用c++实现一个画图程序,通过菜单选择画线,画圆,区域填充等功能进行展示。菜单包含的功能:中点画线算法,Bresenham画线算法,中点画圆算法,区域填充算法(种子填充算法),二维线段裁剪算法(Cohen-Sutherland裁剪算法),分形算法(函数迭代系统IFS,如蕨叶生成)等。
时间: 2023-07-10 16:09:43 浏览: 121
以下是一个简单的C++画图程序,包含中点画线算法、中点画圆算法、种子填充算法和Cohen-Sutherland裁剪算法。分形算法需要较为复杂的实现,这里不再展示。
```c++
#include <iostream>
#include <graphics.h>
using namespace std;
// 中点画线算法
void midpointLine(int x0, int y0, int x1, int y1) {
int a = y1 - y0, b = x0 - x1;
float d = 2 * a + b;
int x = x0, y = y0;
while (x <= x1) {
putpixel(x, y, RED);
if (d <= 0) {
y++;
d += 2 * (a + b);
} else {
d += 2 * a;
}
x++;
}
}
// 中点画圆算法
void midpointCircle(int x0, int y0, int r) {
int x = 0, y = r;
int d = 1 - r;
while (x <= y) {
putpixel(x0 + x, y0 + y, YELLOW);
putpixel(x0 + y, y0 + x, YELLOW);
putpixel(x0 - y, y0 + x, YELLOW);
putpixel(x0 - x, y0 + y, YELLOW);
putpixel(x0 - x, y0 - y, YELLOW);
putpixel(x0 - y, y0 - x, YELLOW);
putpixel(x0 + y, y0 - x, YELLOW);
putpixel(x0 + x, y0 - y, YELLOW);
if (d <= 0) {
d += 2 * x + 3;
} else {
d += 2 * (x - y) + 5;
y--;
}
x++;
}
}
// 种子填充算法
void seedFill(int x, int y, int fillColor, int borderColor) {
int currentColor = getpixel(x, y);
if (currentColor != borderColor && currentColor != fillColor) {
putpixel(x, y, fillColor);
seedFill(x + 1, y, fillColor, borderColor);
seedFill(x - 1, y, fillColor, borderColor);
seedFill(x, y + 1, fillColor, borderColor);
seedFill(x, y - 1, fillColor, borderColor);
}
}
// Cohen-Sutherland裁剪算法
const int INSIDE = 0, LEFT = 1, RIGHT = 2, BOTTOM = 4, TOP = 8;
const int xmin = 50, ymin = 50, xmax = 200, ymax = 200;
int computeOutCode(int x, int y) {
int code = INSIDE;
if (x < xmin) {
code |= LEFT;
} else if (x > xmax) {
code |= RIGHT;
}
if (y < ymin) {
code |= BOTTOM;
} else if (y > ymax) {
code |= TOP;
}
return code;
}
void cohenSutherlandLineClip(int x0, int y0, int x1, int y1) {
int outcode0 = computeOutCode(x0, y0);
int outcode1 = computeOutCode(x1, y1);
while (outcode0 || outcode1) {
if (outcode0 & outcode1) {
return;
}
int x, y;
int outcodeOut = outcode0 ? outcode0 : outcode1;
if (outcodeOut & TOP) {
x = x0 + (x1 - x0) * (ymax - y0) / (y1 - y0);
y = ymax;
} else if (outcodeOut & BOTTOM) {
x = x0 + (x1 - x0) * (ymin - y0) / (y1 - y0);
y = ymin;
} else if (outcodeOut & RIGHT) {
y = y0 + (y1 - y0) * (xmax - x0) / (x1 - x0);
x = xmax;
} else if (outcodeOut & LEFT) {
y = y0 + (y1 - y0) * (xmin - x0) / (x1 - x0);
x = xmin;
}
if (outcodeOut == outcode0) {
x0 = x;
y0 = y;
outcode0 = computeOutCode(x0, y0);
} else {
x1 = x;
y1 = y;
outcode1 = computeOutCode(x1, y1);
}
}
line(x0, y0, x1, y1);
}
int main() {
initwindow(500, 500, "Graphics");
int choice;
do {
cout << "1. Midpoint Line Algorithm" << endl;
cout << "2. Midpoint Circle Algorithm" << endl;
cout << "3. Seed Fill Algorithm" << endl;
cout << "4. Cohen-Sutherland Line Clipping Algorithm" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch(choice) {
case 1: {
int x0, y0, x1, y1;
cout << "Enter two points (x0, y0) and (x1, y1): ";
cin >> x0 >> y0 >> x1 >> y1;
midpointLine(x0, y0, x1, y1);
break;
}
case 2: {
int x0, y0, r;
cout << "Enter center point (x0, y0) and radius: ";
cin >> x0 >> y0 >> r;
midpointCircle(x0, y0, r);
break;
}
case 3: {
int x, y, fillColor, borderColor;
cout << "Enter starting point (x, y), fill color and border color: ";
cin >> x >> y >> fillColor >> borderColor;
seedFill(x, y, fillColor, borderColor);
break;
}
case 4: {
int x0, y0, x1, y1;
cout << "Enter two points (x0, y0) and (x1, y1): ";
cin >> x0 >> y0 >> x1 >> y1;
cohenSutherlandLineClip(x0, y0, x1, y1);
break;
}
case 5:
break;
default:
cout << "Invalid choice!" << endl;
break;
}
} while (choice != 5);
closegraph();
return 0;
}
```
注意:此程序使用的是BGI图形库,在一些现代IDE环境中可能需要进行配置才能正常运行。
阅读全文
相关推荐
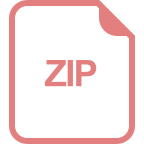
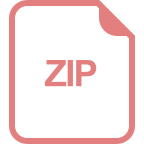

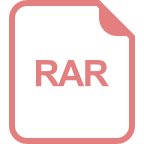
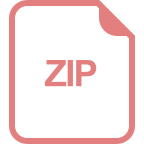
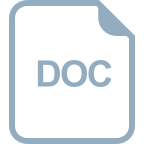
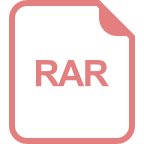
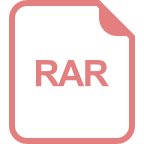
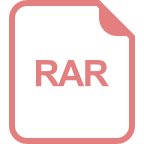
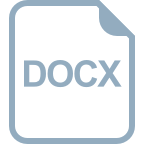
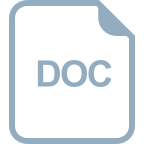
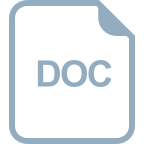
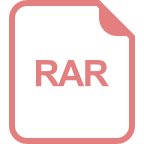
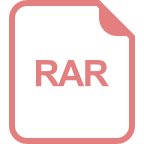
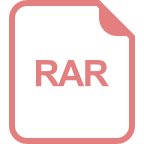
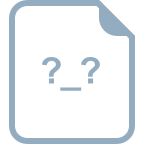