了大数据处理能力,也加深了对分布式计算的理解,对未来的学习和工作都有一定的借鉴意义。
总之,本次实验内容丰富,操作繁琐,不过从中我也收获颇丰,掌握了Spark和Hadoop数据处理的基本操作和API接口,了解了Hadoop和Spark的优缺点。通过实验,我进一步加深了对专业知识的理解,同时也获得了实践经验,对以后的学习和研究具有重要意义。
相关推荐
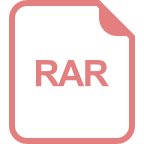
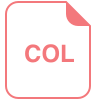
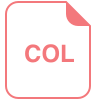
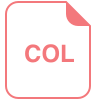
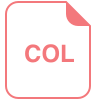
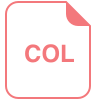







