用c语言编写链式栈s,分别编写算法SelectItem,要求在堆栈中查找元素n在栈中第一次出现的位置,并将该位置元素移至栈顶,同时其他元素次序不变。
时间: 2024-09-24 13:30:14 浏览: 8
在C语言中,我们可以使用链式结构(如单向链表)来实现一个简单的链式栈S。首先,我们需要定义一个节点结构体,包含数据域和指向下一个节点的指针。然后,可以创建一个函数`push()`用于入栈,`pop()`用于出栈,以及一个辅助函数`selectItem()`来查找元素n并将其移到栈顶。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链式栈节点结构体
typedef struct Node {
int data;
struct Node* next;
} StackNode;
// 初始化链式栈
StackNode* initStack() {
return NULL;
}
// 入栈操作
void push(StackNode** top, int value) {
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode));
if (newNode != NULL) {
newNode->data = value;
newNode->next = *top;
*top = newNode;
}
}
// 出栈操作
int pop(StackNode** top) {
if (*top == NULL) {
return -1; // 栈空
}
int value = (*top)->data;
StackNode* temp = *top;
*top = (*top)->next;
free(temp);
return value;
}
// 查找并移动元素n到栈顶
int selectItem(StackNode** top, int n) {
StackNode* current = *top;
while (current != NULL && current->data != n) {
current = current->next;
}
if (current == NULL) { // 如果没找到n,则返回-1
return -1;
}
// 移动n到栈顶,需要保存当前栈顶元素
StackNode* temp = *top;
*top = current;
// 将原栈顶元素插入n之后
current->next = temp->next;
return current->data; // 返回n在栈中的位置
}
int main() {
StackNode* stackTop = initStack();
// 插入元素
push(&stackTop, 5);
push(&stackTop, 3);
push(&stackTop, n); // 你要查找的元素
int itemLocation = selectItem(&stackTop, n);
if (itemLocation != -1) {
printf("元素 %d 的位置移到了栈顶,新栈顶元素为 %d\n", n, itemLocation);
} else {
printf("元素 %d 没有在栈中找到。\n", n);
}
// 清理内存
StackNode* temp = stackTop;
while (temp != NULL) {
StackNode* nextTemp = temp->next;
free(temp);
temp = nextTemp;
}
return 0;
}
```
相关推荐
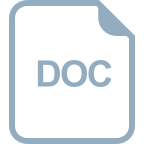
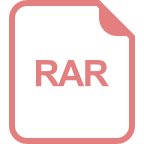














