编写程序实现静态方法copyImage(String img, String path),该方法的功能是将img图片拷贝到path目录下;其中img参数是包含图片名称及后缀名的绝对路径名称,path参数是本地已经存在的某个目录。
时间: 2024-03-11 14:49:28 浏览: 86
以下是 Java 代码实现:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ImageCopy {
public static void copyImage(String img, String path) throws IOException {
// 创建源文件和目标文件对象
File sourceFile = new File(img);
File targetFile = new File(path, sourceFile.getName());
// 创建输入流和输出流对象
FileInputStream inputStream = new FileInputStream(sourceFile);
FileOutputStream outputStream = new FileOutputStream(targetFile);
// 定义缓冲区,用于存储读取到的数据
byte[] buffer = new byte[1024];
int length;
// 将数据从输入流读取到缓冲区,并将缓冲区的数据写入输出流
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
// 关闭输入流和输出流
inputStream.close();
outputStream.close();
}
public static void main(String[] args) throws IOException {
String img = "C:\\Users\\User\\Desktop\\example.jpg"; // 图片的绝对路径名称
String path = "C:\\Users\\User\\Desktop"; // 目标目录的绝对路径名称
copyImage(img, path); // 调用静态方法实现拷贝
}
}
```
在该代码中,我们使用了 FileInputStream 和 FileOutputStream 类来分别创建输入流和输出流对象,然后通过缓冲区逐个字节地读取输入流中的数据,并将其写入输出流中。最后,我们需要调用 close() 方法关闭输入流和输出流。
阅读全文
相关推荐
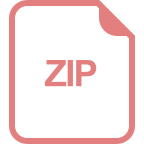
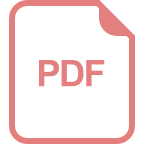
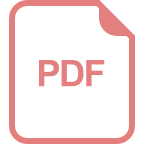















