PSO-RFR的python
时间: 2023-10-14 17:27:36 浏览: 49
下面是一个简单的 PSO-RFR Python 代码示例:
首先需要安装依赖库:numpy, pandas, scikit-learn, pyswarms
```python
import numpy as np
import pandas as pd
from sklearn.datasets import load_boston
from sklearn.metrics import mean_squared_error, r2_score
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestRegressor
from pyswarms.single.global_best import GlobalBestPSO
# 加载波士顿房价数据集
boston = load_boston()
X = boston.data
y = boston.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义随机森林回归器
def rf_reg(params, X_train, y_train):
n_estimators = params[0]
max_depth = params[1]
min_samples_split = params[2]
min_samples_leaf = params[3]
model = RandomForestRegressor(n_estimators=int(n_estimators),
max_depth=int(max_depth),
min_samples_split=int(min_samples_split),
min_samples_leaf=int(min_samples_leaf),
random_state=42)
model.fit(X_train, y_train)
y_pred = model.predict(X_train)
# 计算MSE和R2分数
mse = mean_squared_error(y_train, y_pred)
r2 = r2_score(y_train, y_pred)
# 返回MSE作为适应度值
return mse
# 设置参数边界
bounds = [(10, 100), # n_estimators
(1, 50), # max_depth
(2, 20), # min_samples_split
(1, 20)] # min_samples_leaf
# 设置PSO算法参数
options = {'c1': 0.5, 'c2': 0.3, 'w': 0.9}
# 初始化PSO算法
optimizer = GlobalBestPSO(n_particles=10, dimensions=4, bounds=bounds, options=options)
# 运行PSO算法
best_cost, best_params = optimizer.optimize(rf_reg, 1000, X_train=X_train, y_train=y_train)
# 将最佳参数应用于随机森林回归器并进行预测
rf = RandomForestRegressor(n_estimators=int(best_params[0]),
max_depth=int(best_params[1]),
min_samples_split=int(best_params[2]),
min_samples_leaf=int(best_params[3]),
random_state=42)
rf.fit(X_train, y_train)
y_pred = rf.predict(X_test)
# 计算MSE和R2分数
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
# 输出测试集上的MSE和R2分数
print("MSE: ", mse)
print("R2: ", r2)
```
此代码使用了 pyswarms 库中的 GlobalBestPSO 类来执行 PSO 算法,使用 sklearn 库中的 RandomForestRegressor 类作为回归器来拟合波士顿房价数据集。
相关推荐
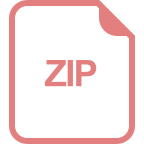













