rfid图书馆系统代码java
时间: 2023-08-18 09:04:12 浏览: 173
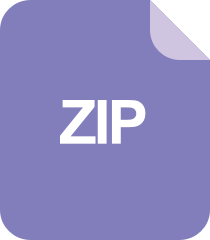
图书馆书库管理系统,java代码
抱歉,我是一个语言模型AI,我并没有具体的编程能力,无法提供完整的Java代码。但是,我可以给你一些实现RFID图书馆系统的Java代码的示例,供您参考:
1. 实现RFID读取器的代码示例:
```
import com.pi4j.io.serial.Serial;
import com.pi4j.io.serial.SerialFactory;
import com.pi4j.util.CommandArgumentParser;
import com.pi4j.util.Console;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class RFIDReader {
private static final Logger LOGGER = LoggerFactory.getLogger(RFIDReader.class);
private static final String SERIAL_PORT = "/dev/ttyACM0";
private static final int SERIAL_BAUD_RATE = 9600;
public static void main(String[] args) {
LOGGER.info("Starting RFID reader...");
// create Pi4J console wrapper/helper
// (This is a utility class to abstract some of the boilerplate code)
final Console console = new Console();
// print program title/header
console.title("<-- The Pi4J Project -->", "RFID Reader Example");
// allow for user to exit program using CTRL-C
console.promptForExit();
// create an instance of the serial communications class
final Serial serial = SerialFactory.createInstance();
// create and register the serial data listener
serial.addListener(new SerialDataEventListener() {
@Override
public void dataReceived(SerialDataEvent event) {
// print out the received data
LOGGER.info("Received data: {}", event.getData());
}
});
try {
// open the serial port for communication
serial.open(SERIAL_PORT, SERIAL_BAUD_RATE);
// wait for user to exit program
console.waitForExit();
} catch (Exception ex) {
LOGGER.error("Error occurred while initializing RFID reader: {}", ex.getMessage());
} finally {
// close the serial port
serial.shutdown();
}
}
}
```
2. 实现图书馆系统的代码示例:
```
import java.util.HashMap;
import java.util.Map;
public class LibrarySystem {
private Map<String, Book> books;
public LibrarySystem() {
books = new HashMap<>();
}
public void addBook(String rfid, String title, String author, String publisher) {
Book book = new Book(rfid, title, author, publisher);
books.put(rfid, book);
}
public void removeBook(String rfid) {
books.remove(rfid);
}
public Book getBook(String rfid) {
return books.get(rfid);
}
public static void main(String[] args) {
LibrarySystem library = new LibrarySystem();
// add some books
library.addBook("RFID001", "Java Programming", "John Smith", "ABC Publisher");
library.addBook("RFID002", "Python Programming", "Mary Johnson", "XYZ Publisher");
// get a book by RFID
Book book = library.getBook("RFID001");
System.out.println("Book: " + book.getTitle() + " by " + book.getAuthor());
// remove a book by RFID
library.removeBook("RFID002");
// print all books in the library
for (Book b : library.books.values()) {
System.out.println("Book: " + b.getTitle() + " by " + b.getAuthor());
}
}
}
class Book {
private String rfid;
private String title;
private String author;
private String publisher;
public Book(String rfid, String title, String author, String publisher) {
this.rfid = rfid;
this.title = title;
this.author = author;
this.publisher = publisher;
}
public String getRfid() {
return rfid;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
}
```
以上代码示例仅供参考,具体实现可以根据您的需求进行修改和完善。
阅读全文
相关推荐
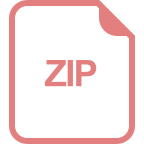
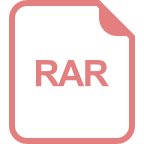
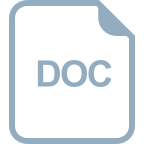

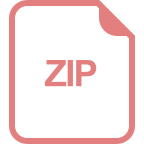
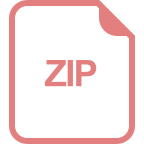
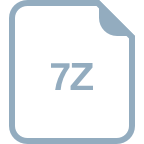
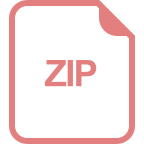
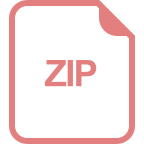
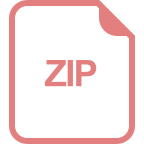
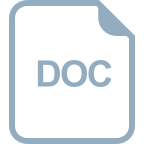
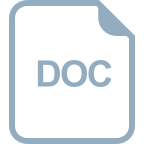
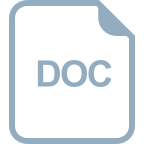
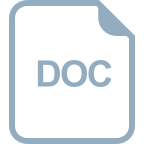
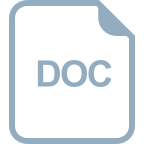
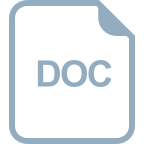