java 动态修改配置文件_Java 项目中一种简单的动态修改配置即时生效的方式 WatchService...
时间: 2024-03-16 20:43:26 浏览: 24
是的,Java项目中可以使用WatchService实现动态修改配置文件即时生效的功能。WatchService是Java 7引入的文件监控机制,可以监控文件或目录的变化,例如创建、修改、删除等操作,并在发生变化时通知应用程序。通过使用WatchService,我们可以实时监控配置文件的变化,并在发生修改时重新加载配置文件,从而实现配置即时生效的功能。具体实现方式可以参考以下代码:
```java
import java.io.File;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.WatchEvent;
import java.nio.file.WatchKey;
import java.nio.file.WatchService;
import java.util.HashMap;
import java.util.Map;
public class ConfigWatcher {
private WatchService watchService;
private Map<WatchKey, Path> keyPathMap;
public ConfigWatcher(String path) throws Exception {
watchService = FileSystems.getDefault().newWatchService();
keyPathMap = new HashMap<>();
Path dir = Paths.get(path);
registerAll(dir);
}
private void registerAll(final Path start) throws IOException {
Files.walkFileTree(start, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult preVisitDirectory(Path dir, BasicFileAttributes attrs)
throws IOException {
WatchKey key = dir.register(watchService, StandardWatchEventKinds.ENTRY_CREATE,
StandardWatchEventKinds.ENTRY_DELETE, StandardWatchEventKinds.ENTRY_MODIFY);
keyPathMap.put(key, dir);
return FileVisitResult.CONTINUE;
}
});
}
public void watch() {
while (true) {
WatchKey key;
try {
key = watchService.take();
} catch (InterruptedException e) {
return;
}
Path dir = keyPathMap.get(key);
if (dir == null) {
continue;
}
for (WatchEvent<?> event : key.pollEvents()) {
WatchEvent.Kind<?> kind = event.kind();
if (kind == StandardWatchEventKinds.OVERFLOW) {
continue;
}
WatchEvent<Path> ev = (WatchEvent<Path>) event;
Path filename = ev.context();
Path child = dir.resolve(filename);
if (kind == StandardWatchEventKinds.ENTRY_CREATE ||
kind == StandardWatchEventKinds.ENTRY_MODIFY) {
// 重新加载配置文件
} else if (kind == StandardWatchEventKinds.ENTRY_DELETE) {
// 删除配置文件
}
}
boolean valid = key.reset();
if (!valid) {
keyPathMap.remove(key);
if (keyPathMap.isEmpty()) {
break;
}
}
}
}
}
```
在上述代码中,我们通过watchService监控配置文件所在目录的变化,当有文件创建、修改、删除等操作时,会触发WatchEvent事件。我们可以在事件处理函数中重新加载配置文件,实现配置的即时生效。同时,我们也可以在事件处理函数中删除配置文件,防止无用的配置文件占用系统资源。
相关推荐
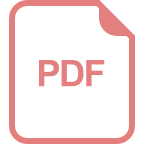
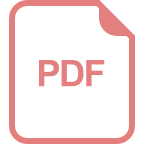
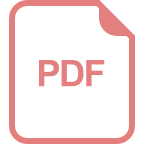
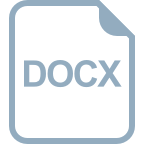
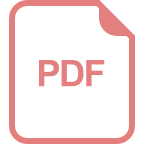
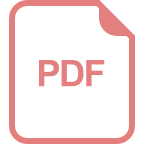
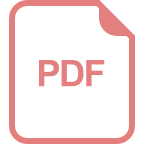
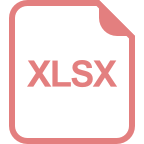
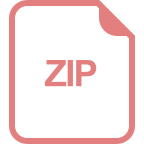
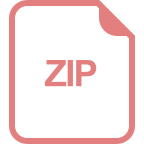
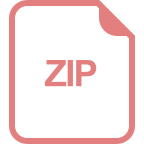
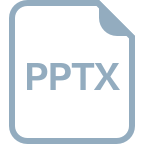