用一个例子说明ioctl
时间: 2024-04-29 13:25:27 浏览: 7
假设我们有一个串口设备,我们想要设置其波特率和停止位,使用ioctl函数可以实现。
首先,我们需要打开串口设备,使用open函数:
```
int fd = open("/dev/ttyS0", O_RDWR);
```
然后,我们可以定义一个结构体来保存串口参数:
```
struct termios tty;
```
接着,我们需要获取当前的串口参数,使用tcgetattr函数:
```
tcgetattr(fd, &tty);
```
然后,我们可以修改串口参数,例如设置波特率为9600:
```
cfsetospeed(&tty, B9600);
```
再次调用tcsetattr函数将修改后的参数应用到串口设备:
```
tcsetattr(fd, TCSANOW, &tty);
```
最后,我们可以使用ioctl函数设置停止位:
```
int stop_bits = 1;
ioctl(fd, TIOCMGET, &stop_bits);
stop_bits &= ~CSTOPB;
ioctl(fd, TIOCMSET, &stop_bits);
```
其中,TIOCMGET用于获取串口控制信息,TIOCMSET用于设置串口控制信息,CSTOPB表示使用两个停止位,我们可以将其取反表示使用一个停止位。
完整的代码如下:
```
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <sys/ioctl.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR);
if (fd < 0) {
perror("open");
return -1;
}
struct termios tty;
tcgetattr(fd, &tty);
cfsetospeed(&tty, B9600);
tcsetattr(fd, TCSANOW, &tty);
int stop_bits = 1;
ioctl(fd, TIOCMGET, &stop_bits);
stop_bits &= ~CSTOPB;
ioctl(fd, TIOCMSET, &stop_bits);
close(fd);
return 0;
}
```
相关推荐
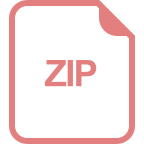
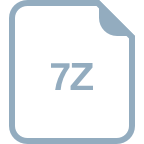
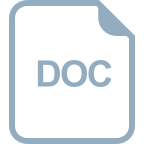
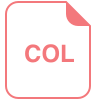
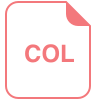












