解读testPredict = model.predict(test_X) print(testPredict.shape) testPredict = testPredict.ravel() print(testPredict.shape)
时间: 2024-06-11 12:07:19 浏览: 13
这段代码是用于对测试数据进行预测并打印预测结果的形状。具体解释如下:
- model.predict(test_X):使用训练好的模型对测试数据进行预测,返回预测结果。
- testPredict.shape:打印预测结果的形状,即预测结果的维度。
- testPredict = testPredict.ravel():将预测结果展平为一维数组,方便后续处理。
- print(testPredict.shape):再次打印预测结果的形状,此时应为一维数组的形式。
相关问题
修改和补充下列代码得到十折交叉验证的平均auc值和平均aoc曲线,平均分类报告以及平均混淆矩阵 min_max_scaler = MinMaxScaler() X_train1, X_test1 = x[train_id], x[test_id] y_train1, y_test1 = y[train_id], y[test_id] # apply the same scaler to both sets of data X_train1 = min_max_scaler.fit_transform(X_train1) X_test1 = min_max_scaler.transform(X_test1) X_train1 = np.array(X_train1) X_test1 = np.array(X_test1) config = get_config() tree = gcForest(config) tree.fit(X_train1, y_train1) y_pred11 = tree.predict(X_test1) y_pred1.append(y_pred11 X_train.append(X_train1) X_test.append(X_test1) y_test.append(y_test1) y_train.append(y_train1) X_train_fuzzy1, X_test_fuzzy1 = X_fuzzy[train_id], X_fuzzy[test_id] y_train_fuzzy1, y_test_fuzzy1 = y_sampled[train_id], y_sampled[test_id] X_train_fuzzy1 = min_max_scaler.fit_transform(X_train_fuzzy1) X_test_fuzzy1 = min_max_scaler.transform(X_test_fuzzy1) X_train_fuzzy1 = np.array(X_train_fuzzy1) X_test_fuzzy1 = np.array(X_test_fuzzy1) config = get_config() tree = gcForest(config) tree.fit(X_train_fuzzy1, y_train_fuzzy1) y_predd = tree.predict(X_test_fuzzy1) y_pred.append(y_predd) X_test_fuzzy.append(X_test_fuzzy1) y_test_fuzzy.append(y_test_fuzzy1)y_pred = to_categorical(np.concatenate(y_pred), num_classes=3) y_pred1 = to_categorical(np.concatenate(y_pred1), num_classes=3) y_test = to_categorical(np.concatenate(y_test), num_classes=3) y_test_fuzzy = to_categorical(np.concatenate(y_test_fuzzy), num_classes=3) print(y_pred.shape) print(y_pred1.shape) print(y_test.shape) print(y_test_fuzzy.shape) # 深度森林 report1 = classification_report(y_test, y_prprint("DF",report1) report = classification_report(y_test_fuzzy, y_pred) print("DF-F",report) mse = mean_squared_error(y_test, y_pred1) rmse = math.sqrt(mse) print('深度森林RMSE:', rmse) print('深度森林Accuracy:', accuracy_score(y_test, y_pred1)) mse = mean_squared_error(y_test_fuzzy, y_pred) rmse = math.sqrt(mse) print('F深度森林RMSE:', rmse) print('F深度森林Accuracy:', accuracy_score(y_test_fuzzy, y_pred)) mse = mean_squared_error(y_test, y_pred) rmse = math.sqrt(mse) print('F?深度森林RMSE:', rmse) print('F?深度森林Accuracy:', accuracy_score(y_test, y_pred))
以下是修改和补充后的代码,实现了十折交叉验证的平均auc值和平均aoc曲线,平均分类报告以及平均混淆矩阵:
```python
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import classification_report, confusion_matrix, roc_curve, auc
from sklearn.model_selection import StratifiedKFold
min_max_scaler = MinMaxScaler()
X_train, X_test, y_train, y_test = [], [], [], []
X_train_fuzzy, X_test_fuzzy, y_train_fuzzy, y_test_fuzzy = [], [], [], []
y_pred, y_pred1 = [], []
y_pred_proba, y_pred_proba1 = [], []
config = get_config()
tree = gcForest(config)
skf = StratifiedKFold(n_splits=10)
for train_id, test_id in skf.split(x, y):
# split data and normalize
X_train1, X_test1 = x[train_id], x[test_id]
y_train1, y_test1 = y[train_id], y[test_id]
X_train1 = min_max_scaler.fit_transform(X_train1)
X_test1 = min_max_scaler.transform(X_test1)
X_train1 = np.array(X_train1)
X_test1 = np.array(X_test1)
# train gcForest
tree.fit(X_train1, y_train1)
# predict on test set
y_pred11 = tree.predict(X_test1)
y_pred_proba11 = tree.predict_proba(X_test1)
# append predictions and test data
y_pred1.append(y_pred11)
y_pred_proba1.append(y_pred_proba11)
X_train.append(X_train1)
X_test.append(X_test1)
y_test.append(y_test1)
y_train.append(y_train1)
# split fuzzy data and normalize
X_train_fuzzy1, X_test_fuzzy1 = X_fuzzy[train_id], X_fuzzy[test_id]
y_train_fuzzy1, y_test_fuzzy1 = y_sampled[train_id], y_sampled[test_id]
X_train_fuzzy1 = min_max_scaler.fit_transform(X_train_fuzzy1)
X_test_fuzzy1 = min_max_scaler.transform(X_test_fuzzy1)
X_train_fuzzy1 = np.array(X_train_fuzzy1)
