写一个用Unity 2020中UnityWebRequest读取外部文件夹下最新txt并将数值数值存入变量来的程序
时间: 2024-06-12 20:05:40 浏览: 212
下面是一个示例程序,它使用UnityWebRequest读取外部文件夹下最新的txt文件,并将文件中的数值存储在变量中:
using System.Collections;
using UnityEngine;
using UnityEngine.Networking;
public class ReadLatestTxt : MonoBehaviour
{
// 外部文件夹的路径
public string folderPath;
// 存储最新文件中的数值
public float latestValue;
// Start方法会在场景启动时执行
void Start()
{
// 开始协程,等待读取最新的txt文件
StartCoroutine(ReadLatestTxtFile());
}
IEnumerator ReadLatestTxtFile()
{
// 获取文件夹中所有txt文件的路径
string[] fileNames = System.IO.Directory.GetFiles(folderPath, "*.txt");
// 如果没有txt文件,结束协程
if (fileNames.Length == 0)
{
Debug.LogError("No txt files found in folder " + folderPath);
yield break;
}
// 找到最新的txt文件
string latestFileName = "";
System.DateTime latestFileTime = System.DateTime.MinValue;
foreach (string fileName in fileNames)
{
System.DateTime fileTime = System.IO.File.GetLastWriteTime(fileName);
if (fileTime > latestFileTime)
{
latestFileTime = fileTime;
latestFileName = fileName;
}
}
// 使用UnityWebRequest读取最新的txt文件
UnityWebRequest www = UnityWebRequest.Get("file://" + latestFileName);
yield return www.SendWebRequest();
// 如果出错,结束协程
if (www.result != UnityWebRequest.Result.Success)
{
Debug.LogError("Error reading file " + latestFileName + ": " + www.error);
yield break;
}
// 从txt文件中读取数值
string text = www.downloadHandler.text;
if (float.TryParse(text, out latestValue))
{
Debug.Log("Latest value read from file " + latestFileName + ": " + latestValue);
}
else
{
Debug.LogError("Error parsing float from text " + text);
}
}
}
要使用此程序,请将其添加到场景中的任何游戏对象上,并将“folderPath”字段设置为外部文件夹的路径。当场景启动时,程序将在外部文件夹中查找所有txt文件,并找到最新的一个。然后,程序将使用UnityWebRequest读取此文件,并从文件中提取一个数值。最后,该数值将存储在“latestValue”变量中,供您在程序中使用。
阅读全文
相关推荐
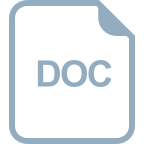
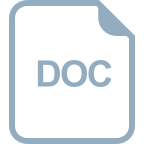
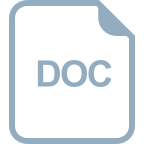







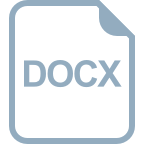
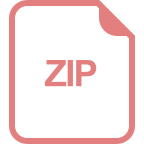
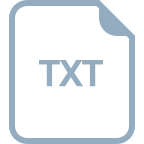
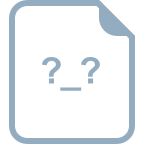
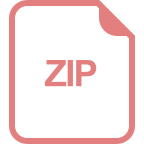
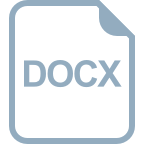
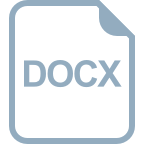
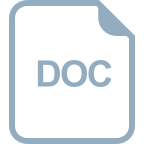