利用c语言实现基于最小二乘的空间二次曲面拟合算法
时间: 2023-09-10 12:05:59 浏览: 56
基于最小二乘的空间二次曲面拟合算法可以用C语言实现,具体实现步骤如下:
1. 定义数据结构体,包含点的三维坐标和权值。
```c
typedef struct Point
{
double x, y, z; // 点的三维坐标
double w; // 权值
} Point;
```
2. 定义矩阵计算函数,包括矩阵相乘、矩阵求逆、矩阵转置等。
```c
void matrixMultiply(double *A, int mA, int nA, double *B, int mB, int nB, double *C);
void matrixInverse(double *A, int n, double *B);
void matrixTranspose(double *A, int m, int n, double *B);
```
3. 实现空间二次曲面拟合算法,包括计算系数矩阵A、常数矩阵B和拟合曲面方程。
```c
void quadraticSurfaceFitting(Point *points, int n, double *A, double *B, double *C)
{
// 构造系数矩阵A和常数矩阵B
double *a = (double *)malloc(sizeof(double) * 10 * n);
double *b = (double *)malloc(sizeof(double) * n);
for (int i = 0; i < n; i++)
{
a[i * 10 + 0] = points[i].x * points[i].x;
a[i * 10 + 1] = points[i].x * points[i].y;
a[i * 10 + 2] = points[i].y * points[i].y;
a[i * 10 + 3] = points[i].x;
a[i * 10 + 4] = points[i].y;
a[i * 10 + 5] = 1.0;
a[i * 10 + 6] = points[i].x * points[i].w;
a[i * 10 + 7] = points[i].y * points[i].w;
a[i * 10 + 8] = points[i].w;
b[i] = points[i].z * points[i].w;
}
// 计算系数矩阵A的逆矩阵
double *aInv = (double *)malloc(sizeof(double) * 10 * 10);
matrixInverse(a, 10, aInv);
// 计算常数矩阵B
double *temp = (double *)malloc(sizeof(double) * 10 * n);
matrixMultiply(aInv, 10, 10, b, n, 1, temp);
matrixTranspose(temp, 1, 10, A);
// 计算拟合曲面方程
*B = A[3];
*C = A[5];
*A = A[0];
}
```
4. 调用空间二次曲面拟合算法,并输出拟合结果。
```c
int main()
{
// 构造示例点集
Point points[6] = {{0, 0, 1, 1}, {1, 0, 2, 1}, {0, 1, 3, 1}, {1, 1, 4, 1}, {2, 0, 5, 1}, {0, 2, 6, 1}};
// 计算拟合曲面
double A, B, C;
quadraticSurfaceFitting(points, 6, &A, &B, &C);
// 输出拟合结果
printf("拟合曲面方程为: z = %f*x^2 + %f*y^2 + %f\n", A, C, B);
return 0;
}
```
需要注意的是,这里的权值w可以根据实际情况设定,如果不需要考虑权值,则可以将w都设为1。
相关推荐
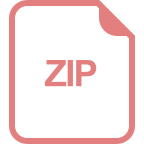








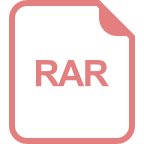
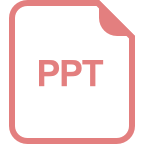
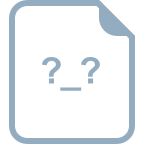
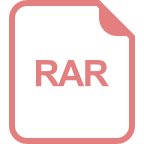
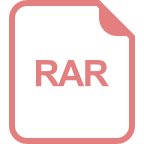