用vue2写一个当天时间轴前端代码,刻度条可以随着每分钟变化,并且有每天的固定小时时间显示
时间: 2024-05-10 08:16:20 浏览: 15
以下是一个简单的当天时间轴前端代码,使用Vue2来实现。主要功能是显示当天的时间轴,包括固定小时时间和随着每分钟变化的刻度条。
HTML代码:
```
<template>
<div class="time-axis">
<div class="fixed-hours">
<div v-for="hour in hours" class="hour">{{ hour }}:00</div>
</div>
<div class="scale">
<div v-for="minute in minutes" class="minute" :style="{ left: `${minute}px` }"></div>
<div class="current-time" :style="{ left: `${currentTime}px` }"></div>
</div>
</div>
</template>
```
CSS代码:
```
.time-axis {
position: relative;
height: 50px;
border-bottom: 1px solid #ccc;
}
.fixed-hours {
position: absolute;
top: 0;
bottom: 0;
left: 0;
width: 100%;
display: flex;
justify-content: space-between;
align-items: center;
}
.hour {
font-size: 14px;
}
.scale {
position: absolute;
top: 20px;
bottom: 0;
left: 0;
width: 100%;
overflow: hidden;
}
.minute {
position: absolute;
top: 0;
bottom: 0;
width: 1px;
background-color: #ccc;
}
.current-time {
position: absolute;
top: 0;
bottom: 0;
width: 1px;
background-color: red;
z-index: 1;
height: 100%;
}
```
JavaScript代码:
```
<script>
export default {
data() {
return {
hours: Array.from({ length: 24 }, (_, i) => i),
minutes: Array.from({ length: 1440 }, (_, i) => i),
currentTime: 0,
};
},
mounted() {
this.updateCurrentTime();
setInterval(this.updateCurrentTime, 60000);
},
methods: {
updateCurrentTime() {
const date = new Date();
const hour = date.getHours();
const minute = date.getMinutes();
this.currentTime = hour * 60 + minute;
},
},
};
</script>
```
该代码中,我们使用了Vue的数据绑定来动态生成固定小时时间和刻度条。在`mounted`生命周期钩子中,我们使用`setInterval`函数每分钟更新一次当前时间。同时,我们使用了CSS来定义样式,使时间轴看起来更美观。
相关推荐
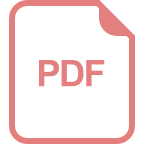
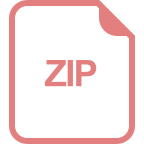














