用Unity中UnityWebRequest写一个读外部文件夹下最新txt并将数值存入变量中的程序
时间: 2024-05-01 18:16:59 浏览: 10
以下是使用UnityWebRequest来读取最新的txt文件并将数值存入变量中的示例程序:
```csharp
using System.Collections;
using UnityEngine;
using UnityEngine.Networking;
using System.IO;
public class ReadTextFile : MonoBehaviour
{
private string fileDirectory = "file:///C:/Users/YourUsername/Documents/TextFiles/"; //修改为你的txt文件所在的文件夹路径
private string latestFileName = "";
private float latestValue = 0;
void Start()
{
StartCoroutine(GetLatestFile());
}
IEnumerator GetLatestFile()
{
UnityWebRequest www = UnityWebRequest.Get(fileDirectory);
yield return www.SendWebRequest();
if (www.isNetworkError || www.isHttpError)
{
Debug.Log(www.error);
}
else
{
string[] fileNames = www.downloadHandler.text.Split('\n');
foreach (string fileName in fileNames)
{
if (fileName.EndsWith(".txt") && (latestFileName == "" || fileName.CompareTo(latestFileName) > 0))
{
latestFileName = fileName;
}
}
if (latestFileName != "")
{
UnityWebRequest fileRequest = UnityWebRequest.Get(fileDirectory + latestFileName);
yield return fileRequest.SendWebRequest();
if (fileRequest.isNetworkError || fileRequest.isHttpError)
{
Debug.Log(fileRequest.error);
}
else
{
string text = fileRequest.downloadHandler.text;
float.TryParse(text, out latestValue);
Debug.Log("Latest value: " + latestValue);
}
}
else
{
Debug.Log("No txt files found in directory.");
}
}
}
}
```
在上面的示例程序中,我们首先定义了一个字符串变量“fileDirectory”,用于存储txt文件所在的文件夹路径。然后我们定义了两个变量“latestFileName”和“latestValue”,用于存储最新的txt文件名和txt文件中的数值。
在Start方法中,我们调用了一个协程“GetLatestFile”。在这个协程中,我们使用UnityWebRequest来获取指定文件夹下的所有文件名。然后我们遍历所有文件,找到最新的txt文件。如果找到了最新的txt文件,我们再次使用UnityWebRequest来读取该文件的内容,并将其转换为float类型的数值,存储在“latestValue”变量中。
最后,我们在控制台中打印出“latestValue”变量的值,以便我们可以查看读取到的最新数值。
需要注意的是,我们需要将“fileDirectory”变量的值修改为我们实际的txt文件所在的文件夹路径。此外,我们还需要在Unity的“Player Settings”中开启“Internet Access”,以便我们可以使用UnityWebRequest来读取外部文件夹中的文件。
相关推荐
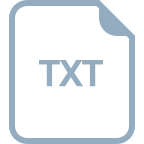
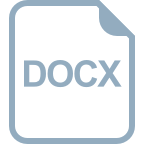














