有一个untitled.ui文件,请写一个项目代码实现引用这个ui文件,基于opencv实现点击self.pushButton打开搜索到的相机列表,以鼠标点击选择相机打开再将视频显示在self.label,点击self.pushButton_2保存当前帧到当前文件目录并显示在self.label_2
时间: 2023-06-26 19:04:39 浏览: 56
以下是一个基于 PyQt5 和 OpenCV 的示例代码,可以实现你所描述的功能:
```python
import cv2
import os
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QFileDialog
from PyQt5.uic import loadUi
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
loadUi('untitled.ui', self) # 加载 UI 文件
self.camera_list = [] # 存储搜索到的相机列表
self.current_camera = None # 当前打开的相机
self.capture = None # 视频捕获对象
# 搜索相机列表
self.pushButton.clicked.connect(self.search_cameras)
# 打开相机并显示视频
self.label.mousePressEvent = self.select_camera
self.pushButton_2.clicked.connect(self.capture_frame)
def search_cameras(self):
# 使用 OpenCV 搜索相机列表
index = 0
while True:
cap = cv2.VideoCapture(index)
if not cap.isOpened():
break
self.camera_list.append(cap)
index += 1
self.label.setText('搜索到 %d 个相机' % len(self.camera_list))
def select_camera(self, event):
# 鼠标点击选择相机并打开
if not self.camera_list:
return
index = event.pos().y() // 20 # 计算点击的相机索引
if index >= len(self.camera_list):
return
self.current_camera = self.camera_list[index]
self.capture = cv2.VideoCapture(index)
self.label.setText('正在打开相机 %d' % index)
self.timer = self.label.startTimer(30)
def timerEvent(self, event):
# 定时刷新视频画面
if not self.capture:
return
ret, frame = self.capture.read()
if not ret:
return
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) # 转换颜色空间
h, w, ch = frame.shape
bytesPerLine = ch * w
convertToQtFormat = QImage(frame.data, w, h, bytesPerLine, QImage.Format_RGB888)
p = convertToQtFormat.scaled(self.label.width(), self.label.height())
self.label.setPixmap(QPixmap.fromImage(p))
def capture_frame(self):
# 保存当前帧到当前文件目录并显示在 self.label_2
if not self.capture:
return
ret, frame = self.capture.read()
if not ret:
return
filename, _ = QFileDialog.getSaveFileName(self, '保存', os.getcwd(), 'Image files (*.jpg *.png)')
if filename:
cv2.imwrite(filename, frame)
pixmap = QPixmap(filename).scaled(self.label_2.width(), self.label_2.height())
self.label_2.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
请注意,这只是一个简单的示例代码,可能需要根据你的具体需求进行修改。
相关推荐
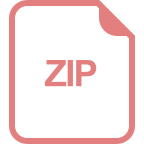














