用c/c++源码举例说明应用软件调用驱动软件实例
时间: 2023-07-16 12:16:39 浏览: 59
假设我们有一个驱动程序,它可以控制一个LED灯的开关。现在我们要编写一个应用程序来控制这个LED灯,我们可以使用以下步骤来调用驱动程序:
1. 打开设备驱动程序
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main()
{
int fd;
fd = open("/dev/led", O_RDWR); // 打开设备文件
if(fd < 0)
{
printf("Can't open device file.\n");
return -1;
}
return 0;
}
```
2. 控制LED灯
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#define LED_ON _IO('L', 0)
#define LED_OFF _IO('L', 1)
int main()
{
int fd;
fd = open("/dev/led", O_RDWR); // 打开设备文件
if(fd < 0)
{
printf("Can't open device file.\n");
return -1;
}
ioctl(fd, LED_ON); // 控制LED灯亮
sleep(5); // 程序暂停5秒
ioctl(fd, LED_OFF); // 控制LED灯灭
close(fd); // 关闭设备文件
return 0;
}
```
在上面的例子中,我们使用了 `open` 函数来打开设备文件,然后使用 `ioctl` 函数来控制LED灯的开关,最后使用 `close` 函数来关闭设备文件。这些函数都是操作系统提供的系统调用,我们可以使用C/C++语言来调用它们。通过这些函数的调用,应用程序就可以和驱动程序进行交互,从而实现控制硬件设备的功能。
相关推荐
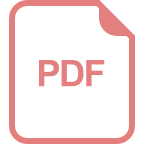
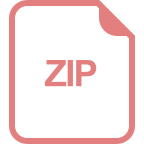
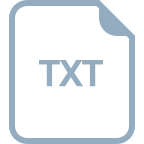
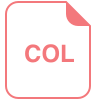

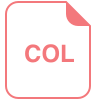
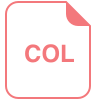
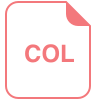









