怎么通过python连接数据库,拉取数据库内数据
时间: 2024-05-12 07:20:38 浏览: 107
要通过Python连接数据库,需要使用相应的数据库驱动程序。以下是连接常见数据库的基本示例:
1. MySQL
使用Python的MySQL驱动程序 `mysql-connector-python` 来连接MySQL数据库。首先需要安装驱动程序,可以使用 `pip` 命令安装:
```
pip install mysql-connector-python
```
连接MySQL数据库的示例代码如下:
```python
import mysql.connector
# 连接数据库
cnx = mysql.connector.connect(user='root', password='password', host='localhost', database='mydatabase')
# 查询数据
cursor = cnx.cursor()
query = ("SELECT id, name, age FROM mytable")
cursor.execute(query)
# 处理数据
for (id, name, age) in cursor:
print("{} - {} - {}".format(id, name, age))
# 关闭连接
cursor.close()
cnx.close()
```
2. SQLite
使用Python的SQLite驱动程序 `sqlite3` 来连接SQLite数据库,这个驱动程序是Python标准库的一部分,不需要额外安装。连接SQLite数据库的示例代码如下:
```python
import sqlite3
# 连接数据库
conn = sqlite3.connect('example.db')
# 查询数据
cursor = conn.cursor()
query = ("SELECT id, name, age FROM mytable")
cursor.execute(query)
# 处理数据
for (id, name, age) in cursor:
print("{} - {} - {}".format(id, name, age))
# 关闭连接
cursor.close()
conn.close()
```
3. PostgreSQL
使用Python的PostgreSQL驱动程序 `psycopg2` 来连接PostgreSQL数据库。首先需要安装驱动程序,可以使用 `pip` 命令安装:
```
pip install psycopg2
```
连接PostgreSQL数据库的示例代码如下:
```python
import psycopg2
# 连接数据库
conn = psycopg2.connect(database="mydatabase", user="myusername", password="mypassword", host="localhost", port="5432")
# 查询数据
cursor = conn.cursor()
query = ("SELECT id, name, age FROM mytable")
cursor.execute(query)
# 处理数据
for (id, name, age) in cursor:
print("{} - {} - {}".format(id, name, age))
# 关闭连接
cursor.close()
conn.close()
```
以上示例代码只是演示如何连接数据库和查询数据,实际的查询语句和操作方式需要根据具体的数据库和表结构进行调整。
阅读全文
相关推荐
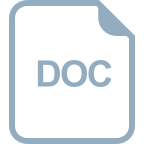
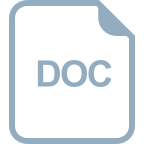
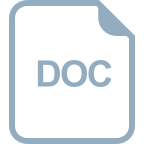
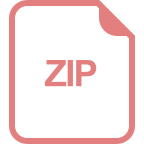
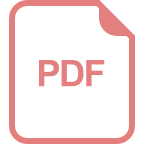
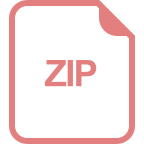
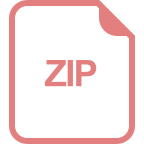
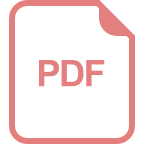
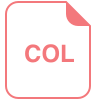
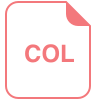
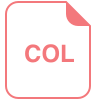
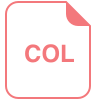
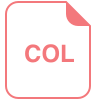





