这是一段python代码,请根据这段代码基于python_opencv实现点击self.pushButton时打开已搜到的相机列表并实现鼠标点击选择打开相应相机并显示在self.label,当点击self.pushButton_2时抓取当时帧显示在self.label_2
时间: 2023-06-24 17:06:32 浏览: 55
请先安装Python的opencv库,然后使用以下代码实现:
```python
import cv2
from PyQt5.QtWidgets import QMainWindow, QApplication, QFileDialog
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtCore import QTimer
from ui_mainwindow import Ui_MainWindow
class MyMainWindow(QMainWindow, Ui_MainWindow):
def __init__(self, parent=None):
super(MyMainWindow, self).__init__(parent)
self.setupUi(self)
self.camera_list = []
self.current_camera = None
self.timer = QTimer(self)
self.timer.timeout.connect(self.show_camera)
def on_pushButton_clicked(self):
self.camera_list = []
for i in range(10):
cap = cv2.VideoCapture(i)
if cap.isOpened():
self.camera_list.append(i)
cap.release()
if len(self.camera_list) > 0:
camera_str = ''
for i in self.camera_list:
camera_str += 'Camera ' + str(i) + '\n'
camera_str = camera_str[:-1]
camera, ok = QFileDialog.getOpenFileName(self, 'Select Camera', camera_str)
if ok:
index = int(camera.split(' ')[1])
self.current_camera = cv2.VideoCapture(index)
self.timer.start(30)
def show_camera(self):
ret, frame = self.current_camera.read()
if ret:
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image = QImage(frame.data, frame.shape[1], frame.shape[0], QImage.Format_RGB888)
pixmap = QPixmap.fromImage(image)
self.label.setPixmap(pixmap)
def on_pushButton_2_clicked(self):
if self.current_camera is not None:
ret, frame = self.current_camera.read()
if ret:
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image = QImage(frame.data, frame.shape[1], frame.shape[0], QImage.Format_RGB888)
pixmap = QPixmap.fromImage(image)
self.label_2.setPixmap(pixmap)
if __name__ == '__main__':
import sys
app = QApplication(sys.argv)
mainWindow = MyMainWindow()
mainWindow.show()
sys.exit(app.exec_())
```
在这段代码中,我们创建了一个名为`MyMainWindow`的类,它继承自`QMainWindow`和`Ui_MainWindow`。`Ui_MainWindow`是使用Qt Designer创建的窗口,其中包含两个`QPushButton`和两个`QLabel`控件。我们在类的构造函数中初始化了一些变量,包括相机列表和当前相机。我们还创建了一个名为`timer`的Qtimer对象,该对象每隔30毫秒触发一次`show_camera`函数。
在`on_pushButton_clicked`函数中,我们首先查找所有可用的相机,并将它们添加到`camera_list`列表中。然后,我们使用`QFileDialog.getOpenFileName`方法打开一个对话框,该对话框显示可用相机的列表。如果用户选择了相机,则我们使用`cv2.VideoCapture`方法打开相机,并将其赋值给`current_camera`变量。最后,我们启动计时器以开始显示图像。
在`show_camera`函数中,我们使用`current_camera`捕获当前帧。如果捕获成功,则我们将图像转换为Qt支持的格式,并将其设置为`label`的Pixmap。
在`on_pushButton_2_clicked`函数中,我们检查`current_camera`是否为`None`。如果不是,则我们捕获当前帧,将其转换为Qt支持的格式,并将其设置为`label_2`的Pixmap。
相关推荐
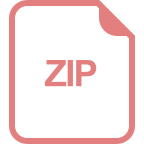









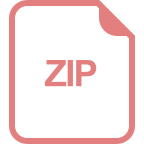
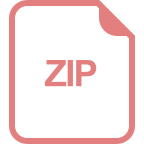
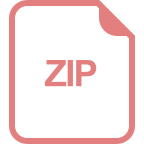
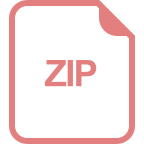
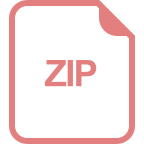