用c语言写一个学生成绩管理系统,用链表
时间: 2024-05-24 19:14:24 浏览: 17
来存储学生信息和成绩,实现增删改查功能。
好的,您需要一个学生成绩管理系统,使用链表来存储学生信息和成绩,实现增删改查功能。以下是一些步骤来实现这个系统:
1. 创建一个结构体,包括学生的姓名、学号、成绩等信息。
2. 创建一个链表节点结构体,包括指向下一个节点的指针和存储学生信息的结构体变量。
3. 实现添加学生信息的函数。这个函数应该将学生信息添加到链表中,以保证能够实现增删改查的功能。
4. 实现删除学生信息的函数。这个函数应该接收一个学生的学号,并从链表中删除对应的学生信息。
5. 实现修改学生信息的函数。这个函数应该接收一个学生的学号,并修改链表中对应学生的信息。
6. 实现查询学生信息的函数。这个函数应该接收一个学生的学号,并返回该学生的信息。如果找不到该学生,则返回相应的错误信息。
以上是用C语言实现一个学生成绩管理系统的一般步骤,你可以根据自己的具体需求进行修改和完善。希望对您有所帮助!
相关问题
用c语言使用链表写一个学生成绩管理系统
以下是一个简单的 C 语言实现的学生成绩管理系统,使用链表来存储学生信息和成绩:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 学生信息结构体
typedef struct Student {
char name[20]; // 姓名
int age; // 年龄
int score; // 成绩
struct Student* next; // 指向下一个学生的指针
} Student;
// 函数声明
Student* createStudent(char* name, int age, int score);
Student* addStudent(Student* head, Student* newStudent);
void printStudents(Student* head);
void freeStudents(Student* head);
int main(void) {
Student* head = NULL; // 学生链表头指针
// 添加学生
head = addStudent(head, createStudent("Tom", 18, 90));
head = addStudent(head, createStudent("Jerry", 19, 85));
head = addStudent(head, createStudent("Alice", 20, 95));
// 打印学生信息
printStudents(head);
// 释放链表内存
freeStudents(head);
return 0;
}
// 创建一个新的学生信息结构体
Student* createStudent(char* name, int age, int score) {
Student* newStudent = (Student*)malloc(sizeof(Student));
strcpy(newStudent->name, name);
newStudent->age = age;
newStudent->score = score;
newStudent->next = NULL;
return newStudent;
}
// 添加一个学生信息到链表中
Student* addStudent(Student* head, Student* newStudent) {
if (head == NULL) {
head = newStudent;
} else {
Student* p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = newStudent;
}
return head;
}
// 打印链表中的学生信息
void printStudents(Student* head) {
printf("姓名\t年龄\t成绩\n");
printf("=======================\n");
Student* p = head;
while (p != NULL) {
printf("%s\t%d\t%d\n", p->name, p->age, p->score);
p = p->next;
}
}
// 释放链表内存
void freeStudents(Student* head) {
Student* p = head;
while (p != NULL) {
Student* q = p;
p = p->next;
free(q);
}
}
```
这个程序中,我们定义了一个 `Student` 结构体,包含了学生的姓名、年龄、成绩和指向下一个学生的指针。我们使用 `createStudent` 函数来创建一个新的学生信息结构体,使用 `addStudent` 函数将新的学生信息加入到链表中。使用 `printStudents` 函数来打印链表中的学生信息,使用 `freeStudents` 函数来释放链表内存。在 `main` 函数中,我们先创建几个学生信息结构体,然后加入到链表中,最后打印学生信息并释放链表内存。
用c语言写一个成绩管理系统,要求用动态链表和注释
以下是用C语言实现的成绩管理系统,使用了动态链表和注释。
```
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
// 学生信息结构体
typedef struct student{
char name[20]; // 学生姓名
int id; // 学生学号
float score; // 学生成绩
struct student *next; // 指向下一个学生的指针
}Student;
// 创建新学生节点
Student* createStudent(char name[], int id, float score){
