利用栈实现输入十进制得到十六进制
时间: 2023-06-02 13:03:58 浏览: 94
答案:可以通过以下步骤实现:
1. 将十进制转化为二进制
2. 将二进制按照4位一组进行分组
3. 将每一组二进制转化为对应的十六进制数
4. 将每个十六进制数压入栈中
5. 依次弹出栈中的元素,得到最终的十六进制数。
相关问题
C语言利用栈实现十进制转换二进制、八进制、十六进制的代码
以下是C语言利用栈实现十进制转换二进制、八进制、十六进制的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int top;
int capacity;
char* array;
} Stack;
Stack* createStack(int capacity) {
Stack* stack = (Stack*)malloc(sizeof(Stack));
stack->top = -1;
stack->capacity = capacity;
stack->array = (char*)malloc(capacity * sizeof(char));
return stack;
}
int isFull(Stack* stack) {
return stack->top == stack->capacity - 1;
}
int isEmpty(Stack* stack) {
return stack->top == -1;
}
void push(Stack* stack, char item) {
if (isFull(stack)) {
printf("Stack is full\n");
}
else {
stack->array[++stack->top] = item;
}
}
char pop(Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty\n");
return 0;
}
else {
return stack->array[stack->top--];
}
}
char peek(Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty\n");
return 0;
}
else {
return stack->array[stack->top];
}
}
void convertDecimalToBinary(int decimal) {
Stack* stack = createStack(100);
while (decimal > 0) {
int rem = decimal % 2;
push(stack, rem + '0');
decimal /= 2;
}
printf("Binary: ");
while (!isEmpty(stack)) {
printf("%c", pop(stack));
}
printf("\n");
free(stack->array);
free(stack);
}
void convertDecimalToOctal(int decimal) {
Stack* stack = createStack(100);
while (decimal > 0) {
int rem = decimal % 8;
push(stack, rem + '0');
decimal /= 8;
}
printf("Octal: ");
while (!isEmpty(stack)) {
printf("%c", pop(stack));
}
printf("\n");
free(stack->array);
free(stack);
}
void convertDecimalToHexadecimal(int decimal) {
Stack* stack = createStack(100);
while (decimal > 0) {
int rem = decimal % 16;
if (rem < 10) {
push(stack, rem + '0');
}
else {
push(stack, rem - 10 + 'A');
}
decimal /= 16;
}
printf("Hexadecimal: ");
while (!isEmpty(stack)) {
printf("%c", pop(stack));
}
printf("\n");
free(stack->array);
free(stack);
}
int main() {
int decimal;
printf("Enter a decimal number: ");
scanf("%d", &decimal);
convertDecimalToBinary(decimal);
convertDecimalToOctal(decimal);
convertDecimalToHexadecimal(decimal);
return 0;
}
```
在这个程序中,我们使用了一个`Stack`结构体,用于存储转换后的数字。`createStack`函数用于创建一个栈,`isFull`函数用于检查栈是否已满,`isEmpty`函数用于检查栈是否为空,`push`函数用于向栈中添加元素,`pop`函数用于从栈中弹出元素,`peek`函数用于查看栈顶元素。`convertDecimalToBinary`函数、`convertDecimalToOctal`函数和`convertDecimalToHexadecimal`函数分别用于将十进制数字转换为二进制、八进制和十六进制数字,并将结果输出到控制台上。
在`main`函数中,我们首先获取用户输入的十进制数字,然后调用上述三个函数分别将其转换为二进制、八进制和十六进制数字。
c语言利用栈十进制转十六进制
以下是利用栈实现十进制转十六进制的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int top;
} Stack;
void InitStack(Stack *s) {
s->top = -1;
}
int IsEmpty(Stack *s) {
return s->top == -1;
}
int IsFull(Stack *s) {
return s->top == MAXSIZE - 1;
}
void Push(Stack *s, int x) {
if (IsFull(s)) {
printf("Stack is full\n");
exit(1);
}
s->data[++s->top] = x;
}
int Pop(Stack *s) {
if (IsEmpty(s)) {
printf("Stack is empty\n");
exit(1);
}
return s->data[s->top--];
}
void DecimalToHex(int n) {
Stack s;
InitStack(&s);
while (n) {
Push(&s, n % 16);
n /= 16;
}
while (!IsEmpty(&s)) {
int x = Pop(&s);
if (x < 10)
printf("%d", x);
else
printf("%c", x - 10 + 'A');
}
}
int main() {
int n;
printf("Enter a decimal number: ");
scanf("%d", &n);
printf("Hexadecimal equivalent: ");
DecimalToHex(n);
return 0;
}
```
该程序使用栈来实现十进制转十六进制。首先将输入的十进制数每次除以16并将余数压入栈中,直到商为0。然后依次弹出栈中的元素并输出即可。注意,当弹出的元素大于等于10时,需要将其转换为对应的十六进制字符(A-F)。
阅读全文
相关推荐
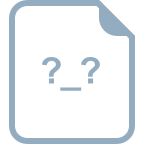
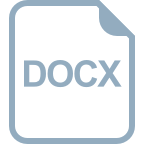





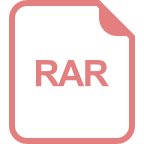






