使用antd写一个表格嵌套表格
时间: 2023-11-14 08:21:35 浏览: 131
可以使用antd中的Table组件和ExpandableRow属性来实现表格嵌套表格的效果。
首先,需要定义一个父表格和一个子表格的columns和dataSource,然后在父表格中设置ExpandableRow属性来渲染子表格。
以下是示例代码:
```javascript
import React, { useState } from 'react';
import { Table } from 'antd';
const ParentTable = () => {
// 定义父表格数据
const [data, setData] = useState([
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York No. 1 Lake Park',
children: [
{
key: '11',
name: 'Jim Green',
age: 42,
address: 'London No. 1 Lake Park',
},
{
key: '12',
name: 'Joe Black',
age: 32,
address: 'Sidney No. 1 Lake Park',
},
],
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London No. 1 Lake Park',
children: [
{
key: '21',
name: 'Jim Green',
age: 42,
address: 'London No. 2 Lake Park',
},
{
key: '22',
name: 'Joe Black',
age: 32,
address: 'Sidney No. 2 Lake Park',
},
],
},
]);
// 定义父表格列
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
];
// 定义子表格列
const subColumns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
];
// 定义展开行方法
const expandable = {
expandedRowRender: (record) => {
return (
<Table
columns={subColumns}
dataSource={record.children}
pagination={false}
/>
);
},
rowExpandable: (record) => record.children.length > 0,
};
return (
<Table
columns={columns}
dataSource={data}
expandable={expandable}
pagination={false}
/>
);
};
export default ParentTable;
```
在以上示例代码中,我们首先定义了父表格的columns和dataSource,以及子表格的columns。然后定义了一个expandable对象,其中定义了展开行的渲染方法和展开条件。最后,在父表格中使用expandable属性来渲染子表格。
注意,为了使子表格的列与父表格的列对齐,需要在子表格的列中定义key值,并且保证与父表格列的key值一致。
阅读全文
相关推荐
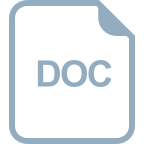
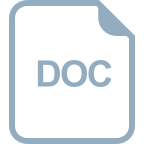
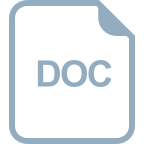






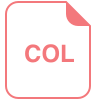
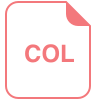