Student *newStudent = (Student*)malloc(sizeof(Student)); // 分配内存
strcpy(newStudent->name, name); // 复制姓名
newStudent->id = id; // 学号赋值
newStudent->score = score; // 成绩赋值
newStudent->next = NULL; // 指针初始化为NULL
return newStudent;
}
// 插入新学生节点到链表中
Student* insertStudent(Student *head, char name[], int id, float score){
Student *newStudent = createStudent(name, id, score); // 创建新学生
if(head == NULL){ // 如果链表为空
head = newStudent; // 新学生作为头结点
}else{ // 如果链表不为空
Student *current = head; // 从头开始遍历链表
while(current->next != NULL){ // 找到链表尾部
current = current->next;
}
current->next = newStudent; // 将新学生节点插入到链表尾部
}
return head; // 返回头结点
}
// 从链表中删除指定学生节点
Student* deleteStudent(Student* head, int id){
Student *current = head; // 从头开始遍历链表
Student *prev = NULL; // 当前节点的前驱节点
while(current != NULL){ // 遍历链表
if(current->id == id){ // 找到指定学号的学生
if(prev == NULL){ // 如果是头结点
head = current->next; // 将头结点指向下一个节点
}else{ // 如果不是头结点
prev->next = current->next; // 前驱节点指向当前节点的下一个节点
}
free(current); // 释放当前节点的内存
printf("删除成功!\n");
return head; // 返回头结点
}
prev = current; // 更新前驱节点
current = current->next; // 更新当前节点
}
printf("未找到该学生!\n");
return head; // 返回头结点
}
// 打印链表中所有学生的信息
void printList(Student *head){
printf("学号\t姓名\t成绩\n");
Student *current = head;
while(current != NULL){
printf("%d\t%s\t%.2f\n", current->id, current->name, current->score);
current = current->next;
}
}
int main(){
Student *head = NULL; // 头结点初始化为NULL
int choice = 0;
while(1){
printf("------菜单------\n");
printf("1. 添加学生信息\n");
printf("2. 删除学生信息\n");
printf("3. 显示所有学生信息\n");
printf("4. 退出\n");
printf("请选择操作:");
scanf("%d", &choice);
switch(choice){
case 1:{
char name[20];
int id;
float score;
printf("请输入学生姓名:");
scanf("%s", name);
printf("请输入学生学号:");
scanf("%d", &id);
printf("请输入学生成绩:");
scanf("%f", &score);
head = insertStudent(head, name, id, score); // 插入新学生节点
printf("添加成功!\n");
break;
}
case 2:{
int id;
printf("请输入要删除的学生学号:");
scanf("%d", &id);
head = deleteStudent(head, id); // 从链表中删除指定学生节点
break;
}
case 3:{
printList(head); // 打印链表中所有学生的信息
break;
}
case 4:{
return 0; // 退出程序
}
default:{
printf("输入有误,请重新输入!\n");
break;
}
}
}
return 0;
}
```
这个程序使用了动态链表来存储学生信息,通过 `createStudent()` 函数创建新的学生节点,通过 `insertStudent()` 函数将新节点插入到链表中,通过 `deleteStudent()` 函数从链表中删除指定节点,通过 `printList()` 函数打印链表中所有学生的信息。
在 `main()` 函数中,使用一个 `while` 循环来显示菜单,让用户选择操作。用户可以选择添加学生信息、删除学生信息、显示所有学生信息或退出程序。
程序中的注释可以帮助读者理解代码的功能和实现方式。
相关推荐
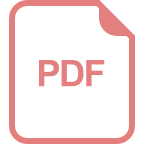
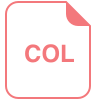
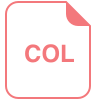
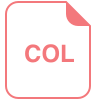
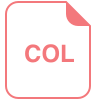
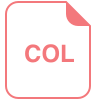







