int Fun_Any_10(int cet) { Stack S = (Stack)malloc(sizeof(struct StackNode));; InitStack(S); char c; int num, sum = 0; printf("请输入要转化的数:\n"); c = getchar(); while (c != '\n') { if (isalnum(c)) Push(S, c - '0'); else Push(S, tolower(c) - 'a' + 10); c = getchar(); } int i = 0; while (!IsEmpty(S)) { num = Top(S); Pop(S); sum += (num * (int)pow(cet, i)); ++i; } free(S); return sum; }
时间: 2023-07-15 09:11:17 浏览: 83
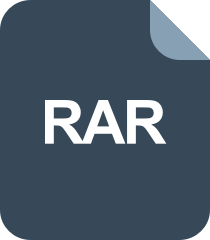
STACK1_SEGMENT_STACK.rar_STACK1 SEGMENT_stack segment stack

这是一个将任意进制的数转成十进制的函数。输入参数是一个整数cet,表示需要转化的数的进制。函数内部首先创建了一个栈S,并且通过InitStack函数初始化。接着,程序通过getchar()函数逐个读取输入的字符,如果是数字或字母,则将其转化成数值并压入栈S中;如果不是,则直接忽略。最后,程序通过弹出栈顶元素并乘以对应进制的幂次方,计算出十进制数值。最后释放栈内存,并返回所得到的十进制数值。
阅读全文
相关推荐
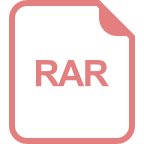
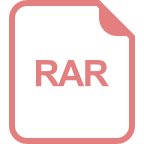

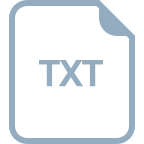





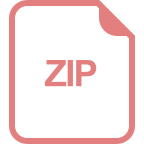
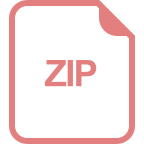
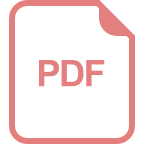