c语言学生成绩管理系统用链表
时间: 2024-03-13 21:39:30 浏览: 109
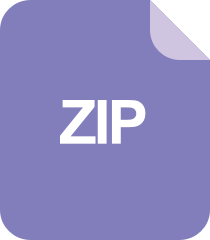
纯C语言实现学生成绩管理系统,采用链表结构

以下是一个用链表实现的C语言学生成绩管理系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct Student {
int id;
char name[50];
int score_chinese;
int score_math;
int score_english;
int score_politics;
int total_score;
struct Student* next;
} Student;
// 创建学生节点
Student* createStudent(int id, char name[], int score_chinese, int score_math, int score_english, int score_politics) {
Student* student = (Student*)malloc(sizeof(Student));
student->id = id;
strcpy(student->name, name);
student->score_chinese = score_chinese;
student->score_math = score_math;
student->score_english = score_english;
student->score_politics = score_politics;
student->total_score = score_chinese + score_math + score_english + score_politics;
student->next = NULL;
return student;
}
// 插入学生节点
void insertStudent(Student** head, Student* student) {
if (*head == NULL) {
*head = student;
} else {
Student* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = student;
}
}
// 打印学生信息
void printStudent(Student* student) {
printf("学号:%d\n", student->id);
printf("姓名:%s\n", student->name);
printf("语文成绩:%d\n", student->score_chinese);
printf("数学成绩:%d\n", student->score_math);
printf("英语成绩:%d\n", student->score_english);
printf("政治成绩:%d\n", student->score_politics);
printf("总分:%d\n", student->total_score);
printf("\n");
}
// 遍历打印学生链表
void printStudentList(Student* head) {
Student* current = head;
while (current != NULL) {
printStudent(current);
current = current->next;
}
}
int main() {
Student* head = NULL;
// 添加学生节点
Student* student1 = createStudent(1, "张三", 80, 90, 85, 75);
insertStudent(&head, student1);
Student* student2 = createStudent(2, "李四", 70, 85, 90, 80);
insertStudent(&head, student2);
Student* student3 = createStudent(3, "王五", 90, 75, 80, 85);
insertStudent(&head, student3);
// 打印学生链表
printStudentList(head);
return 0;
}
```
阅读全文
相关推荐
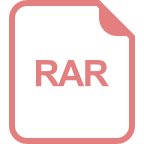

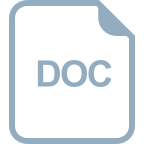

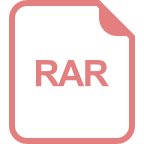
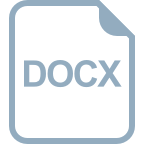
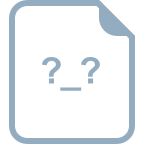
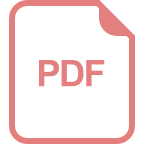
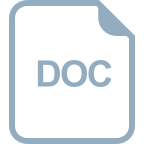
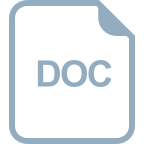
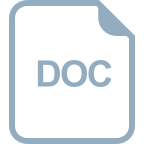
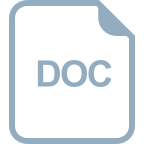
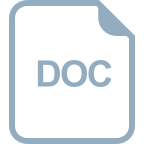

