shell脚本拷贝目录下除了某个文件的其他文件
时间: 2023-09-03 09:26:48 浏览: 179
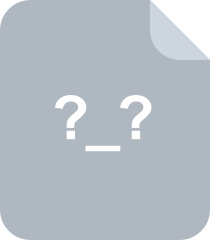
拷贝文件到另一个目录
### 回答1:
你可以用一个命令行工具 `rsync` 来实现。
使用 `rsync` 时,你需要在命令行中输入下面的格式:
```
rsync -av --exclude='文件名' 源目录 目标目录
```
其中,`-a` 表示保留文件属性,`-v` 表示在拷贝过程中显示详细信息,`--exclude` 表示要排除的文件。
你可以把它放到一个 shell 脚本中,然后执行脚本就可以了。
例如,你可以在终端中输入下面的命令来拷贝当前目录下的所有文件,但是排除名为 `file1` 的文件:
```
rsync -av --exclude='file1' . ../destination
```
如果你想要排除多个文件,可以使用多个 `--exclude` 参数,例如:
```
rsync -av --exclude='file1' --exclude='file2' . ../destination
```
希望这对你有帮助!
### 回答2:
可以使用以下的shell脚本来拷贝目录下除了某个文件的其他文件:
```shell
#!/bin/bash
# 指定源目录和目标目录
source_dir="/path/to/source"
target_dir="/path/to/target"
# 指定需要排除的文件名
exclude_file="exclude.txt"
# 检查目标目录是否存在,不存在则创建
if [ ! -d "$target_dir" ]; then
mkdir -p "$target_dir"
fi
# 遍历源目录下的文件和子目录
for item in "$source_dir"/*; do
# 排除exclude_file文件
if [ "$item" != "$source_dir/$exclude_file" ]; then
# 判断是否是文件
if [ -f "$item" ]; then
# 获取文件名
file_name=$(basename "$item")
# 拷贝文件到目标目录
cp "$item" "$target_dir/$file_name"
fi
fi
done
echo "拷贝完成!"
```
以上脚本中,首先要替换`source_dir`为源目录的路径,`target_dir`为目标目录的路径,`exclude_file`为需要排除的文件名。然后运行该脚本,它会遍历源目录下的所有文件和子目录,排除了`exclude_file`文件的拷贝其余文件到目标目录中。
### 回答3:
可以使用shell脚本来拷贝目录下除了某个文件的其他文件。以下是一个示例脚本:
```bash
#!/bin/bash
# 指定源目录和目标目录
source_dir="/path/to/source/directory"
target_dir="/path/to/target/directory"
# 指定要排除的文件名
exclude_file="exclude_file.txt"
# 遍历源目录下的文件和子目录
for file in "${source_dir}"/*; do
# 跳过排除的文件
if [[ "${file}" == *"${exclude_file}" ]]; then
continue
fi
# 如果是文件则进行拷贝
if [[ -f "${file}" ]]; then
cp "${file}" "${target_dir}"
fi
done
```
以上脚本指定了源目录和目标目录,并排除了名为 `exclude_file.txt` 的文件。使用 `for` 循环遍历源目录下的文件和子目录,通过判断文件名是否包含排除的文件名来跳过不需要拷贝的文件。然后,使用 `cp` 命令将文件拷贝到目标目录中。
注意,需要将 `source_dir` 和 `target_dir` 变量替换为实际的目录路径,并将 `exclude_file` 替换为要排除的文件名。另外,可能需要给脚本文件添加执行权限 (`chmod +x script.sh`),然后通过 `./script.sh` 来运行该脚本。
阅读全文
相关推荐
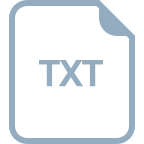
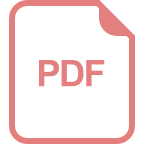










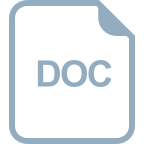



