C语言模拟一个简单的学生成绩管理系统,在D盘中存储一个初始密码,主函数开始就打开文件并读取密码存放在一个字符数组中,定义一个子函数实现输入密码的功能,用户最多可以输入三次密码,如果密码输入正确,该函数返回1,否则函数返回0。进入系统后,从键盘输入范围在1~7之间的选项序号,1可以修改密码并重新存入文件中,2表示列出所有学生记录,3表示列出总分最高的学生,4表示按姓名查找学生记录,5表示按学号查找学生记录,6表示按平均分降序排序,7表示退出系统。
时间: 2024-03-08 22:50:12 浏览: 131
好的,这是一个比较复杂的问题,需要一步一步来实现。首先,我们需要定义一个结构体来存储学生的信息,包括学号、姓名、各科成绩和总分。代码如下:
```c
#define MAX_NAME 20
#define MAX_STUDENT 100
struct student {
int sid; // 学号
char name[MAX_NAME]; // 姓名
float score[3]; // 三科成绩
float total; // 总分
};
struct student stu[MAX_STUDENT];
int num = 0; // 学生人数
```
接下来,我们需要实现一个函数来输入密码,并在用户输入错误密码时给出提示信息。代码如下:
```c
#include <stdio.h>
#include <string.h>
#define MAX_PASSWORD 20
#define MAX_TRY 3
void input_password(char* password) {
FILE* fp;
char password_file[] = "D:/password.txt";
char buffer[MAX_PASSWORD];
int i, try_times = 0;
fp = fopen(password_file, "r");
if (fp == NULL) {
printf("Failed to open password file.\n");
return;
}
fgets(buffer, MAX_PASSWORD, fp);
buffer[strlen(buffer) - 1] = '\0'; // 去掉换行符
fclose(fp);
while (try_times < MAX_TRY) {
printf("Please input password: ");
scanf("%s", password);
if (strcmp(password, buffer) == 0) {
printf("Password correct.\n");
return;
} else {
printf("Password incorrect. Please try again.\n");
try_times++;
}
}
printf("You have tried too many times. Exiting program.\n");
exit(1);
}
```
这个函数会从文件中读取密码,并在用户输入错误密码时给出提示信息。如果用户尝试次数超过了三次,程序会退出。
接下来,我们需要实现一个函数来修改密码。代码如下:
```c
void modify_password() {
FILE* fp;
char password_file[] = "D:/password.txt";
char password[MAX_PASSWORD];
printf("Please input new password: ");
scanf("%s", password);
fp = fopen(password_file, "w");
if (fp == NULL) {
printf("Failed to open password file.\n");
return;
}
fprintf(fp, "%s\n", password);
fclose(fp);
printf("Password has been modified.\n");
}
```
这个函数会让用户输入新密码,并将新密码写入文件中。
接下来,我们需要实现一个函数来列出所有学生记录。代码如下:
```c
void list_all_students() {
int i;
printf("sid\tname\tscore1\tscore2\tscore3\ttotal\n");
for (i = 0; i < num; i++) {
printf("%d\t%s\t%.1f\t%.1f\t%.1f\t%.1f\n", stu[i].sid, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
}
}
```
这个函数会逐个输出所有学生的信息。
接下来,我们需要实现一个函数来列出总分最高的学生。代码如下:
```c
void list_highest_score() {
int i, highest = 0;
for (i = 1; i < num; i++) {
if (stu[i].total > stu[highest].total) {
highest = i;
}
}
printf("The student with highest score is: %s, with a total score of %.1f.\n", stu[highest].name, stu[highest].total);
}
```
这个函数会找出总分最高的学生并输出其信息。
接下来,我们需要实现一个函数来按姓名查找学生记录。代码如下:
```c
void search_by_name() {
char name[MAX_NAME];
int i, found = 0;
printf("Please input the name of the student: ");
scanf("%s", name);
for (i = 0; i < num; i++) {
if (strcmp(stu[i].name, name) == 0) {
printf("sid\tname\tscore1\tscore2\tscore3\ttotal\n");
printf("%d\t%s\t%.1f\t%.1f\t%.1f\t%.1f\n", stu[i].sid, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
found = 1;
}
}
if (!found) {
printf("No student with name %s found.\n", name);
}
}
```
这个函数会让用户输入要查找的学生姓名,并输出该学生的信息。
接下来,我们需要实现一个函数来按学号查找学生记录。代码如下:
```c
void search_by_sid() {
int sid, i, found = 0;
printf("Please input the sid of the student: ");
scanf("%d", &sid);
for (i = 0; i < num; i++) {
if (stu[i].sid == sid) {
printf("sid\tname\tscore1\tscore2\tscore3\ttotal\n");
printf("%d\t%s\t%.1f\t%.1f\t%.1f\t%.1f\n", stu[i].sid, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
found = 1;
}
}
if (!found) {
printf("No student with sid %d found.\n", sid);
}
}
```
这个函数会让用户输入要查找的学生学号,并输出该学生的信息。
接下来,我们需要实现一个函数来按平均分降序排序。代码如下:
```c
void sort_by_average_score() {
int i, j;
struct student temp;
for (i = 0; i < num; i++) {
for (j = i + 1; j < num; j++) {
if ((stu[i].score[0] + stu[i].score[1] + stu[i].score[2]) / 3 < (stu[j].score[0] + stu[j].score[1] + stu[j].score[2]) / 3) {
temp = stu[i];
stu[i] = stu[j];
stu[j] = temp;
}
}
}
printf("sid\tname\tscore1\tscore2\tscore3\ttotal\n");
for (i = 0; i < num; i++) {
printf("%d\t%s\t%.1f\t%.1f\t%.1f\t%.1f\n", stu[i].sid, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
}
}
```
这个函数会按平均分降序排序,并输出排序后的结果。
最后,我们需要在主函数中调用这些函数来实现完整的学生成绩管理系统。代码如下:
```c
int main() {
char password[MAX_PASSWORD];
int choice;
input_password(password);
while (1) {
printf("\nPlease choose an option:\n");
printf("1. Modify password\n");
printf("2. List all students\n");
printf("3. List student with highest score\n");
printf("4. Search by name\n");
printf("5. Search by sid\n");
printf("6. Sort by average score\n");
printf("7. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
modify_password();
break;
case 2:
list_all_students();
break;
case 3:
list_highest_score();
break;
case 4:
search_by_name();
break;
case 5:
search_by_sid();
break;
case 6:
sort_by_average_score();
break;
case 7:
printf("Exiting program.\n");
return 0;
default:
printf("Invalid choice. Please try again.\n");
break;
}
}
return 0;
}
```
这个程序会在用户输入正确密码后进入主循环,等待用户输入选项。根据用户的选择,程序会调用相应的函数来执行相应的操作。如果用户选择退出,程序会退出。
阅读全文
相关推荐
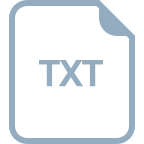
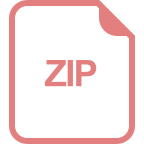
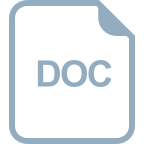
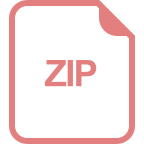
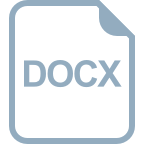
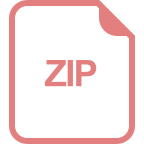
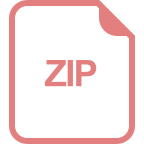
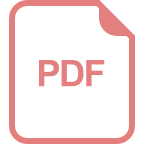