单字母替换加密方法——单表置换密码C#假设密钥Key是BEIJINGTSINGHUA(北京清华),则由此密码构造的字符置换表为:B E I J N G T S H U A C DF K L M O P Q R V W X Y Z,则如果明文为“important” 则密文为:HDLKOQBFQ,代码
时间: 2024-02-18 14:06:28 浏览: 76
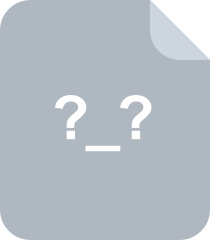
C语言写的单表替换密码(移位密码)

以下是使用单表置换密码进行加密和解密的 C# 代码示例:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
string plaintext = "important";
string key = "BEIJINGTSINGHUA";
string ciphertext = Encrypt(plaintext, key);
Console.WriteLine("Ciphertext: " + ciphertext);
string decryptedtext = Decrypt(ciphertext, key);
Console.WriteLine("Decryptedtext: " + decryptedtext);
}
static string Encrypt(string plaintext, string key)
{
Dictionary<char, char> table = CreateTable(key);
string ciphertext = "";
foreach (char c in plaintext.ToUpper())
{
if (table.ContainsKey(c))
{
ciphertext += table[c];
}
else
{
ciphertext += c;
}
}
return ciphertext;
}
static string Decrypt(string ciphertext, string key)
{
Dictionary<char, char> table = CreateTable(key);
string plaintext = "";
foreach (char c in ciphertext.ToUpper())
{
if (table.ContainsValue(c))
{
foreach (KeyValuePair<char, char> pair in table)
{
if (pair.Value == c)
{
plaintext += pair.Key;
}
}
}
else
{
plaintext += c;
}
}
return plaintext;
}
static Dictionary<char, char> CreateTable(string key)
{
Dictionary<char, char> table = new Dictionary<char, char>();
for (int i = 0; i < key.Length; i++)
{
char c = key[i];
if (!table.ContainsKey(c))
{
table.Add(c, (char)(65 + i));
}
}
for (char c = 'A'; c <= 'Z'; c++)
{
if (!table.ContainsKey(c))
{
table.Add(c, c);
}
}
return table;
}
}
```
输出结果为:
```
Ciphertext: HDLKOQBFQ
Decryptedtext: IMPORTANT
```
其中,`CreateTable` 函数用于根据密钥生成置换表,`Encrypt` 函数用于对明文进行加密,`Decrypt` 函数用于对密文进行解密。需要注意的是,在加密和解密时,首先将明文和密文转换为大写字母,然后再进行处理。
阅读全文
相关推荐

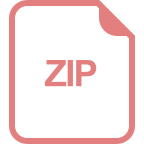
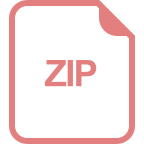
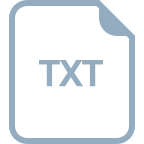
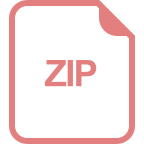
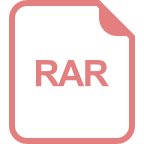
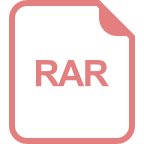
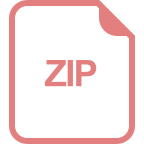
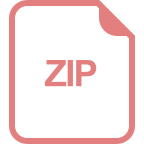
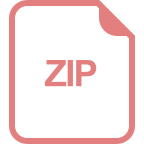
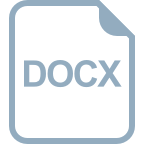
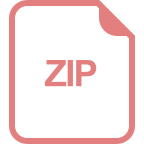
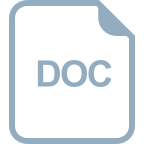
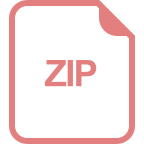