Antd表格中的复杂数据操作示例
发布时间: 2024-02-23 04:56:56 阅读量: 44 订阅数: 25 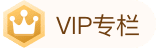
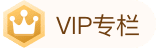
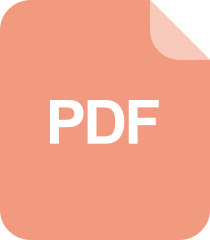
Antd的一些用法操作
# 1. Antd表格(Table)组件介绍
Ant Design(简称 Antd)是一个优秀的React UI组件库,提供了丰富的组件以及强大的定制能力,其中的表格(Table)组件是使用频率很高的一种组件。在本章中,我们将介绍Antd表格组件的基本用法、主要功能及特点,以及参数和配置项。
## 1.1 Antd表格组件的基本用法
Antd表格组件在React项目中的基本引入方式如下:
```jsx
import { Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 30, address: 'New York' },
{ key: '2', name: 'Jane Smith', age: 25, address: 'London' },
];
const columns = [
{ title: 'Name', dataIndex: 'name', key: 'name' },
{ title: 'Age', dataIndex: 'age', key: 'age' },
{ title: 'Address', dataIndex: 'address', key: 'address' },
];
const BasicTable = () => (
<Table dataSource={dataSource} columns={columns} />
);
export default BasicTable;
```
## 1.2 Antd表格组件的主要功能及特点
Antd表格组件支持的功能包括但不限于:分页、排序、筛选、编辑,同时具备响应式设计及自定义样式等特点。
## 1.3 Antd表格组件的参数和配置项
Antd表格组件提供了丰富的参数和配置项,例如`columns`、`dataSource`、`pagination`等,开发者可以根据需要进行灵活配置。
在本章中,我们介绍了Antd表格组件的基本用法、主要功能及特点,以及参数和配置项。接下来,我们将深入探讨Antd表格中的数据渲染。
# 2. Antd表格中的数据渲染
在Antd表格中,数据的渲染是非常重要的一部分,因为它直接影响到表格展示的内容和样式。本章将介绍在Antd表格中如何渲染简单数据、复杂数据,并进行自定义渲染列的操作。
### 2.1 在Antd表格中渲染简单数据
在Antd表格中,可以直接将简单的数据数组传入`dataSource`属性中进行渲染,例如:
```jsx
import { Table } from 'antd';
const dataSource = [
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York No. 1 Lake Park',
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London No. 1 Lake Park',
},
// more data...
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
// more columns...
];
const SimpleTable = () => <Table dataSource={dataSource} columns={columns} />;
```
在上面的示例中,`dataSource`数组包含了简单的用户数据,而`columns`数组定义了表格的列信息,通过`Table`组件的`dataSource`和`columns`属性,我们可以轻松地将简单数据渲染到Antd表格中。
### 2.2 在Antd表格中渲染复杂数据
有时候,我们需要在Antd表格中渲染复杂的数据结构,比如嵌套的对象或数组。这时,可以借助`render`函数来对数据进行处理和渲染,例如:
```jsx
const complexDataSource = [
{
key: '1',
name: { first: 'John', last: 'Brown' },
age: 32,
hobbies: ['Reading', 'Traveling'],
},
// more data...
];
const complexColumns = [
{
title: 'Name',
key: 'name',
render: (text, record) => (
<span>{`${record.name.first} ${record.name.last}`}</span>
),
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Hobbies',
key: 'hobbies',
render: (text, record) => (
<ul>
{record.hobbies.map((hobby, index) => (
<li key={index}>{hobby}</li>
))}
</ul>
),
},
// more columns...
];
const ComplexTable = () => <Table dataSource={complexDataSource} columns={complexColumns} />;
```
在上面的示例中,`complexDataSource`中的`name`字段是一个对象,`hobbies`字段是一个数组,通过在`render`函数中自定义处理,我们可以将复杂数据渲染到Antd表格中。
### 2.3 自定义渲染列
除了使用`render`函数进行数据处理和渲染外,还可以通过自定义组件来实现对列的渲染,例如:
```jsx
const customColumns = [
{
title: 'Avatar',
key: 'avatar',
render: () => <Avatar src="https://example.com/avatar.jpg" />,
},
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
// more columns...
];
```
在上面的示例中,我们可以使用`render`函数返回一个`Avatar`组件来渲染头像列,实现对列的自定义渲染。
以上就是在Antd表格中进行数据渲染的操作方法,可以根据实际需求,灵活运用这些方法来展示各种类型的数据。
# 3. Antd表格中的数据筛选与排序
在本章中,我们将介绍在Antd表格中如何进行数据筛选与排序的操作,这些功能对于提升表格的用户体验和数据展示效果非常重要。
#### 3.1 Antd表格中数据的筛选功能
在Antd表格中,可以通过设置`filterDropdown`和`onFilter`属性来实现筛选功能。`filterDropdown`用于自定义筛选框,`onFilter`用于自定义筛选逻辑。
```jsx
const columns = [
{
title: '姓名',
dataIndex: 'name',
key: 'name',
filterDropdown: ({ setSelectedKeys, selectedKeys, confirm, clearFilters }) => (
<div style={{ padding: 8 }}>
<Input
placeholder="搜索姓名"
value={selectedKeys[0]}
onChange={e => setSelectedKeys(e.target.value ? [e.target.value] : [])}
onPressEnter={() => confirm()}
style={{ width: 188, marginBottom: 8, display: 'block' }}
/>
<Button
type="primary"
onClick={() => confirm()}
icon={<SearchOutlined />}
size="small"
style={{ width: 90, marginRight: 8 }}
>
确定
</Button>
<Button onClick={() => clearFilters()} size="small" style={{ width: 90 }}>
重置
</Button>
</div>
),
onFilter: (value, record) => record.name.toLowerCase().includes(value.toLowerCase()),
},
// 更多列配置
];
```
#### 3.2 Antd表格中数据的排序功能
Antd表格可以轻松实现基于单列或多列的排序功能,只需设置`sorter`属性即可。`sorter`接受一个函数,用于自定义排序逻辑。
```jsx
const columns = [
{
title: '年龄',
dataIndex: 'age',
key: 'age',
sorter: (a, b) => a.age - b.age,
},
// 更多列配置
];
```
#### 3.3 自定义筛选与排序功能
通过Antd的`filterDropdown`和`onFilter`以及`sorter`属性,我们可以实现高度自定义的筛选与排序功能,满足各种复杂的数据展示需求。
```jsx
const columns = [
{
title: '金额',
dataIndex: 'amount',
key: 'amount',
sorter: (a, b) => a.amount - b.amount,
filterDropdown: ({ setSelectedKeys, selectedKeys, confirm, clearFilters }) => (
<div style={{ padding: 8 }}>
<Input
placeholder="搜索金额"
value={selectedKeys[0]}
onChange={e => setSelectedKeys(e.target.value
```
0
0
相关推荐
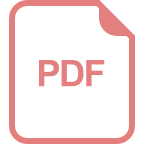
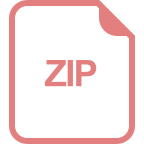
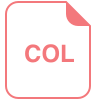
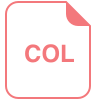
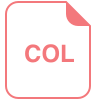
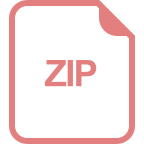
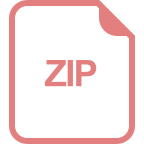
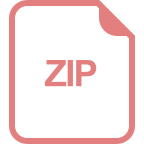