list_t* list_new(){list_t* list=NULL;list=(list_t*)malloc(sizeof(list_t));list->head=NULL;return(list);)}报错int()differs in levels of indirection from list_t*()
时间: 2023-10-06 16:11:43 浏览: 90
这个错误通常是因为在调用 `list_new()` 函数时,有一个不匹配的类型。可能是因为你的函数原型和调用不匹配,或者你在调用时没有正确的传递参数。
请确保你在调用 `list_new()` 函数时不需要传递任何参数,并且你在调用该函数时,使用了正确的语法,如下所示:
```
list_t* my_list = list_new();
```
如果你仍然遇到问题,请检查你的代码,确保在 `list_t` 结构体中没有定义任何错误,并且你在调用 `list_new()` 函数时,使用了正确的头文件和库。
相关问题
list_t* list_new(){list_t* list=NULL;list=(list_t*)malloc(sizeof(list_t));list->head=NULL;return(list);)}意思
这段代码定义了一个函数 `list_new()`,它返回一个指向 `list_t` 类型的指针。函数内部实现了以下操作:
1. 创建一个指向 `list_t` 结构体的指针 `list`,并将其初始化为 `NULL`。
2. 使用 `malloc()` 函数为 `list` 分配了一段内存,大小为 `sizeof(list_t)`。
3. 将 `list->head` 指向 `NULL`。
4. 返回指向 `list` 的指针。
这段代码的作用是创建一个新的链表对象,并返回指向该对象的指针。
请参考我给出的代码框架,实现对EMPLOYEE结构体为数据的双向链表的排序算法,要求按照按employeeId升序排列 typedef struct linkNode { void* data; //使用空指针使得NODE适配多种数据结构 struct linkNode* preNode; struct linkNode* nextNode; }LINKED_NODE; /*Define the struct of double linked list.*/ typedef struct { LINKED_NODE* head; LINKED_NODE* tail; size_t size; }DOUBLE_LINK_LIST; typedef struct { int employeeId; char name[20]; char ipAddress[30]; char seatNumber[20]; char group[10]; } EMPLOYEE; DOUBLE_LINK_LIST* createDoubleLinkedList() { DOUBLE_LINK_LIST* newList = (DOUBLE_LINK_LIST*)malloc(sizeof(DOUBLE_LINK_LIST)); newList->head = NULL; newList->tail = NULL; newList->size = 0; return newList; } void destroyDoubleLinkedList(DOUBLE_LINK_LIST* list) {} /*Add a new node before the head.*/ void insertHead(DOUBLE_LINK_LIST* list, void* data) // void执政适配其他data类型? {} /*Add a new node after tail.*/ void insertTail(DOUBLE_LINK_LIST* list, void* data) // 如何适配其他data类型? {} /*Insert a new node.*/ void insertNode(DOUBLE_LINK_LIST* list, void* data,int index) // 如何适配其他data类型? {} void deleteHead(DOUBLE_LINK_LIST* list) {} void deleteTail(DOUBLE_LINK_LIST* list) {} void deleteNode(DOUBLE_LINK_LIST* list, int index) {} LINKED_NODE* getNode(DOUBLE_LINK_LIST* list, int index) {} /* 遍历链表,对每个节点执行指定操作*/ void traverseList(DOUBLE_LINK_LIST* list, void (*callback)(void*)) { LINKED_NODE* currentNode = list->head; while (currentNode != NULL) { callback(currentNode->data); currentNode = currentNode->nextNode; } } void printEmployee(void* data) {}
你可以使用以下代码来实现对EMPLOYEE结构体为数据的双向链表的排序算法,按照按employeeId升序排列:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct linkNode
{
void* data; //使用空指针使得NODE适配多种数据结构
struct linkNode* preNode;
struct linkNode* nextNode;
} LINKED_NODE;
/*Define the struct of double linked list.*/
typedef struct
{
LINKED_NODE* head;
LINKED_NODE* tail;
size_t size;
} DOUBLE_LINK_LIST;
typedef struct
{
int employeeId;
char name[20];
char ipAddress[30];
char seatNumber[20];
char group[10];
} EMPLOYEE;
DOUBLE_LINK_LIST* createDoubleLinkedList()
{
DOUBLE_LINK_LIST* newList = (DOUBLE_LINK_LIST*)malloc(sizeof(DOUBLE_LINK_LIST));
newList->head = NULL;
newList->tail = NULL;
newList->size = 0;
return newList;
}
void destroyDoubleLinkedList(DOUBLE_LINK_LIST* list)
{
LINKED_NODE* currentNode = list->head;
while (currentNode != NULL)
{
LINKED_NODE* nextNode = currentNode->nextNode;
free(currentNode);
currentNode = nextNode;
}
free(list);
}
/*Add a new node before the head.*/
void insertHead(DOUBLE_LINK_LIST* list, void* data)
{
LINKED_NODE* newNode = (LINKED_NODE*)malloc(sizeof(LINKED_NODE));
newNode->data = data;
newNode->preNode = NULL;
newNode->nextNode = list->head;
if (list->head != NULL)
list->head->preNode = newNode;
list->head = newNode;
if (list->tail == NULL)
list->tail = newNode;
list->size++;
}
/*Add a new node after tail.*/
void insertTail(DOUBLE_LINK_LIST* list, void* data)
{
LINKED_NODE* newNode = (LINKED_NODE*)malloc(sizeof(LINKED_NODE));
newNode->data = data;
newNode->preNode = list->tail;
newNode->nextNode = NULL;
if (list->tail != NULL)
list->tail->nextNode = newNode;
list->tail = newNode;
if (list->head == NULL)
list->head = newNode;
list->size++;
}
/*Insert a new node.*/
