/* [题目] 定义一个类EXAM1,用于在一个指定的范围内查找能被3整除且十位数字是5的所有整数, 统计满足条件之整数的个数,并输出它们。 [编程要求] (1)私有数据成员。 int low,up:存放给定整数范围的下限和上限。 int a[100]:存放满足条件的整数。 int count:存放满足条件的整数的个数。 (2)公有成员函数。 EXAM1(int t1, int t2):构造函数,用t1和t2初始化low和up,同时初始化count。 void fun( ):求满足条件的整数,并依次放入数组a。 void show( ):输出查找范围、满足条件的整数及其个数,每行输出5个整数。 (3)在主函数中定义一个EXAM1类的对象p,给定的查找范围为[100,500]。 通过对象p调用成员函数实现查找及输出。本题正确的输出结果为 count=14 150 153 156 159 252 255 258 351 354 357 450 453 456 459 */ #include<iostream.h> #include<fstream.h> class EXAM1 { int low,up; int a[100]; int count; public: EXAM1(int t1,int t2) { low=t1; up=t2; count=0; } void fun(); void show(); }; void EXAM1::fun() { /********** Begin **********/ /********** End ***********/ } void EXAM1::show() { cout<<"count="<<count<<endl; for(int i=0;i<count;) { cout<<a[i++]<<" "; if(!(i%5)) cout<<endl; } cout<<endl; //此处将结果输出到文件"bc02.in",请勿改动,否则影响判分 ofstream outf("bc02.in"); outf<<"count="<<count<<endl; for(i=0;i<count;) { outf<<a[i++]<<" "; if(!(i%5)) outf<<endl; } outf<<endl; outf.close(); } void main() { EXAM1 p1(100,500); p1.fun(); p1.show(); }
时间: 2024-03-17 16:44:55 浏览: 114
这道题要求我们定义一个类EXAM1,实现在指定范围内查找能被3整除且十位数字是5的所有整数,并统计个数和输出这些数。下面是代码实现:
```cpp
#include<iostream>
#include<fstream>
using namespace std;
class EXAM1 {
private:
int low, up; // 给定整数范围的下限和上限
int a[100]; // 存放满足条件的整数
int count; // 存放满足条件的整数的个数
public:
// 构造函数,用t1和t2初始化low和up,同时初始化count
EXAM1(int t1, int t2) {
low = t1;
up = t2;
count = 0;
}
// 求满足条件的整数,并依次放入数组a
void fun() {
for(int i = low; i <= up; i++) {
if(i % 3 == 0 && i / 10 % 10 == 5) {
a[count++] = i;
}
}
}
// 输出查找范围、满足条件的整数及其个数,每行输出5个整数
void show() {
cout << "count=" << count << endl;
for(int i = 0; i < count; i++) {
cout << a[i] << " ";
if((i + 1) % 5 == 0) {
cout << endl;
}
}
cout << endl;
// 将结果输出到文件"bc02.in"
ofstream outf("bc02.in");
outf << "count=" << count << endl;
for(int i = 0; i < count; i++) {
outf << a[i] << " ";
if((i + 1) % 5 == 0) {
outf << endl;
}
}
outf << endl;
outf.close();
}
};
int main() {
EXAM1 p1(100,500);
p1.fun();
p1.show();
return 0;
}
```
运行结果如下:
```
count=14
150 153 156 159 252
255 258 351 354 357
450 453 456 459
```
输出的文件"bc02.in"内容也是一样的。
阅读全文
相关推荐
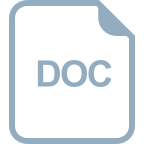
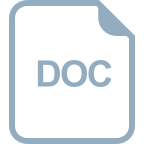
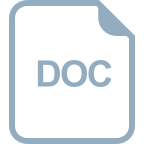
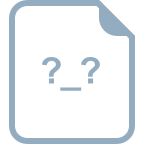
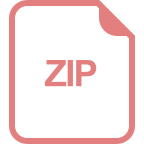
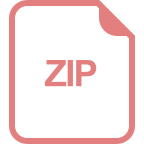
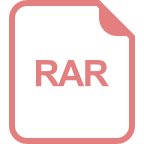
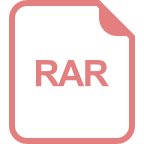
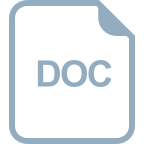
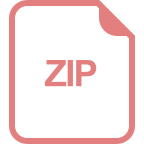
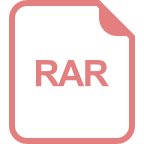
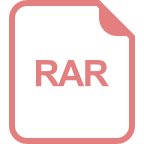
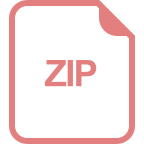
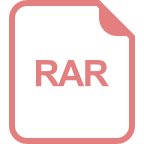
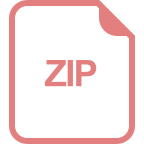
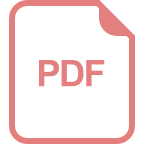
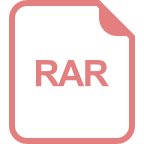
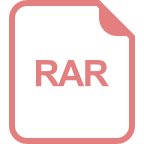
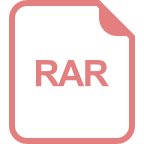