回归树的matlab实现
时间: 2023-07-14 16:14:18 浏览: 57
回归树是一种基于树结构的预测模型,它将数据集递归地分成多个小的区域,并在每个区域内拟合一个常数。回归树的实现可以通过以下步骤完成:
1. 数据准备:将数据集分成训练集和测试集,并进行必要的数据预处理。
2. 定义树的结构:定义树的节点结构,并初始化根节点。
3. 树的构建:通过递归的方式,对树进行分裂,直到满足停止条件。
4. 回归模型拟合:在每个叶节点,拟合一个回归模型。
5. 预测:通过遍历树,对新数据进行预测。
以下是一个简单的回归树的Matlab实现示例:
```matlab
% 生成样本数据
x = linspace(0, 2*pi, 100)';
y = sin(x) + randn(size(x))*0.1;
% 划分训练集和测试集
train_size = 80;
train_idx = randsample(length(x), train_size);
test_idx = setdiff(1:length(x), train_idx);
train_x = x(train_idx);
train_y = y(train_idx);
test_x = x(test_idx);
test_y = y(test_idx);
% 定义树的结构
tree = struct('left', {}, 'right', {}, 'feature', {}, 'threshold', {}, 'value', {});
% 初始化根节点
tree(1).left = 0;
tree(1).right = 0;
tree(1).feature = 0;
tree(1).threshold = 0;
tree(1).value = mean(train_y);
% 树的构建
max_depth = 5;
min_leaf_samples = 5;
tree = split_node(tree, train_x, train_y, max_depth, min_leaf_samples);
% 预测
y_pred = predict(tree, test_x);
% 绘制结果
figure;
plot(test_x, test_y, 'b.');
hold on;
plot(test_x, y_pred, 'r-');
legend('True', 'Predicted');
xlabel('x');
ylabel('y');
function tree = split_node(tree, x, y, max_depth, min_leaf_samples)
% 根据当前节点的深度和样本数量,决定是否停止分裂
if length(y) < min_leaf_samples || max_depth == 0
return;
end
% 在特征中选择最佳的分裂点
best_feature = 0;
best_threshold = 0;
best_loss = inf;
for i = 1:size(x, 2)
[sorted_x, sort_idx] = sort(x(:, i));
sorted_y = y(sort_idx);
for j = min_leaf_samples:length(y)-min_leaf_samples
left_y = sorted_y(1:j);
right_y = sorted_y(j+1:end);
left_loss = sum((left_y - mean(left_y)).^2);
right_loss = sum((right_y - mean(right_y)).^2);
loss = left_loss + right_loss;
if loss < best_loss
best_feature = i;
best_threshold = (sorted_x(j) + sorted_x(j+1)) / 2;
best_loss = loss;
end
end
end
% 分裂节点
left_idx = x(:, best_feature) <= best_threshold;
right_idx = ~left_idx;
if sum(left_idx) == 0 || sum(right_idx) == 0
return;
end
tree(end+1).left = length(tree)+1;
tree(end+1).right = length(tree)+2;
tree(end).feature = best_feature;
tree(end).threshold = best_threshold;
tree(end).value = mean(y(left_idx));
tree(end+1).value = mean(y(right_idx));
% 递归分裂左右子树
tree = split_node(tree, x(left_idx, :), y(left_idx), max_depth-1, min_leaf_samples);
tree = split_node(tree, x(right_idx, :), y(right_idx), max_depth-1, min_leaf_samples);
end
function y_pred = predict(tree, x)
y_pred = zeros(size(x, 1), 1);
for i = 1:size(x, 1)
node_idx = 1;
while tree(node_idx).left ~= 0
if x(i, tree(node_idx).feature) <= tree(node_idx).threshold
node_idx = tree(node_idx).left;
else
node_idx = tree(node_idx).right;
end
end
y_pred(i) = tree(node_idx).value;
end
end
```
在这个示例中,我们首先生成一个带有噪声的正弦曲线作为样本数据,然后将数据集分为训练集和测试集。接下来,我们定义了一个树结构,包括节点的左右子树、划分特征、划分阈值和叶节点的值。然后,我们初始化根节点,并开始递归地构建回归树。在构建树时,我们选择了最佳的特征和阈值来分裂当前节点,并在每个叶节点拟合一个常数。最后,我们使用预测函数对测试数据进行预测,并绘制了真实值和预测值之间的比较。
值得注意的是,这只是一个简单的回归树实现示例,实际上,回归树有许多变体和改进算法,例如CART和C4.5,可以在不同的应用场景中获得更好的性能。
相关推荐
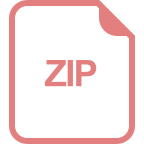
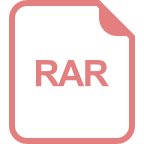
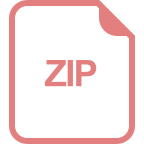