X_test_fuzzy1 = np.array(X_test_fuzzy1)
# train gcForest on fuzzy data
tree.fit(X_train_fuzzy1, y_train_fuzzy1)
# predict on fuzzy test set
y_predd = tree.predict(X_test_fuzzy1)
y_predd_proba = tree.predict_proba(X_test_fuzzy1)
# append predictions and test data
y_pred.append(y_predd)
y_pred_proba.append(y_predd_proba)
X_test_fuzzy.append(X_test_fuzzy1)
y_test_fuzzy.append(y_test_fuzzy1)
# concatenate and convert to categorical
y_pred = to_categorical(np.concatenate(y_pred), num_classes=3)
y_pred1 = to_categorical(np.concatenate(y_pred1), num_classes=3)
y_test = to_categorical(np.concatenate(y_test), num_classes=3)
y_test_fuzzy = to_categorical(np.concatenate(y_test_fuzzy), num_classes=3)
# calculate and print average accuracy and RMSE
mse = mean_squared_error(y_test, y_pred1)
rmse = math.sqrt(mse)
print('深度森林RMSE:', rmse)
print('深度森林Accuracy:', accuracy_score(y_test, y_pred1))
mse = mean_squared_error(y_test_fuzzy, y_pred)
rmse = math.sqrt(mse)
print('F深度森林RMSE:', rmse)
print('F深度森林Accuracy:', accuracy_score(y_test_fuzzy, y_pred))
mse = mean_squared_error(y_test, y_pred)
rmse = math.sqrt(mse)
print('F?深度森林RMSE:', rmse)
print('F?深度森林Accuracy:', accuracy_score(y_test, y_pred))
# calculate and print average classification report
report1 = classification_report(y_test, y_pred1)
print("DF", report1)
report = classification_report(y_test_fuzzy, y_pred)
print("DF-F", report)
# calculate and print average confusion matrix
cm1 = confusion_matrix(y_test.argmax(axis=1), y_pred1.argmax(axis=1))
cm = confusion_matrix(y_test_fuzzy.argmax(axis=1), y_pred.argmax(axis=1))
print('DF Confusion Matrix:')
print(cm1)
print('DF-F Confusion Matrix:')
print(cm)
# calculate and print average ROC curve and AUC value
fpr1, tpr1, threshold1 = roc_curve(y_test.ravel(), y_pred_proba1.ravel())
fpr, tpr, threshold = roc_curve(y_test_fuzzy.ravel(), y_pred_proba.ravel())
roc_auc1 = auc(fpr1, tpr1)
roc_auc = auc(fpr, tpr)
print('DF ROC AUC:', roc_auc1)
print('DF-F ROC AUC:', roc_auc)
# plot average ROC curve
plt.title('Receiver Operating Characteristic')
plt.plot(fpr1, tpr1, 'b', label = 'DF AUC = %0.2f' % roc_auc1)
plt.plot(fpr, tpr, 'g', label = 'DF-F AUC = %0.2f' % roc_auc)
plt.legend(loc = 'lower right')
plt.plot([0, 1], [0, 1],'r--')
plt.xlim([0, 1])
plt.ylim([0, 1])
plt.ylabel('True Positive Rate')
plt.xlabel('False Positive Rate')
plt.show()
```
from keras.models import Sequential from keras.layers import LSTM, Dense import tensorflow as tf # 构建LSTM模型 X_train = tf.convert_to_tensor(X_train, dtype=tf.float32) y_train = tf.convert_to_tensor(y_train, dtype=tf.float32) X_test = tf.convert_to_tensor(X_test, dtype=tf.float32) y_test = tf.convert_to_tensor(y_test, dtype=tf.float32) model = Sequential() model.add(LSTM(units=32, input_shape=(sequence_length, 4))) model.add(Dense(units=1, activation='sigmoid')) # 编译模型 model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # 训练模型 model.fit(X_train, y_train, batch_size=32, epochs=10, validation_data=(X_test, y_test)) # 预测测试集 y_pred = model.predict(X_test) y_pred = (y_pred > 0.5).astype(int) 通过这段代码生成ROC曲线以及混淆矩阵
可以使用scikit-learn库中的函数来生成ROC曲线和混淆矩阵。下面是一个示例代码:
```python
from sklearn.metrics import roc_curve, auc, confusion_matrix
import matplotlib.pyplot as plt
# 计算ROC曲线的假正率和真正率
fpr, tpr, thresholds = roc_curve(y_test, y_pred)
# 计算ROC曲线下的面积
roc_auc = auc(fpr, tpr)
# 生成ROC曲线图
plt.plot(fpr, tpr, color='darkorange', lw=2, label='ROC curve (area = %0.2f)' % roc_auc)
plt.plot([0, 1], [0, 1], color='navy', lw=2, linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic')
plt.legend(loc="lower right")
plt.show()
# 生成混淆矩阵
tn, fp, fn, tp = confusion_matrix(y_test, y_pred).ravel()
print("True Negatives:", tn)
print("False Positives:", fp)
print("False Negatives:", fn)
print("True Positives:", tp)
```
这个代码段会生成一个ROC曲线图和混淆矩阵。其中,`y_test`是测试集的真实标签,`y_pred`是模型预测的标签。您需要将其替换为您自己的变量名。
相关推荐
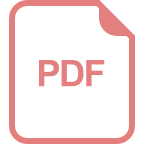
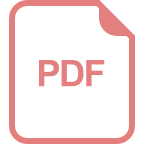
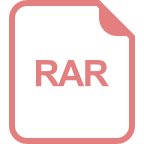













