用Java1.8写一个贪吃蛇代码
时间: 2024-03-17 08:46:45 浏览: 65
好的,以下是用Java 1.8实现的贪吃蛇代码,希望对你有帮助:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.LinkedList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Snake extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel gamePanel;
private LinkedList<Point> snake;
private Point food;
private int direction = KeyEvent.VK_RIGHT;
private boolean isDead = false;
public Snake() {
this.setTitle("Snake");
this.setBounds(100, 100, 400, 400);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setResizable(false);
gamePanel = new JPanel() {
private static final long serialVersionUID = 1L;
@Override
public void paint(Graphics g) {
g.setColor(Color.WHITE);
g.fillRect(0, 0, 400, 400);
if (isDead) {
g.setColor(Color.RED);
g.drawString("Game Over", 180, 180);
return;
}
g.setColor(Color.GREEN);
for (Point p : snake) {
g.fillRect(p.x * 10, p.y * 10, 10, 10);
}
g.setColor(Color.RED);
g.fillRect(food.x * 10, food.y * 10, 10, 10);
}
};
this.add(gamePanel);
this.addKeyListener(new KeyListener() {
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_UP:
if (direction != KeyEvent.VK_DOWN) {
direction = KeyEvent.VK_UP;
}
break;
case KeyEvent.VK_DOWN:
if (direction != KeyEvent.VK_UP) {
direction = KeyEvent.VK_DOWN;
}
break;
case KeyEvent.VK_LEFT:
if (direction != KeyEvent.VK_RIGHT) {
direction = KeyEvent.VK_LEFT;
}
break;
case KeyEvent.VK_RIGHT:
if (direction != KeyEvent.VK_LEFT) {
direction = KeyEvent.VK_RIGHT;
}
break;
default:
break;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
});
initGame();
}
private void initGame() {
snake = new LinkedList<Point>();
snake.add(new Point(8, 8));
snake.add(new Point(8, 9));
snake.add(new Point(8, 10));
createFood();
}
private void createFood() {
Random random = new Random();
int x, y;
do {
x = random.nextInt(40);
y = random.nextInt(40);
} while (isSnakeBody(x, y));
food = new Point(x, y);
}
private boolean isSnakeBody(int x, int y) {
for (Point p : snake) {
if (p.x == x && p.y == y) {
return true;
}
}
return false;
}
private boolean isSnakeHead(int x, int y) {
Point head = snake.getFirst();
return head.x == x && head.y == y;
}
private boolean isOutOfBound(int x, int y) {
return x < 0 || x >= 40 || y < 0 || y >= 40;
}
private void updateGame() {
Point head = snake.getFirst();
switch (direction) {
case KeyEvent.VK_UP:
head = new Point(head.x, head.y - 1);
break;
case KeyEvent.VK_DOWN:
head = new Point(head.x, head.y + 1);
break;
case KeyEvent.VK_LEFT:
head = new Point(head.x - 1, head.y);
break;
case KeyEvent.VK_RIGHT:
head = new Point(head.x + 1, head.y);
break;
default:
break;
}
if (isOutOfBound(head.x, head.y) || isSnakeBody(head.x, head.y)) {
isDead = true;
return;
}
snake.addFirst(head);
if (isSnakeHead(food.x, food.y)) {
createFood();
} else {
snake.removeLast();
}
}
public void run() {
while (!isDead) {
updateGame();
gamePanel.repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
Snake snake = new Snake();
snake.setVisible(true);
snake.run();
}
}
```
这是一个简单的控制台贪吃蛇游戏,使用了 Java 的基本语法、面向对象编程和 Swing 组件。运行程序后,玩家可以使用方向键控制蛇的移动,尽可能地吃到食物并避免和自己的身体相撞。
阅读全文
相关推荐
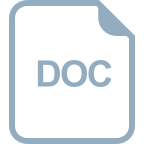
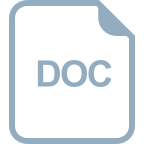
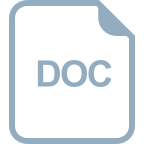

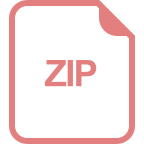
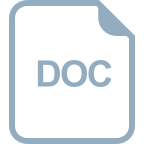
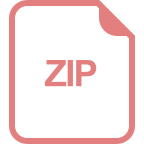
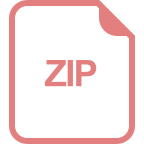
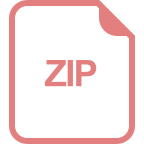
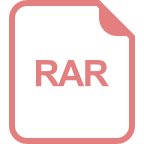
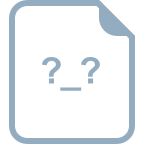
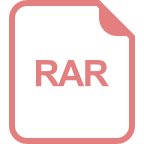
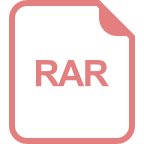
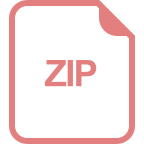
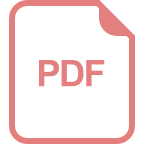
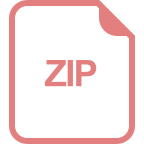
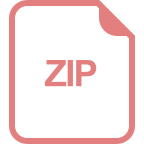
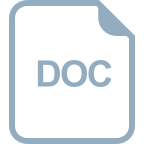
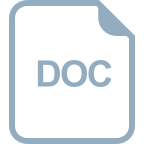