python bp神经网络 异或_BP神经网络求解异或问题(Python实现)
时间: 2023-10-28 08:01:37 浏览: 76
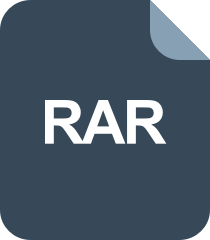
BP神经网络解决异或问题_BP算法_
好的,下面是 Python 实现的 BP 神经网络解决异或问题的代码:
```python
import numpy as np
# 定义 sigmoid 函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义 sigmoid 函数的导数
def sigmoid_derivative(x):
return x * (1 - x)
# 训练 BP 神经网络
def train(X, y, hidden_neurons=3, epochs=10000, alpha=0.1):
# 初始化输入层权重和偏置
input_neurons = X.shape[1]
w1 = np.random.uniform(size=(input_neurons, hidden_neurons))
b1 = np.random.uniform(size=(1, hidden_neurons))
# 初始化输出层权重和偏置
output_neurons = y.shape[1]
w2 = np.random.uniform(size=(hidden_neurons, output_neurons))
b2 = np.random.uniform(size=(1, output_neurons))
# 开始训练
for i in range(epochs):
# 前向传播
hidden_layer_input = np.dot(X, w1) + b1
hidden_layer_output = sigmoid(hidden_layer_input)
output_layer_input = np.dot(hidden_layer_output, w2) + b2
output_layer_output = sigmoid(output_layer_input)
# 反向传播
error = y - output_layer_output
d_output = error * sigmoid_derivative(output_layer_output)
error_hidden_layer = d_output.dot(w2.T)
d_hidden_layer = error_hidden_layer * sigmoid_derivative(hidden_layer_output)
# 更新权重和偏置
w2 += hidden_layer_output.T.dot(d_output) * alpha
b2 += np.sum(d_output, axis=0, keepdims=True) * alpha
w1 += X.T.dot(d_hidden_layer) * alpha
b1 += np.sum(d_hidden_layer, axis=0, keepdims=True) * alpha
return w1, b1, w2, b2
# 测试 BP 神经网络
def predict(X, w1, b1, w2, b2):
# 前向传播
hidden_layer_input = np.dot(X, w1) + b1
hidden_layer_output = sigmoid(hidden_layer_input)
output_layer_input = np.dot(hidden_layer_output, w2) + b2
output_layer_output = sigmoid(output_layer_input)
return output_layer_output
# 定义输入和输出数据
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]])
# 训练 BP 神经网络
w1, b1, w2, b2 = train(X, y, hidden_neurons=3, epochs=10000, alpha=0.1)
# 测试 BP 神经网络
predictions = predict(X, w1, b1, w2, b2)
print(predictions)
```
代码中使用了 sigmoid 函数作为激活函数,并且使用了 sigmoid 函数的导数来计算反向传播的梯度。在训练时,通过随机初始化权重和偏置,使用前向传播和反向传播来更新权重和偏置,最终得到训练好的 BP 神经网络。在测试时,使用训练好的 BP 神经网络来预测输出结果。
阅读全文
相关推荐
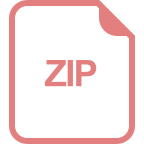
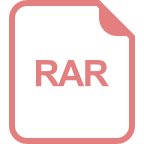
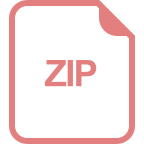
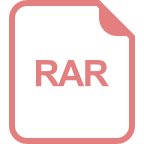
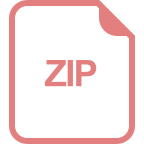
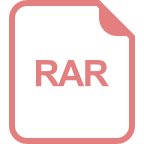
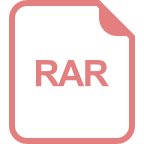
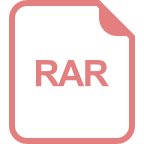
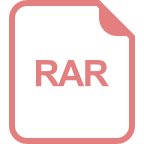
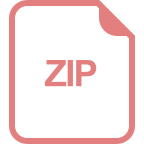
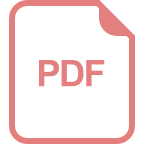