void insertNode(DOUBLE_LINK_LIST* list, void* data, int index)
{
if (index < 0 || index > list->size)
{
printf("Invalid index\n");
return;
}
if (index == 0)
{
insertHead(list, data);
return;
}
if (index == list->size)
{
insertTail(list, data);
return;
}
LINKED_NODE* currentNode = getNode(list, index);
LINKED_NODE* newNode = (LINKED_NODE*)malloc(sizeof(LINKED_NODE));
newNode->data = data;
newNode->preNode = currentNode->preNode;
newNode->nextNode = currentNode;
currentNode->preNode->nextNode = newNode;
currentNode->preNode = newNode;
list->size++;
}
void deleteHead(DOUBLE_LINK_LIST* list)
{
if (list->head == NULL)
return;
LINKED_NODE* oldHead = list->head;
list->head = oldHead->nextNode;
if (list->head != NULL)
list->head->preNode = NULL;
free(oldHead);
list->size--;
if (list->size == 0)
list->tail = NULL;
}
void deleteTail(DOUBLE_LINK_LIST* list)
{
if (list->tail == NULL)
return;
LINKED_NODE* oldTail = list->tail;
list->tail = oldTail->preNode;
if (list->tail != NULL)
list->tail->nextNode = NULL;
free(oldTail);
list->size--;
if (list->size == 0)
list->head = NULL;
}
void deleteNode(DOUBLE_LINK_LIST* list, int index)
{
if (index < 0 || index >= list->size)
{
printf("Invalid index\n");
return;
}
if (index == 0)
{
deleteHead(list);
return;
}
if (index == list->size - 1)
{
deleteTail(list);
return;
}
LINKED_NODE* currentNode = getNode(list, index);
currentNode->preNode->nextNode = currentNode->nextNode;
currentNode->nextNode->preNode = currentNode->preNode;
free(currentNode);
list->size--;
}
LINKED_NODE* getNode(DOUBLE_LINK_LIST* list, int index)
{
if (index < 0 || index >= list->size)
{
printf("Invalid index\n");
return NULL;
}
LINKED_NODE* currentNode = list->head;
int i = 0;
while (i < index)
{
currentNode = currentNode->nextNode;
i++;
}
return currentNode;
}
void traverseList(DOUBLE_LINK_LIST* list, void (*callback)(void*))
{
LINKED_NODE* currentNode = list->head;
while (currentNode != NULL)
{
callback(currentNode->data);
currentNode = currentNode->nextNode;
}
}
void printEmployee(void* data)
{
EMPLOYEE* employee = (EMPLOYEE*)data;
printf("Employee ID: %d, Name: %s\n", employee->employeeId, employee->name);
}
int compareEmployee(const void* a, const void* b)
{
EMPLOYEE* employeeA = (EMPLOYEE*)a;
EMPLOYEE* employeeB = (EMPLOYEE*)b;
return employeeA->employeeId - employeeB->employeeId;
}
void sortList(DOUBLE_LINK_LIST* list)
{
size_t dataSize = sizeof(EMPLOYEE);
EMPLOYEE** employeeArray = (EMPLOYEE**)malloc(list->size * sizeof(EMPLOYEE*));
LINKED_NODE* currentNode = list->head;
size_t i = 0;
while (currentNode != NULL)
{
employeeArray[i] = (EMPLOYEE*)currentNode->data;
currentNode = currentNode->nextNode;
i++;
}
qsort(employeeArray, list->size, dataSize, compareEmployee);
currentNode = list->head;
i = 0;
while (currentNode != NULL)
{
currentNode->data = employeeArray[i];
currentNode = currentNode->nextNode;
i++;
}
free(employeeArray);
}
int main()
{
DOUBLE_LINK_LIST* list = createDoubleLinkedList();
EMPLOYEE* employee1 = (EMPLOYEE*)malloc(sizeof(EMPLOYEE));
employee1->employeeId = 2;
strcpy(employee1->name, "John");
insertHead(list, employee1);
EMPLOYEE* employee2 = (EMPLOYEE*)malloc(sizeof(EMPLOYEE));
employee2->employeeId = 1;
strcpy(employee2->name, "Alice");
insertHead(list, employee2);
EMPLOYEE* employee3 = (EMPLOYEE*)malloc(sizeof(EMPLOYEE));
employee3->employeeId = 3;
strcpy(employee3->name, "Bob");
insertHead(list, employee3);
printf("Before sorting:\n");
traverseList(list, printEmployee);
sortList(list);
printf("\nAfter sorting:\n");
traverseList(list, printEmployee);
destroyDoubleLinkedList(list);
return 0;
}
```
这段代码首先定义了双向链表的结构体和EMPLOYEE结构体,然后实现了双向链表的创建、销毁、插入、删除、遍历等操作。其中,`sortList`函数使用了快速排序算法对双向链表中的EMPLOYEE结构体按照employeeId升序进行排序。在`main`函数中,创建了一个双向链表并插入了三个EMPLOYEE结构体,然后调用`sortList`函数对链表进行排序并输出结果。
请注意,在代码中使用了动态内存分配(`malloc`)来分配内存,并在适当的时候使用了`free`来释放内存,以防止内存泄漏。
阅读全文
相关推荐
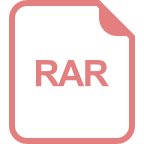
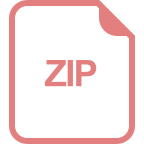
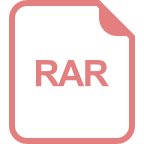













